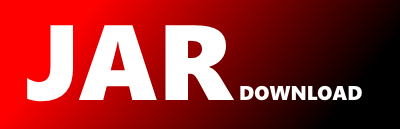
org.bidib.wizard.comm.Communication Maven / Gradle / Ivy
package org.bidib.wizard.comm;
import java.util.BitSet;
import java.util.Calendar;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.bidib.jbidibc.core.AddressData;
import org.bidib.jbidibc.core.Feature;
import org.bidib.jbidibc.core.FirmwareUpdateStat;
import org.bidib.jbidibc.core.MessageListener;
import org.bidib.jbidibc.core.Node;
import org.bidib.jbidibc.core.NodeListener;
import org.bidib.jbidibc.core.ProtocolVersion;
import org.bidib.jbidibc.core.RcPlusBindData;
import org.bidib.jbidibc.core.RcPlusUniqueIdData;
import org.bidib.jbidibc.core.SoftwareVersion;
import org.bidib.jbidibc.core.TidData;
import org.bidib.jbidibc.core.enumeration.AccessoryAcknowledge;
import org.bidib.jbidibc.core.enumeration.BoosterState;
import org.bidib.jbidibc.core.enumeration.CommandStationPom;
import org.bidib.jbidibc.core.enumeration.CommandStationPt;
import org.bidib.jbidibc.core.enumeration.CommandStationState;
import org.bidib.jbidibc.core.enumeration.FirmwareUpdateOperation;
import org.bidib.jbidibc.core.enumeration.IdentifyState;
import org.bidib.jbidibc.core.enumeration.IoBehaviourEnum;
import org.bidib.jbidibc.core.enumeration.LcMacroState;
import org.bidib.jbidibc.core.enumeration.LcOutputType;
import org.bidib.jbidibc.core.enumeration.PomAcknowledge;
import org.bidib.jbidibc.core.enumeration.RcPlusPhase;
import org.bidib.jbidibc.core.enumeration.TimeBaseUnitEnum;
import org.bidib.jbidibc.core.enumeration.TimingControlEnum;
import org.bidib.jbidibc.core.exception.InvalidConfigurationException;
import org.bidib.jbidibc.core.helpers.Context;
import org.bidib.jbidibc.core.node.ConfigurationVariable;
import org.bidib.jbidibc.core.node.listener.TransferListener;
import org.bidib.jbidibc.core.port.PortConfigValue;
import org.bidib.wizard.comm.listener.CommunicationListener;
import org.bidib.wizard.mvc.common.model.PreferencesPortType;
import org.bidib.wizard.mvc.main.model.Accessory;
import org.bidib.wizard.mvc.main.model.AnalogPort;
import org.bidib.wizard.mvc.main.model.BacklightPort;
import org.bidib.wizard.mvc.main.model.FeedbackPort;
import org.bidib.wizard.mvc.main.model.Flag;
import org.bidib.wizard.mvc.main.model.GenericPort;
import org.bidib.wizard.mvc.main.model.InputPort;
import org.bidib.wizard.mvc.main.model.LightPort;
import org.bidib.wizard.mvc.main.model.Macro;
import org.bidib.wizard.mvc.main.model.MotorPort;
import org.bidib.wizard.mvc.main.model.ServoPort;
import org.bidib.wizard.mvc.main.model.SoundPort;
import org.bidib.wizard.mvc.main.model.SwitchPort;
public interface Communication {
/**
* Open the comm port.
*
* @param commPort
* the connected comm port
*/
void open(PreferencesPortType portType, Context context);
/**
* Close the comm port.
*/
void close();
/**
* @return returns if the port is opened
*/
boolean isOpened();
/**
* @return the connected port type
*/
PreferencesPortType getConnectedPortType();
/**
* Add the message listener.
*
* @param listener
* the message listener
*/
void addMessageListener(MessageListener listener);
/**
* Remove the message listener.
*
* @param listener
* the message listener
*/
void removeMessageListener(MessageListener listener);
/**
* Add the node listener.
*
* @param nodeListener
* the node listener
*/
void addNodeListener(NodeListener nodeListener);
/**
* Remove the node listener.
*
* @param nodeListener
* the node listener
*/
void removeNodeListener(NodeListener nodeListener);
void addTransferListener(TransferListener listener);
/**
* Add the communication listener.
*
* @param listener
* the communication listener
*/
void addCommunicationListener(CommunicationListener listener);
/**
* Remove the communication listener.
