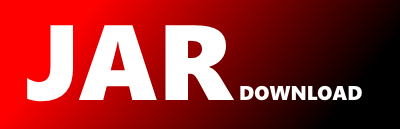
com.embeddedunveiled.serial.usb.SerialComUSB Maven / Gradle / Ivy
Show all versions of scm Show documentation
/*
* Author : Rishi Gupta
*
* This file is part of 'serial communication manager' library.
* Copyright (C) <2014-2016>
*
* This 'serial communication manager' is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later version.
*
* The 'serial communication manager' is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with 'serial communication manager'. If not, see .
*/
package com.embeddedunveiled.serial.usb;
import com.embeddedunveiled.serial.SerialComException;
import com.embeddedunveiled.serial.internal.SerialComPortJNIBridge;
/**
* Encapsulates USB related operations and values.
*
* An end product may be based on dedicated USB-UART bridge IC for providing serial over USB or
* may use general purpose microcontroller like PIC18F4550 from Microchip technology Inc. and
* program appropriate firmware (USB CDC) into it to provide UART communication over USB port.
*
* [1] If your USB-UART converter based design is not working, consider not connecting USB connector
* shield directly to ground. Further, double check if termination resistors in D+/D- lines are
* really required or not.
*
* @author Rishi Gupta
*/
public final class SerialComUSB {
/** Value indicating all vendors (vendor neutral operation).
*/
public static final int V_ALL = 0x0000;
/** Value indicating vendor - Future technology devices international, Ltd. It manufactures FT232
* USB-UART bridge IC.
*/
public static final int V_FTDI = 0x0403;
/** Value indicating vendor - Silicon Laboratories. It manufactures CP2102 USB-UART bridge IC.
*/
public static final int V_SLABS = 0x10C4;
/** Value indicating vendor - Microchip technology Inc. It manufactures MCP2200 USB-UART bridge IC.
*/
public static final int V_MCHIP = 0x04D8;
/** Value indicating vendor - Prolific technology Inc. It manufactures PL2303 USB-UART bridge IC.
*/
public static final int V_PL = 0x067B;
/** Value indicating vendor - Exar corporation. It manufactures XR21V1410 USB-UART bridge IC.
*/
public static final int V_EXAR = 0x04E2;
/** Value indicating vendor - Atmel corporation. It manufactures AT90USxxx and other processors which
* can be used as USB-UART bridge.
*/
public static final int V_ATML = 0x03EB;
/** Value indicating vendor - MosChip semiconductor. It manufactures MCS7810 USB-UART bridge IC.
*/
public static final int V_MOSCHP = 0x9710;
/** Value indicating vendor - Cypress semiconductor corporation. It manufactures CY7C65213 USB-UART
* bridge IC.
*/
public static final int V_CYPRS = 0x04B4;
/** Value indicating vendor - Texas instruments, Inc. It manufactures TUSB3410 USB-UART bridge IC.
*/
public static final int V_TI = 0x0451;
/** Value indicating vendor - WinChipHead. It manufactures CH340 USB-UART bridge IC.
*/
public static final int V_WCH = 0x4348;
/** Value indicating vendor - QinHeng electronics. It manufactures HL-340 converter product.
*/
public static final int V_QHE = 0x1A86;
/** Value indicating vendor - NXP semiconductors. It manufactures LPC134x series of microcontrollers.
*/
public static final int V_NXP = 0x1FC9;
/** Value indicating vendor - Renesas electronics (NEC electronics). It manufactures μPD78F0730
* microcontroller which can be used as USB-UART converter.
*/
public static final int V_RNSAS = 0x0409;
/** The value indicating that the USB device can have any vendor id and product id.
*/
public static final int DEV_ANY = 0x00;
/** The value indicating that a USB device has been added into system.
*/
public static final int DEV_ADDED = 0x01;
/** The value indicating that a USB device has been removed from system.
*/
public static final int DEV_REMOVED = 0x02;
SerialComPortJNIBridge mComPortJNIBridge;
/**
* Allocates a new SerialComUSB object.
* @param mComPortJNIBridge interface to native library for serial port communication.
*/
public SerialComUSB(SerialComPortJNIBridge mComPortJNIBridge) {
this.mComPortJNIBridge = mComPortJNIBridge;
}
/**
* Gets the USB device firmware revision number as reported by USB device descriptor in its
* device descriptor using bcdDevice field.
*
* Application can get this firmware revision number and can self adopt to a particular hardware
* device. For example if a particular feature is present in firmware version 1.00 than application
* create a button in GUI, however for revision 1.11 it creates a different button in GUI window.
*
* Embedded system device vendors sometimes use bcdDevice value to indicate the 'embedded bootloader'
* version so that the firmware image flash loader program can identify the bootloader in use and use the
* appropriate protocol to flash firmware in flash memory. Typically, USB Device Firmware Upgrade (DFU)
* which is an official USB device class specification of the USB Implementers Forum is used.
*
* On custom hardware the RTS and DTR pins of USB-UART device can be used to control GPIO or boot mode
* pins to enter a particular boot mode. For example, open the host serial port, make DTR low and RTS high
* and then reset microcontroller board. The microcontroller will see levels at its boot pins and will
* enter into a particular boot mode.
*
* @param usbvid USB vendor ID to match.
* @param usbpid USB product ID to match.
* @param serialNumber serial number of USB device (case insensitive, optional) to match.
* @return firmware number revision string on success.
* @throws SerialComException if an I/O error occurs.
*/
public String[] getFirmwareRevisionNumber(int usbvid, int usbpid, String serialNumber) throws SerialComException {
if((usbvid < 0) || (usbvid > 0XFFFF)) {
throw new IllegalArgumentException("Argument usbvid can not be negative or greater than 0xFFFF !");
}
if((usbpid < 0) || (usbpid > 0XFFFF)) {
throw new IllegalArgumentException("Argument usbpid can not be negative or greater than 0xFFFF !");
}
String serial = null;
if(serialNumber != null) {
serial = serialNumber.trim().toLowerCase();
}
String[] bcdCodedRevNumber = mComPortJNIBridge.getFirmwareRevisionNumber(usbvid, usbpid, serial);
if(bcdCodedRevNumber == null) {
throw new SerialComException("Could not get the bcdDevice field of USB device descriptor. Please retry !");
}
String[] fwver = new String[bcdCodedRevNumber.length];
int firmwareRevisionNum;
for(int x=0; x < bcdCodedRevNumber.length; x++) {
firmwareRevisionNum = Integer.parseInt(bcdCodedRevNumber[x]);
fwver[x] = String.format("%x.%02x", (firmwareRevisionNum & 0xFF00) >> 8, firmwareRevisionNum & 0x00FF);
}
return fwver;
}
/**
* Read all the power management related information about a particular USB device. The returned
* instance of SerialComUSBPowerInfo class contains information about auto suspend, selective suspend,
* current power status etc.
*
*
* @param comPort serial port name/path (COMxx, /dev/ttyUSBx) which is associated with a particular
* USB CDC/ACM interface in the USB device to be analyzed for power management.
* @return an instance of SerialComUSBPowerInfo class containing operating system and device specific
* information about power management or null if given COM port does not belong to a USB
* device.
* @throws SerialComException if an I/O error occurs.
*/
public SerialComUSBPowerInfo getCDCUSBDevPowerInfo(String comPort) throws SerialComException {
if(comPort == null) {
throw new IllegalArgumentException("Argument comPort can not be null !");
}
String portNameVal = comPort.trim();
if(portNameVal.length() == 0) {
throw new IllegalArgumentException("Argument comPort can not be empty string !");
}
String[] usbPowerInfo = mComPortJNIBridge.getCDCUSBDevPowerInfo(portNameVal);
if(usbPowerInfo != null) {
if(usbPowerInfo.length > 2) {
return new SerialComUSBPowerInfo(usbPowerInfo[0], usbPowerInfo[1], usbPowerInfo[2],
usbPowerInfo[3], usbPowerInfo[4], usbPowerInfo[5]);
}
}else {
throw new SerialComException("Could not find USB devices. Please retry !");
}
return null;
}
/**
* Causes re-scan for USB devices. It is equivalent to clicking the "Scan for hardware changes"
* button in the Device Manager. Only USB hardware is checked for new devices. This can be of use
* when trying to recover devices programmatically.
*
* This is applicable to Windows operating system only.
*
* @return true on success.
* @throws SerialComException if an I/O error occurs.
*/
public boolean rescanUSBDevicesHW() throws SerialComException {
int ret = mComPortJNIBridge.rescanUSBDevicesHW();
if(ret < 0) {
throw new SerialComException("Could not cause re-scanning for hardware change. Please retry !");
}
return true;
}
/**
* Sets the latency timer value for FTDI devices. When using FTDI USB-UART devices, optimal values
* of latency timer and read/write block size may be required to obtain optimal data throughput.
*
* Note that built-in drivers in Linux kernel image may not allow changing timer values as it may have
* been hard-coded. Drivers supplied by FTDI at their website should be used if changing latency timer
* values is required by application.
*
* @return true on success.
* @throws SerialComException if an I/O error occurs.
*/
public boolean setLatencyTimer(String comPort, byte timerValue) throws SerialComException {
int ret = mComPortJNIBridge.setLatencyTimer(comPort, timerValue);
if(ret < 0) {
throw new SerialComException("Could not set the latency timer value. Please retry !");
}
return true;
}
/**
* Gets the current latency timer value for FTDI devices.
*
* @return current latency timer value.
* @throws SerialComException if an I/O error occurs.
*/
public int getLatencyTimer(String comPort) throws SerialComException {
int value = mComPortJNIBridge.getLatencyTimer(comPort);
if(value < 0) {
throw new SerialComException("Could not get the latency timer value. Please retry !");
}
return value;
}
}