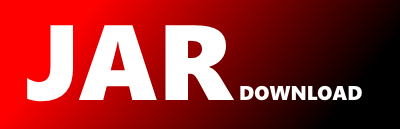
org.beigesoft.ajetty.MngDb Maven / Gradle / Ivy
/*
BSD 2-Clause License
Copyright (c) 2019, Beigesoft™
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.beigesoft.ajetty;
import java.io.File;
import java.util.Date;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import org.beigesoft.exc.ExcCode;
import org.beigesoft.mdl.IReqDt;
import org.beigesoft.mdl.RqCtxAttr;
import org.beigesoft.fct.IFctAsm;
/**
* Database manager with encryption.
* Additional it encrypt/decrypt all log files.
*
* @param platform dependent RDBMS recordset
* @author Yury Demidenko
*/
public class MngDb implements IMngDb {
/**
* Log files folder (inner).
**/
private File logDir;
/**
* Databases folder (inner).
**/
private String dbDir;
/**
* Encrypted backup databases folder (public).
**/
private String backupDir;
/**
* App beans factory.
**/
private IFctAsm fctApp;
/**
* Crypto service.
**/
private IHpCrypt hpCrypt;
/**
* Database prefix - for JDBC is "jdbc:sqlite:[db-dir]/".
**/
private String dbPref = "";
/**
* Lists databases.
* @param pRvs request scoped vars
* @return List list of database files.
* @throws Exception - an exception
**/
@Override
public final List retLst(
final Map pRvs) throws Exception {
List result = new ArrayList();
File dbDr = new File(this.dbDir);
if (dbDr.exists() && dbDr.isDirectory()) {
String[] files = dbDr.list();
if (files != null) {
for (String flNm : files) {
if (flNm.endsWith(".sqlite")) {
result.add(flNm.replace(".sqlite", ""));
}
}
}
} else {
throw new ExcCode(ExcCode.WRCN,
"DB directory not found: " + this.dbDir);
}
return result;
}
/**
* Lists backup databases.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
@Override
public final List retBckLst(
final Map pRvs) throws Exception {
List result = new ArrayList();
File dbDr = new File(this.backupDir);
if (dbDr.exists() && dbDr.isDirectory()) {
String[] files = dbDr.list();
if (files != null) {
for (String flNm : files) {
if (flNm.endsWith(".sqlten")) {
result.add(flNm.replace(".sqlten", ""));
}
}
}
} else {
throw new ExcCode(ExcCode.WRCN,
"Backup directory not found: " + this.backupDir);
}
return result;
}
/**
* Lists logs in backup folder.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
@Override
public final List retLogLst(
final Map pRvs) throws Exception {
List result = new ArrayList();
File dbDr = new File(this.backupDir);
if (dbDr.exists() && dbDr.isDirectory()) {
String[] files = dbDr.list();
if (files != null) {
for (String flNm : files) {
if (flNm.endsWith(".log")) {
result.add(flNm.replace(".log", ""));
}
}
}
} else {
throw new ExcCode(ExcCode.WRCN,
"Backup directory not found: " + this.backupDir);
}
return result;
}
/**
* Lists encrypted logs in backup folder.
* @param pRvs request scoped vars
* @return List list of backup database files.
* @throws Exception - an exception
**/
@Override
public final List retEnLogLst(
final Map pRvs) throws Exception {
List result = new ArrayList();
File dbDr = new File(this.backupDir);
if (dbDr.exists() && dbDr.isDirectory()) {
String[] files = dbDr.list();
if (files != null) {
for (String flNm : files) {
if (flNm.endsWith(".logen")) {
result.add(flNm.replace(".logen", ""));
}
}
}
} else {
throw new ExcCode(ExcCode.WRCN,
"Backup directory not found: " + this.backupDir);
}
return result;
}
/**
* Retrieves current DB name.
* @param pRvs request scoped vars
* @return String DB name.
* @throws Exception - an exception
**/
@Override
public final String retCurDbNm(
final Map pRvs) throws Exception {
return this.fctApp.getFctBlc().getFctDt().getDbUrl();
}
/**
* Creates new database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @param pDbId database ID
* @throws Exception - an exception
**/
@Override
public final void createDb(final Map pRvs,
final String pDbNm, final int pDbId) throws Exception {
String dbNm = this.dbPref + pDbNm + ".sqlite";
synchronized (this.fctApp) {
if (!this.fctApp.getFctBlc().getFctDt().getDbUrl().equals(dbNm)) {
this.fctApp.getFctBlc().getFctDt().setDbUrl(dbNm);
this.fctApp.getFctBlc().getFctDt().setNewDbId(pDbId);
this.fctApp.getFctBlc().release(pRvs);
IReqDt rqDt = (IReqDt) pRvs.get("rqDt");
this.fctApp.init(pRvs, new RqCtxAttr(rqDt));
}
}
}
/**
* Changes database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
@Override
public final void changeDb(final Map pRvs,
final String pDbNm) throws Exception {
String dbNm = this.dbPref + pDbNm + ".sqlite";
synchronized (this.fctApp) {
if (!this.fctApp.getFctBlc().getFctDt().getDbUrl().equals(dbNm)) {
this.fctApp.getFctBlc().getFctDt().setDbUrl(dbNm);
this.fctApp.getFctBlc().release(pRvs);
IReqDt rqDt = (IReqDt) pRvs.get("rqDt");
this.fctApp.init(pRvs, new RqCtxAttr(rqDt));
}
}
}
/**
* Deletes database in inner DB folder, NOT current DB!
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
@Override
public final void deleteDb(final Map pRvs,
final String pDbNm) throws Exception {
String dbNm = this.dbPref + pDbNm + ".sqlite";
if (!dbNm.equals(this.fctApp.getFctBlc().getFctDt().getDbUrl())) {
File dbFile = new File(this.dbDir + File.separator + pDbNm + ".sqlite");
if (dbFile.exists() && !dbFile.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + dbFile);
}
}
}
/**
* Delete all encrypted database files.
* @param pRvs request scoped vars
* @param pNm database name without extension
* @throws Exception - an exception
**/
@Override
public final void deleteEnDb(final Map pRvs,
final String pNm) throws Exception {
File fl = new File(this.backupDir + File.separator + pNm + ".sqlten");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".sqlten.sig");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".sqlten.sken");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".sqlten.sken.sig");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
}
/**
* Delete all encrypted log files.
* @param pRvs request scoped vars
* @param pNm log name without extension
* @throws Exception - an exception
**/
@Override
public final void deleteEnLog(final Map pRvs,
final String pNm) throws Exception {
File fl = new File(this.backupDir + File.separator + pNm + ".logen");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".logen.sig");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".logen.sken");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
fl = new File(this.backupDir + File.separator + pNm + ".logen.sken.sig");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
}
/**
* Delete encrypted log file.
* @param pRvs request scoped vars
* @param pNm log name without extension
* @throws Exception - an exception
**/
@Override
public final void deleteLog(final Map pRvs,
final String pNm) throws Exception {
File fl = new File(this.backupDir + File.separator + pNm + ".log");
if (fl.exists() && !fl.delete()) {
throw new ExcCode(ExcCode.WR, "Can't delete file: " + fl);
}
}
/**
* Backups database.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
@Override
public final void backupDb(final Map pRvs,
final String pDbNm) throws Exception {
String dbPath = this.dbDir + File.separator + pDbNm + ".sqlite";
File dbFile = new File(dbPath);
if (dbFile.exists()) {
String encPath = this.backupDir + File.separator + pDbNm + ".sqlten";
File dbBkFile = new File(encPath);
if (dbBkFile.exists()) {
Long time = new Date().getTime();
encPath = this.backupDir + File.separator + pDbNm + time + ".sqlten";
}
this.hpCrypt.encryptFile(dbPath, encPath);
}
}
/**
* Restores database from backup.
* @param pRvs request scoped vars
* @param pDbNm database name without extension
* @throws Exception - an exception
**/
@Override
public final void restoreDb(final Map pRvs,
final String pDbNm) throws Exception {
String encPath = this.backupDir + File.separator + pDbNm + ".sqlten";
File dbBkFile = new File(encPath);
if (dbBkFile.exists()) {
this.hpCrypt.decryptFile(encPath,
this.dbDir + File.separator + pDbNm + ".sqlite");
}
}
/**
* Encrypts log files into backup directory.
* @param pRvs request scoped vars
* @throws Exception - an exception
**/
@Override
public final void encryptLogs(
final Map pRvs) throws Exception {
if (this.logDir != null && this.logDir.exists() && !this.logDir.isFile()) {
File[] files = this.logDir.listFiles();
if (files != null) {
for (File fl : files) {
if (fl.getName().endsWith(".log")) {
this.hpCrypt.encryptFile(fl.getPath(),
this.backupDir + File.separator + fl.getName() + "en");
}
}
}
}
}
/**
* Decrypts log files in backup directory.
* @param pRvs request scoped vars
* @throws Exception - an exception
**/
@Override
public final void decryptLogs(
final Map pRvs) throws Exception {
File bkDir = new File(this.backupDir);
if (bkDir.exists() && !bkDir.isFile()) {
File[] files = bkDir.listFiles();
if (files != null) {
for (File fl : files) {
if (fl.getName().endsWith(".logen")) {
this.hpCrypt.decryptFile(fl.getPath(), this.backupDir
+ File.separator + fl.getName().replace(".logen", ".log"));
}
}
}
}
}
/**
* Getter for backupDir.
* @return String
**/
@Override
public final String getBackupDir() {
return this.backupDir;
}
/**
* Setter for backupDir.
* @param pBackupDir reference
**/
@Override
public final void setBackupDir(final String pBackupDir) {
this.backupDir = pBackupDir;
}
//Simple getters and setters:
/**
* Getter for logDir.
* @return File
**/
public final File getLogDir() {
return this.logDir;
}
/**
* Setter for logDir.
* @param pLogDir reference
**/
public final void setLogDir(final File pLogDir) {
this.logDir = pLogDir;
}
/**
* Getter for dbDir.
* @return String
**/
public final String getDbDir() {
return this.dbDir;
}
/**
* Setter for dbDir.
* @param pDbDir reference
**/
public final void setDbDir(final String pDbDir) {
this.dbDir = pDbDir;
}
/**
* Getter for fctApp.
* @return IFctAsm
**/
public final IFctAsm getFctApp() {
return this.fctApp;
}
/**
* Setter for fctApp.
* @param pFctApp reference
**/
public final void setFctApp(final IFctAsm pFctApp) {
this.fctApp = pFctApp;
}
/**
* Getter for hpCrypt.
* @return IHpCrypt
**/
public final IHpCrypt getHpCrypt() {
return this.hpCrypt;
}
/**
* Setter for hpCrypt.
* @param pHpCrypt reference
**/
public final void setHpCrypt(final IHpCrypt pHpCrypt) {
this.hpCrypt = pHpCrypt;
}
/**
* Getter for dbPref.
* @return String
**/
public final String getDbPref() {
return this.dbPref;
}
/**
* Setter for dbPref.
* @param pDbPref reference
**/
public final void setDbPref(final String pDbPref) {
this.dbPref = pDbPref;
}
}