*
* @param listener
* the communication listener
*/
void removeCommunicationListener(CommunicationListener listener);
/**
* Get the magic from the node. If the node does not respond correctly in the configured timespan a
* NoAnswerException is thrown.
*
* @param node
* the node
* @return the magic value
*/
int getMagic(Node node);
/**
* Returns the list of features of the specified node
*
* @param node
* the node
* @param discardCache
* discard cached values and read features from node
* @return list of features
*/
Set getFeatures(Node node, boolean discardCache);
/**
* Read a single feature of the specified node.
*
* @param node
* the node
* @param featureNumber
* the feature number
* @param omitCache
* don't get this value from cache and don't store this value in cache
* @return the feature
*/
Feature readFeature(Node node, int featureNumber, boolean omitCache);
/**
* Write a single feature to the specified node.
*
* @param node
* the node
* @param feature
* the feature
*/
void writeFeature(Node node, Feature feature);
/**
* Clear all cached data of a node
*
* @param node
* the node
*/
void clearCachedData(Node node);
void activateAnalogPort(Node node, int port, AnalogPortStatus status);
void activateLightPort(Node node, int port, LightPortStatus status);
void activateBacklightPort(Node node, BacklightPort port, int value);
void activateMotorPort(Node node, int port, MotorPortStatus status);
void activateServoPort(Node node, int port, int value);
void activateSoundPort(Node node, int port, SoundPortStatus status);
void activateSwitchPort(Node node, int port, SwitchPortStatus status);
void boosterOff(Node node);
void boosterOn(Node node);
void boosterQuery(Node node);
/**
* Set the new command station state.
*
* @param node
* the node
* @param commandStationState
* the new command station state
*/
void setCommandStationState(Node node, CommandStationState commandStationState);
/**
* Query the state of the command station.
*
* @param node
* the node
* @return the state of the command station
*/
CommandStationState queryCommandStationState(Node node);
/**
* Query the state of the booster.
*
* @param node
* the node
* @return the state of the booster
*/
BoosterState queryBoosterState(Node node);
void disable(Node node);
/**
* Release the node to allow the node to send spontaneous messages.
*
* @param node
* the node
*/
void enable(Node node);
/**
* Get the list of accessories from the node.
*
* @param node
* the node
* @return the list of accessories
*/
List getAccessories(Node node);
/**
* Get the maximum length of an accessory from the node.
*
* @param node
* the node
* @return the maximum length of an accessory
*/
int getAccessoryLength(Node node);
/**
* Get the analog ports of the node. If the analog ports are not cached, a request to get the number of analog ports
* from the bidib node is performed.
*
* @param node
* the node
* @return the collection of analog ports
*/
List getAnalogPorts(Node node);
/**
* Get the maximum booster current of the node.
*
* @param node
* the node
* @return the maximum booster current value
*/
int getBoosterMaximumCurrent(Node node);
/**
* Get the feedback ports. If the feedback ports are not cached, a request to get the number of feedback ports from
* the bidib node is performed.
*
* @param node
* the node
* @return the collection of feedback ports
*/
List getFeedbackPorts(Node node);
/**
* Get the feedback state, address state and confidence of the feedback ports
*
* @param node
* the node to query
* @param begin
* the start range of port number
* @param end
* the end range of port number
*/
void getFeedbackPortStatus(Node node, int begin, int end);
/**
* Get the flags from the node.
*
* @return the list of flags
*/
List getFlags();
/**
* Get the input ports of the node. If the input ports are not cached, a request to get the number of input ports
* from the bidib node is performed.
*
* @param node
* the node
* @return the collection of input ports
*/
List getInputPorts(Node node);
/**
* Query the input port config of the node.
*
* @param node
* the node
*/
void queryInputPortConfig(Node node);
/**
* Request signaling the status of the input port.
*
* @param node
* the node
* @param portIds
* the list of input port numbers
*/
void getInputPortStatus(Node node, List portIds);
/**
* Get the light ports of the node. If the light ports are not cached, a request to get the number of light ports
* from the bidib node is performed.
*
* @param node
* the node
* @return the collection of light ports
*/
List getLightPorts(Node node);
/**
* Query the light port config of the node.
*
* @param node
* the node
*/
void queryLightPortConfig(Node node);
/**
* Get the backlight ports of the node. If the backlight ports are not cached, a request to get the number of
* backlight ports from the bidib node is performed.
*
* @param node
* the node
* @return the collection of backlight ports
*/
List getBacklightPorts(Node node);
/**
* Query the backlight port config of the node.
*
* @param node
* the node
*/
void queryBacklightPortConfig(Node node);
/**
* Returns the supported number of macro points per macro. This value is dependent on the device that runs on the
* node.
*
* @param node
* the node
* @return the number of macro points per macro
*/
int getMacroLength(Node node);
/**
* Get the content (the macro points) of the macro.
*
* @param node
* the node
* @param macro
* the current macro instance
* @return the provided macro instance with the content retrieved from the device
* @throws InvalidConfigurationException
* if the conversion of the received data into the LcMacro failed
*/
Macro getMacroContent(org.bidib.wizard.mvc.main.model.Node node, Macro macro);
/**
* Get the macros from the node.
*
* @param node
* the node
* @return the list of macros
*/
List getMacros(Node node);
/**
* Get the motor ports of the node. If the motor ports are not cached, a request to get the number of motor ports
* from the bidib node is performed.
*
* @param node
* the node
* @return the collection of motor ports
*/
List getMotorPorts(Node node);
/**
* Get the list of nodes.
*
* @return the list of nodes
*/
List getNodes();
/**
* Load the subnodes of the provided node from the system.
*
* @param node
* the node
* @return the collection of subnodes of the provided node
*/
List loadSubNodes(Node node);
/**
* Get the subnodes of the provided node from the cached nodes.
*
* @param node
* the node
* @return the collection of subnodes of the provided node
*/
List getSubNodes(Node node);
/**
* Register a node in the list of nodes.
*
* @param node
* the node to register
*/
void registerNode(Node node);
/**
* Check if a node is already registered in the list of nodes.
*
* @param node
* the node to register
* @return true if node is already in list, false otherwise
*/
boolean isNodeRegistered(Node node);
/**
* Remove a node from the list of nodes.
*
* @param node
* the node to remove
*/
void removeNode(Node node);
/**
* Get the protocol version of the node.
*
* @param node
* the node
* @return the current protocol version of the node
*/
ProtocolVersion getProtocolVersion(Node node);
/**
* Returns the collection of servo ports in the system.
*
* @param node
* the node
* @return the collection of servo ports in the system
*/
List getServoPorts(Node node);
/**
* Query the servo port config of the node.
*
* @param node
* the node
*/
void queryServoPortConfig(Node node);
/**
* Check if the query port feature is available and enabled.
*
* @param node
* the node
* @return the port supports query port requests
*/
boolean isQueryPortStatusEnabled(Node node);
/**
* Query the port status of the specified port.
*
* @param lcOutputType
* the port type
* @param node
* the node
* @param port
* the port number
*/
void queryPortStatus(LcOutputType lcOutputType, Node node, int port);
/**
* Query the port status of the specified port.
*
* @param lcOutputType
* the port type
* @param node
* the node
* @param portIds
* the port ids
*/
void queryPortStatus(LcOutputType lcOutputType, Node node, List portIds);
/**
* Returns the collection of sound ports in the system.
*
* @param node
* the node
* @return the collection of sound ports in the system
*/
List getSoundPorts(Node node);
/**
* Returns the number of storable macro from the node.
*
* @param node
* the node
* @return the number of storable macro
*/
int getStorableMacroCount(Node node);
/**
* Returns the collection of switch ports in the system.
*
* @param node
* the node
* @return the collection of switch ports in the system
*/
List getSwitchPorts(Node node);
/**
* Query the switch port config of the node.
*
* @param node
* the node
*/
void querySwitchPortConfig(Node node);
/**
* Get the software version of the node
*
* @param node
* the node
* @return the current software version
*/
SoftwareVersion getSoftwareVersion(Node node);
/**
* Sets the identify state of the node (lets the id led blink).
*
* @param node
* the node
* @param state
* the identify state
*/
void identify(Node node, IdentifyState state);
Boolean isAddressMessagesEnabled(Node node);
Boolean isBooster(Node node);
Boolean isCommandStation(Node node);
Boolean isDccStartEnabled(Node node);
Boolean isExternalStartEnabled(Node node);
Boolean isFeedbackMessagesEnabled(Node node);
Boolean isKeyMessagesEnabled(Node node);
/**
* Check if the node supports FW update
*
* @param node
* the node
* @return the node supports FW update
*/
boolean isUpdatable(Node node);
/**
* Returns the list of configuration variables (CV) of the specified node. The variables are fetched from a cache if
* already availble.
*
* @param node
* the node
* @return list of configuration variables (CV)
*/
List getConfigurationVariables(Node node, List configVariables);
/**
* Set a string value in the node
*
* @param node
* the node
* @param namespace
* the namespace (default: 0)
* @param index
* the index
* @param value
* the string value to set
* @return the string value returned from the node
*/
String setString(Node node, int namespace, int index, String value);
/**
* Get a string value from the node
*
* @param node
* the node
* @param namespace
* the namespace (default: 0)
* @param index
* the index
* @return the string value
*/
String getString(Node node, int namespace, int index);
/**
* Writes the CV values of the provided list to the node.
*
* @param node
* the node
* @param configVariables
* the list of configuration variables (CV)
* @return the list with the changed values
*/
List writeConfigurationVariables(Node node, List configVariables);
/**
* Read the value of the configuration variables (CV) directly from the specified node without using the cache.
*
* @param node
* the node
* @param cvNumber
* the CV number
* @return the CV value
*/
// TODO CV number and value can be Strings ...
int readCv(Node node, int cvNumber);
/**
* Send a CV POM request.
*
* @param node
* the node
* @param locoAddress
* the loco address
* @param opCode
* the opCode
* @param cvNumber
* the CV number
* @param cvValue
* the CV value to write (ignored for read opCodes)
* @return the acknowledge value
*/
PomAcknowledge sendCvPomRequest(
Node node, AddressData locoAddress, CommandStationPom opCode, int cvNumber, int cvValue);
/**
* Send a read CV POM request.
*
* @param node
* the node
* @param locoAddress
* the loco address
* @param opCode
* the opCode
* @param cvNumber
* the CV number
* @return the acknowledge value
*/
PomAcknowledge sendReadCvPomRequest(Node node, AddressData locoAddress, CommandStationPom opCode, int cvNumber);
/**
* Send a CV PT request.
*
* @param node
* the node
* @param opCode
* the opCode
* @param cvNumber
* the CV number
* @param cvValue
* the CV value
*/
void sendCvPtRequest(Node node, CommandStationPt opCode, int cvNumber, int cvValue);
/**
* Reload the accessory from the node.
*
* @param node
* the node
* @param accessory
* the accessory
*/
void reloadAccessory(Node node, Accessory accessory);
/**
* Store accessory on the node.
*
* @param node
* the node
* @param accessory
* the accessory
*/
void saveAccessory(Node node, Accessory accessory);
/**
* Send the FW update operation to the node.
*
* @param node
* the node
* @param operation
* the operation
* @param data
* the data
* @return the status
*/
FirmwareUpdateStat sendFirmwareUpdateOperation(Node node, FirmwareUpdateOperation operation, byte... data);
/**
* Reset the node.
*
* @param node
* the node, if null the root node is reset
*/
void reset(Node node);
/**
* Ping the node.
*
* @param node
* the node
* @param data
* the data byte
*/
void ping(Node node, byte data);
/**
* Send the current model time to the system.
*
* @param time
* the current model time
* @param factor
* the acceleration factor
*/
void sendTime(Calendar time, int factor);
void setAddressMessagesEnabled(Node node, boolean enabled);
void setBinaryState(Node node, int address, int state, boolean value);
void setDccStartEnabled(Node node, boolean enabled);
void setExternalStartEnabled(Node node, boolean enabled);
void setFeedbackMessagesEnabled(Node node, boolean enabled);
void setKeyMessagesEnabled(Node node, boolean enabled);
void setLightPortParameters(Node node, int port, int pwmMin, int pwmMax, int dimMin, int dimMax, Integer rgbValue);
void setBacklightPortParameters(Node node, int port, int dimSlopeDown, int dimSlopeUp, int dmxMapping);
void setServoPortParameters(Node node, int port, int trimDown, int trimUp, int speed);
/**
* Set the switch port parameters.
*
* @param node
* the node
* @param port
* the port number
* @param ioBehaviour
* the IO behaviour: 0=output (direct controlled) (default setting), 1=High pulse (if turn on (1), the
* output will go off (0) alone after the given time S). 2=Low pulse, 3=tristate, 4=pullup, 5=pulldown
* @param switchOffTime
* time S
*/
void setSwitchPortParameters(Node node, int port, IoBehaviourEnum ioBehaviour, int switchOffTime);
/**
* Set the port parameters and allow to switch the port to a new type.
*
* @param node
* the node
* @param port
* the port number
* @param portType
* the port type to change (optional). If null
the port type is not changed
* @param values
* the other configuration values
*/
void setPortParameters(Node node, int port, LcOutputType portType, Map> values);
/**
* Set the speed of a loco decoder.
*
* @param node
* the node
* @param address
* the DCC address of the loco decoder
* @param speedSteps
* the speed steps
* @param speed
* the speed
* @param direction
* the direction to drive
* @param activeFunctions
* active functions
* @param functions
* functions
*/
void setSpeed(
Node node, int address, SpeedSteps speedSteps, Integer speed, Direction direction, BitSet activeFunctions,
BitSet functions);
/**
* Set the DCC accessory decoder to the aspect (only accessory decoder for DCC!).
*
* @param node
* the node
* @param dccAddress
* the DCC address of the accessory decoder
* @param aspect
* the aspect
* @param switchTime
* the switch time
* @param timeBaseUnit
* the time base unit (100ms or 1s)
* @param timingControl
* the timing control
* @return the accessory acknowledge
*/
AccessoryAcknowledge setDccAccessory(
Node node, AddressData dccAddress, int aspect, Integer switchTime, TimeBaseUnitEnum timeBaseUnit,
TimingControlEnum timingControl);
/**
* Set the accessory to the aspect.
*
* @param node
* the node
* @param accessory
* the accessory
* @param ascpect
* the aspect
*/
void startAccessory(Node node, Accessory accessory, int ascpect);
/**
* Query the state of the accessory.
*
* @param node
* the node
* @param accessory
* the accessory
*/
void queryAccessoryState(Node node, Accessory... accessory);
/**
* Start the macro.
*
* @param node
* the node
* @param macro
* the macro
* @param transferBeforeStart
* transfer the macro before start
* @return the macro state
*/
LcMacroState startMacro(org.bidib.wizard.mvc.main.model.Node node, Macro macro, boolean transferBeforeStart);
LcMacroState stopMacro(Node node, Macro macro);
/**
* Transfer the macro to the node and store in permanent memory.
*
* @param node
* the node
* @param macro
* the macro
*/
LcMacroState saveMacro(org.bidib.wizard.mvc.main.model.Node node, Macro macro);
/**
* Load the macro with the specified macro id from the node.
*
* @param node
* the node
* @param macro
* the macro
*/
LcMacroState reloadMacro(org.bidib.wizard.mvc.main.model.Node node, Macro macro);
/**
* Transfer the macro to the node but don't store in permanent memory.
*
* @param node
* the node
* @param macro
* the macro
*/
void transferMacro(org.bidib.wizard.mvc.main.model.Node node, Macro macro);
/**
* Set the new resetReconnectDelay
*
* @param resetReconnectDelay
* the new resetReconnectDelay
*/
void setResetReconnectDelay(int resetReconnectDelay);
/**
* Set the response timeout that is used to wait for response from node.
*
* @param responseTimeout
* the new response timeout value
*/
void setResponseTimeout(int responseTimeout);
/**
* Query the port config of the node.
*
* @param node
* the node
* @param ports
* the ports to query
*/
void queryPortConfig(Node node, List ports);
/**
* Query all port config values of the node.
*
* @param node
* the node
* @param outputType
* the port type
* @param rangeFrom
* start number of port that is transmitted (included range)
* @param rangeTo
* end number of port that is not transmitted (excluded range)
*/
void queryAllPortConfig(Node node, LcOutputType outputType, Integer rangeFrom, Integer rangeTo);
/**
* Check if the node has the RailCom+ feature available.
*
* @param node
* the node
* @return the RailCom+ feature is available
*/
Boolean isRailComPlusAvailable(Node node);
/**
* Get the Railcom+ TID from the command station node. The TID is signaled asynchronously.
*
* @param node
* the node
*/
void getRcPlusTid(Node node);
/**
* Set the Railcom+ TID on the command station node.
*
* @param node
* the node
* @param tid
* the TID to set
*/
void setRcPlusTid(Node node, TidData tid);
/**
* Send the PING_ONCE command with the specified phase.
*
* @param node
* the node
* @param phase
* the phase
*/
void sendPingOnce(Node node, RcPlusPhase phase);
/**
* Send the PING command with the specified interval.
*
* @param node
* the node
* @param interval
* the repeat interval in units of 100ms, a value of 0 stops the sending of PINGs
*/
void sendPing(Node node, int interval);
/**
* Send the FIND command with the specified phase.
*
* @param node
* the node
* @param phase
* the phase
* @param decoder
* the decoder MUN and MID
*/
void sendFind(Node node, RcPlusPhase phase, RcPlusUniqueIdData decoder);
/**
* Send the BIND command with the specified bindData.
*
* @param node
* the node
* @param bindData
* the decoder MUN and MID, and the address
*/
void sendBind(Node node, RcPlusBindData bindData);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy