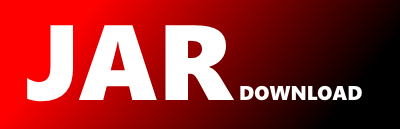
org.bidib.wizard.dialog.NodeDetailsDialog Maven / Gradle / Ivy
package org.bidib.wizard.dialog;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.util.Arrays;
import java.util.Collection;
import javax.swing.JDialog;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.UIManager;
import org.bidib.jbidibc.core.Feature;
import org.bidib.jbidibc.core.ProtocolVersion;
import org.bidib.jbidibc.core.SoftwareVersion;
import org.bidib.jbidibc.core.utils.NodeUtils;
import org.bidib.wizard.comm.Communication;
import org.bidib.wizard.locale.Resources;
import org.bidib.wizard.mvc.main.model.Node;
/**
* Dialog that displays node details.
*
*/
public class NodeDetailsDialog {
public NodeDetailsDialog(Node node, Communication communication, int x, int y) {
String label = node.toString();
byte[] address = node.getNode().getAddr();
String uuid = NodeUtils.getUniqueIdAsString(node.getNode().getUniqueId());
// TODO check if we can make this call asynchronous because it currently
// blocks the AWT thread from updating the UI
SoftwareVersion swVersion = communication.getSoftwareVersion(node.getNode());
ProtocolVersion pVersion = communication.getProtocolVersion(node.getNode());
Collection features = null;
if (!node.isBootloaderNode()) {
// only fetch the features if node is not a bootloader node
features = communication.getFeatures(node.getNode(), false);
}
JOptionPane pane =
new JOptionPane(getPanel(label, address, swVersion, pVersion, uuid, features),
JOptionPane.INFORMATION_MESSAGE);
JDialog dialog = pane.createDialog(null, Resources.getString(getClass(), "title"));
dialog.setLocation(x, y);
dialog.setVisible(true);
}
private String getFeatureName(String name) {
String result = name;
if (result.startsWith("FEATURE_")) {
result = result.substring(8);
}
return result;
}
private JPanel getPanel(
String label, byte[] address, SoftwareVersion swVersion, ProtocolVersion pVersion, String uuid,
Collection features) {
JPanel result = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.anchor = GridBagConstraints.FIRST_LINE_START;
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy = 0;
c.insets = new Insets(5, 5, 5, 5);
result.add(new JLabel(Resources.getString(getClass(), "label") + ":"), c);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(new JLabel(label), c);
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy++;
c.weightx = 0;
result.add(new JLabel(Resources.getString(getClass(), "address") + ":"), c);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(new JLabel(Arrays.toString(address)), c);
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy++;
c.weightx = 0;
result.add(new JLabel(Resources.getString(getClass(), "software") + ":"), c);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(new JLabel(String.valueOf(swVersion)), c);
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy++;
c.weightx = 0;
result.add(new JLabel(Resources.getString(getClass(), "protocol") + ":"), c);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(new JLabel(String.valueOf(pVersion)), c);
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy++;
c.weightx = 0;
result.add(new JLabel(Resources.getString(getClass(), "uuid") + ":"), c);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(new JLabel(uuid), c);
if (features != null) {
// add features if available
c.fill = GridBagConstraints.NONE;
c.gridx = 0;
c.gridy++;
c.weightx = 0;
result.add(new JLabel(Resources.getString(getClass(), "features") + ":"), c);
StringBuilder featuresString = new StringBuilder();
for (Feature feature : features) {
featuresString.append(getFeatureName(feature.getFeatureName()));
featuresString.append(" : ");
featuresString.append(feature.getValue());
featuresString.append('\n');
}
JTextArea featuresTextArea = new JTextArea(featuresString.toString());
featuresTextArea.setEditable(false);
featuresTextArea.setOpaque(false);
featuresTextArea.setFont(UIManager.getFont("Label.font"));
featuresTextArea.setEditable(false);
c.fill = GridBagConstraints.HORIZONTAL;
c.gridx++;
c.weightx = 1;
result.add(featuresTextArea, c);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy