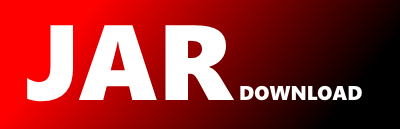
org.beigesoft.settings.IMngSettings Maven / Gradle / Ivy
package org.beigesoft.settings;
/*
* Copyright (c) 2016 Beigesoft ™
*
* Licensed under the GNU General Public License (GPL), Version 2.0
* (the "License");
* you may not use this file except in compliance with the License.
*
* You may obtain a copy of the License at
*
* http://www.gnu.org/licenses/old-licenses/gpl-2.0.en.html
*/
import java.util.Map;
import java.util.Set;
import java.util.LinkedHashSet;
import java.util.List;
/**
* Make settings for application, classes and its fields
* according properties XML.
* According Beige-Settings specification #2.
*
*
* @author Yury Demidenko
*/
public interface IMngSettings {
/**
* Key name for settings subfolder.
**/
String KEY_USE_SUB_FOLDER = "useSubFolder";
/**
* Key name for classes.
**/
String KEY_CLASSES = "classes";
/**
* Key name for fields settings names.
**/
String KEY_CLASS_SETTINGS_NAMES = "settingsKeysForClasses";
/**
* Key name for fields settings names.
**/
String KEY_FIELD_SETTINGS_NAMES = "settingsKeysForFields";
/**
* Key name for exclude fields.
**/
String KEY_EXCLUDED_FIELDS = "excludeFields";
/**
* App setting file name.
**/
String APP_SETTINGS_FILE_NAME = "app-settings.xml";
/**
* Folder class type to classes setting.
**/
String FOLDER_CLASS_TYPE_TO_CS = "classTypeToCs";
/**
* Folder class name to classes setting.
**/
String FOLDER_CLASS_TO_CS = "forClassToCs";
/**
* Folder field type to field setting.
**/
String FOLDER_FIELD_TYPE_TO_FS = "fieldTypeToFs";
/**
* Folder field name field type to field setting.
**/
String DIR_FIELD_NAME_FIELD_TYPE_TO_FS = "fieldNameFieldTypeToFs";
/**
* Folder field name class type to field setting.
**/
String DIR_FIELD_NAME_CLASS_TYPE_TO_FS = "fieldNameClassTypeToFs";
/**
* Folder field name to field setting.
**/
String FOLDER_FIELD_NAME_TO_FS = "fieldNameToFs";
/**
* Folder class name to fields setting.
**/
String FOLDER_CLASS_TO_FS = "forClassToFs";
/**
* Load configuration(settings) from given folder and file.
* @param pDirName base properties directory name
* @param pFileName base properties file name
* @throws Exception - an exception
*/
void loadConfiguration(String pDirName, String pFileName) throws Exception;
/**
* Sorted entries and also removed those with negative value.
* @param pSetEntries Set>> source
* @param pOrderKey key of order property
* @return List>> list ordered according
* entry.getValue().get(pOrderKey) that is Integer.toString()
* @throws Exception - an exception
*/
List>> sortRemoveIntVal(
Set>> pSetEntries, String pOrderKey) throws Exception;
/**
* Make for given class sorted entries of fields settings
* and also removed those with negative value of sodted property.
* @param pClass entity Class
* @param pOrderKey key of order property
* @return List>> list ordered according
* entry.getValue().get(pOrderKey) that is Integer.toString()
* @throws Exception - an exception
*/
List>> makeFldPropLst(
Class pClass, String pOrderKey) throws Exception;
//GS:
/**
* Get all involved classes.
* @return LinkedHashSet all involved clase
*/
LinkedHashSet> getClasses();
/**
* Set all involved classes.
* @param pClasses LinkedHashSet all involved clase
*/
void setClasses(LinkedHashSet> pClasses);
/**
* Get application settings.
* @return Map application settings
*/
Map getAppSettings();
/**
* Set application settings.
* @param pAppSettings Map application settings
*/
void setAppSettings(Map pAppSettings);
/**
* Get class settings.
* This is Map "Class" -
* "Map>"
* @return Map> class settings
*/
Map, Map> getClassesSettings();
/**
* Set class settings.
* This is Map "Class" -
* "Map>"
* @param pClassSettings Map, Map> class settings
*/
void setClassesSettings(Map, Map> pClassSettings);
/**
* Get fields settings.
* This is Map "Class" -
* "Map "fieldName" - "Map "settingName"-"settingValue"""
* @return Map, Map>> fields settings
*/
Map, Map>> getFieldsSettings();
/**
* Set fields settings.
* This is Map "Class" -
* "Map "fieldName" - "Map "settingName"-"settingValue"""
* @param pFieldsSettings Map, Map>>
* fields settings
*/
void setFieldsSettings(
Map, Map>> pFieldsSettings);
}