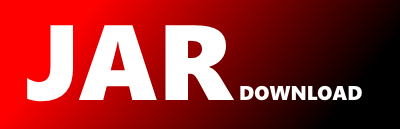
org.bidib.wizard.dk.nullesoft.Airlog.LED Maven / Gradle / Ivy
/*
* $Id: LED.java,v 1.1 2012-05-15 21:38:31 schenk Exp $
*
* Copyright (c) 1999 Per Jensen.
*
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
* If you modify this file, please send us a copy.
*/
package org.bidib.wizard.dk.nullesoft.Airlog;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Insets;
import javax.swing.JComponent;
/**
* Synopsis: LED button will flash in different colors at specified intervals
* License: GNU GPL2, look for details in file "COPYING"
*
*
*
* @author Per Jensen, [email protected]
*/
public class LED extends JComponent implements Runnable {
private static final long serialVersionUID = 1L;
private Color c, c1, c2;
private Insets insets;
private int interv, curWidth, curHeight;
/** If LED is resized, set calculatePaintArea to true in order to update */
public boolean calculatePaintArea = true;
private boolean show = false;
private boolean goOn = true;
private Thread t;
private boolean rounded;
private int index;
/**
* Create a LED which switches between red and gray.
* Switch period = 1000 milli seconds. Color is set to gray
*/
public LED() {
this(Color.red, Color.gray, 1000);
}
/**
* Create a LED which switches between primary and secondary color.
* Switch interval is in milli seconds. Color is initated to the secondary color
*
* @param primary
* the primary color
* @param secondary
* the secondary color
* @param interval
* interval in milliseconds between color switch, no less than 200
*/
public LED(Color primary, Color secondary, int interval) {
c1 = primary;
c2 = secondary;
setPreferredSize(new Dimension(15, 15));
c = c2;
repaint();
if (interval > 200) {
interv = interval;
}
else {
interv = 200;
}
t = new Thread(this);
}
/**
* Create a LED which doesn't blink.
*
* @param primary
* the primary color
* @param secondary
* the secondary color
*/
public LED(Color primary, Color secondary, boolean rounded) {
super();
this.rounded = rounded;
c1 = primary;
c2 = secondary;
c = c2;
interv = 100000;
setPreferredSize(new Dimension(15, 15));
repaint();
t = new Thread(this);
}
public void setIndex(int index) {
this.index = index;
}
public int getIndex() {
return index;
}
public void usePrimary() {
c = c1;
revalidate();
repaint();
}
public void useSecondary() {
c = c2;
revalidate();
repaint();
}
public void toggleColor() {
if (c == c1) {
useSecondary();
}
else {
usePrimary();
}
}
public boolean isUsePrimary() {
return c == c1;
}
protected void paintComponent(Graphics g) {
if (calculatePaintArea) {
insets = getInsets();
curWidth = getWidth() - insets.left - insets.right - 1;
curHeight = getHeight() - insets.top - insets.bottom - 1;
calculatePaintArea = false;
}
super.paintComponent(g);
g.setColor(c);
if (rounded) {
g.fillOval(insets.left, insets.top, curWidth, curHeight);
}
else {
g.fillRect(insets.left, insets.top, curWidth, curHeight);
}
}
/**
* Thread switches between the specified colors. Sleeps between switches. Switch interval is in milli seconds. If a
* stop command has been issued ealier, the LED will turn gray.
*/
public void run() {
try {
while (true) {
if (!goOn) {
c = Color.gray;
repaint();
break;
}
show = !show;
if (show) {
c = c1;
}
else {
c = c2;
}
repaint();
Thread.sleep(interv);
}
}
catch (InterruptedException ie) {
throw new RuntimeException(ie);
}
}
/**
* Stop switching between two colors. Thread will die in max interval milliseconds
*/
public void stop() {
goOn = false;
}
/**
* Start switching between two colors.
*/
public void start() {
// don't make a new thread, if we have one already
if (!t.isAlive()) {
goOn = true;
t = new Thread(this, "LED");
t.start();
}
else {
goOn = true;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy