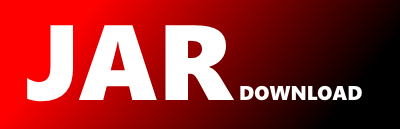
org.bidib.wizard.locale.Resources Maven / Gradle / Ivy
package org.bidib.wizard.locale;
import java.net.URL;
import java.text.MessageFormat;
import java.util.MissingResourceException;
import java.util.ResourceBundle;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Helper class for localization.
*
* @author André Schenk
*/
public final class Resources {
private static final Logger LOGGER = LoggerFactory.getLogger(Resources.class);
private static Resources resources = new Resources();
private ResourceBundle properties;
private static final String WIZARD_PACKAGE = "org.bidib.wizard.";
private static final int START_POS = WIZARD_PACKAGE.length();
private Resources() {
properties = ResourceBundle.getBundle("catalogs/BiDiBWizard_locale");
}
private static synchronized Resources getInstance() {
return resources;
}
private static ResourceBundle getResources() {
return getInstance().properties;
}
public static String getString(Class> clazz, String key) {
String result = null;
ResourceBundle properties = getResources();
String resourceName = null;
if (clazz != null) {
if (clazz.getName().startsWith(WIZARD_PACKAGE)) {
resourceName = clazz.getName().substring(START_POS) + "." + key;
}
else {
resourceName = clazz.getName() + "." + key;
}
}
else {
resourceName = key;
}
if (properties != null) {
try {
result = properties.getString(resourceName);
}
catch (MissingResourceException e) {
result = "<" + resourceName + ">";
}
}
else {
result = resourceName;
}
return result;
}
public static String getString(Class> clazz, String key, Object[] arguments) {
String result = null;
String template = getString(clazz, key);
if (template != null) {
result = new MessageFormat(template).format(arguments);
}
return result;
}
public static JButton makeNavigationButton(String imageName, String toolTipText, String altText) {
// Look for the image.
String imgLocation = "/icons/" + imageName;
URL imageURL = Resources.class.getResource(imgLocation);
// Create and initialize the button.
JButton button = new JButton();
button.setToolTipText(toolTipText);
if (imageURL != null) { // image found
button.setIcon(new ImageIcon(imageURL, altText));
}
else { // no image found
button.setText(altText);
LOGGER.warn("Resource not found: {}", imgLocation);
}
return button;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy