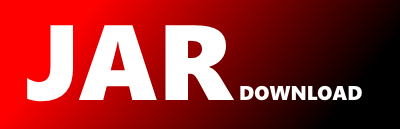
org.bidib.wizard.dialog.AboutDialog Maven / Gradle / Ivy
package org.bidib.wizard.dialog;
import java.awt.Component;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.io.IOException;
import java.util.Properties;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import javax.swing.JTextArea;
import javax.swing.SwingConstants;
import javax.swing.UIManager;
import org.bidib.wizard.locale.Resources;
public class AboutDialog {
private final Properties version = new Properties();
/**
* Create new instance of AboutDialog.
*
* @param parentComponent
* the parent component
* @throws IOException
*/
public AboutDialog(Component parentComponent) throws IOException {
version.load(getClass().getResourceAsStream("/build.properties"));
JOptionPane pane = new JOptionPane(getPanel(), JOptionPane.INFORMATION_MESSAGE);
JDialog dialog =
pane.createDialog(parentComponent,
Resources.getString(getClass(), "title") + " " + version.getProperty("projectname"));
dialog.setVisible(true);
}
private JPanel getPanel() throws IOException {
JPanel result = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.anchor = GridBagConstraints.FIRST_LINE_START;
c.fill = GridBagConstraints.NONE;
c.gridwidth = 2;
c.gridx = 0;
c.gridy = 0;
c.insets = new Insets(5, 5, 5, 5);
result.add(new JLabel(version.getProperty("projectname")), c);
c.gridwidth = 1;
c.gridy++;
result.add(new JSeparator(SwingConstants.HORIZONTAL), c);
c.gridy++;
result.add(new JLabel(Resources.getString(getClass(), "authors") + ":"), c);
JTextArea authors = new JTextArea(version.getProperty("projectauthor"));
authors.setFont(UIManager.getFont("Label.font"));
authors.setEditable(false);
authors.setOpaque(false);
c.gridx++;
result.add(authors, c);
c.gridx = 0;
c.gridy++;
result.add(new JLabel(Resources.getString(getClass(), "version") + ":"), c);
c.gridx++;
result.add(new JLabel(version.getProperty("projectversion")), c);
c.gridx = 0;
c.gridy++;
result.add(new JLabel(Resources.getString(getClass(), "build") + ":"), c);
c.gridx++;
result.add(new JLabel(version.getProperty("buildNumber")), c);
c.gridx = 0;
c.gridy++;
result.add(new JLabel(Resources.getString(getClass(), "date") + ":"), c);
c.gridx++;
result.add(new JLabel(version.getProperty("projectdate")), c);
c.gridx = 0;
c.gridy++;
result.add(new JLabel(Resources.getString(getClass(), "java") + ":"), c);
c.gridx++;
result.add(new JLabel(System.getProperty("java.version")), c);
c.gridx = 0;
c.gridy++;
c.gridwidth = 2;
result.add(new JLabel("Icons provided by http://www.famfamfam.com/lab/icons/silk/"), c);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy