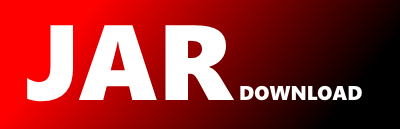
org.bidib.wizard.model.stringdata.StoredStringsImpl Maven / Gradle / Ivy
package org.bidib.wizard.model.stringdata;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class StoredStringsImpl extends Model implements StoredStrings {
private static final Logger LOGGER = LoggerFactory.getLogger(StoredStringsImpl.class);
private static final long serialVersionUID = 1L;
private final int namespace;
private int maxItems;
private int maxLength;
private final Map storedStrings = new HashMap<>();
public StoredStringsImpl(int namespace) {
this.namespace = namespace;
}
/**
* @return the namespace
*/
@Override
public int getNamespace() {
return namespace;
}
/**
* @return the maxItems
*/
@Override
public int getMaxItems() {
return maxItems;
}
/**
* @param maxItems
* the maxItems to set
*/
@Override
public void setMaxItems(int maxItems) {
int oldValue = this.maxItems;
this.maxItems = maxItems;
firePropertyChange(PROPERTY_MAX_ITEMS, oldValue, this.maxItems);
}
/**
* @return the maxLength
*/
@Override
public int getMaxLength() {
return maxLength;
}
/**
* @param maxLength
* the maxLength to set
*/
@Override
public void setMaxLength(int maxLength) {
int oldValue = this.maxLength;
this.maxLength = maxLength;
firePropertyChange(PROPERTY_MAX_LENGTH, oldValue, this.maxLength);
}
@Override
public Map getStoredStrings() {
return Collections.unmodifiableMap(storedStrings);
}
@Override
public String getStoredString(int index) {
return storedStrings.get(index);
}
@Override
public void setStoredStrings(Map storedStrings) {
Map oldValue = getStoredStrings();
this.storedStrings.clear();
if (storedStrings != null) {
this.storedStrings.putAll(storedStrings);
}
firePropertyChange(PROPERTY_STOREDSTRINGS, oldValue, this.storedStrings);
}
@Override
public void setStoredString(int index, String value) {
if (index < this.maxItems) {
String oldValue = getStoredString(index);
this.storedStrings.put(index, value);
fireIndexedPropertyChange(PROPERTY_STOREDSTRINGS, index, oldValue, value);
}
else {
LOGGER.warn("Set the stored string failed because the index is out of bounds.");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy