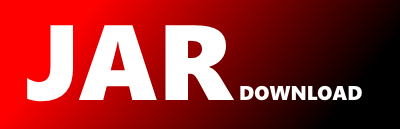
org.beigesoft.ajetty.IMngDb Maven / Gradle / Ivy
/*
BSD 2-Clause License
Copyright (c) 2019, Beigesoft™
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.beigesoft.ajetty;
import java.util.List;
import java.util.Map;
/**
* Database manager for file-based RDBMS (SQLite/H2).
* It can create and change current database (file),
* backup DB to global storage and restore from it,
* also can delete database. Additional it encrypt/decrypt all log files.
*
* @author Yury Demidenko
*/
public interface IMngDb {
/**
* Lists databases.
* @param pRvs request scoped vars
* @return List list of database files.
* @throws Exception - an exception
**/
List retLst(Map pRvs) throws Exception;
/**
* Retrieves current DB name.
* @param pRvs request scoped vars
* @return String DB name.
* @throws Exception - an exception
**/
String retCurDbNm(Map pRvs) throws Exception;
/**
* Creates new database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @param pDbId database ID
* @throws Exception - an exception
**/
void createDb(Map pRvs, String pDbNm,
int pDbId) throws Exception;
/**
* Changes database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
void changeDb(Map pRvs, String pDbNm) throws Exception;
/**
* Lists backup databases.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
List retBckLst(Map pRvs) throws Exception;
/**
* Lists logs in backup folder.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
List retLogLst(Map pRvs) throws Exception;
/**
* Lists encrypted logs in backup folder.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
List retEnLogLst(Map pRvs) throws Exception;
/**
* Deletes database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
void deleteDb(Map pRvs, String pDbNm) throws Exception;
/**
* Delete all encrypted database files.
* @param pRvs request scoped vars
* @param pNm database name without extension
* @throws Exception - an exception
**/
void deleteEnDb(Map pRvs, String pNm) throws Exception;
/**
* Delete all encrypted log files.
* @param pRvs request scoped vars
* @param pNm log name without extension
* @throws Exception - an exception
**/
void deleteEnLog(Map pRvs, String pNm) throws Exception;
/**
* Delete log file.
* @param pRvs request scoped vars
* @param pNm log name without extension
* @throws Exception - an exception
**/
void deleteLog(Map pRvs, String pNm) throws Exception;
/**
* Backups database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
void backupDb(Map pRvs, String pDbNm) throws Exception;
/**
* Restores database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
void restoreDb(Map pRvs, String pDbNm) throws Exception;
/**
* Encrypts log files into backup directory.
* @param pRvs request scoped vars
* @throws Exception - an exception
**/
void encryptLogs(Map pRvs) throws Exception;
/**
* Decrypts log files in backup directory.
* @param pRvs request scoped vars
* @throws Exception - an exception
**/
void decryptLogs(Map pRvs) throws Exception;
/**
* Getter for backupDir.
* @return String
**/
String getBackupDir();
/**
* Setter for backupDir.
* @param pBackupDir reference
**/
void setBackupDir(String pBackupDir);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy