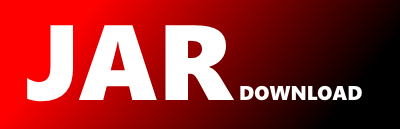
org.bidib.wizard.mvc.booster.model.BoosterModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.booster.model;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import org.apache.commons.lang.StringUtils;
import org.bidib.jbidibc.core.Node;
import org.bidib.jbidibc.core.StringData;
import org.bidib.jbidibc.core.enumeration.BoosterState;
import org.bidib.wizard.mvc.main.model.NodeLabels;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class BoosterModel extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(BoosterModel.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTY_BOOSTER_STATUS = "boosterStatus";
public static final String PROPERTY_BOOSTER_CURRENT = "boosterCurrent";
public static final String PROPERTY_BOOSTER_MAX_CURRENT = "boosterMaxCurrent";
public static final String PROPERTY_BOOSTER_VOLTAGE = "boosterVoltage";
public static final String PROPERTY_BOOSTER_TEMPERATURE = "boosterTemperature";
private final Node booster;
private NodeLabels nodeLabels;
private BoosterState state;
private int maxCurrent;
private int current;
private int voltage;
private int temperature;
private String nodeLabel;
private long lastCurrentUpdate;
private PropertyChangeListener propertyChangeListener;
public BoosterModel(final Node booster, final NodeLabels nodeLabels) {
this.booster = booster;
this.nodeLabels = nodeLabels;
LOGGER.info("Create new BoosterModel for booster: {}", booster);
}
public void registerNode() {
propertyChangeListener = new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
// update the label with the name ...
LOGGER.debug("Property has changed, name: {}, value: {}", evt.getPropertyName(), evt.getNewValue());
String nodeLabel = prepareNodeLabel();
setNodeLabel(nodeLabel);
}
};
this.booster.addPropertyChangeListener(propertyChangeListener);
}
public void freeNode() {
this.booster.removePropertyChangeListener(propertyChangeListener);
}
/**
* @return the booster node
*/
public Node getBooster() {
return booster;
}
/**
* @return the node address
*/
public byte[] getNodeAddress() {
return booster.getAddr();
}
/**
* @param nodeLabel
* the node label to set
*/
public void setNodeLabel(String nodeLabel) {
this.nodeLabel = nodeLabel;
}
/**
* @return the node label
*/
public String getNodeLabel() {
return nodeLabel;
}
/**
* @return the state
*/
public BoosterState getState() {
return state;
}
/**
* @param state
* the state to set
*/
public void setState(BoosterState state) {
BoosterState oldValue = this.state;
this.state = state;
firePropertyChange(PROPERTY_BOOSTER_STATUS, oldValue, this.state);
}
/**
* @return the maxCurrent
*/
public int getMaxCurrent() {
return maxCurrent;
}
/**
* @param maxCurrent
* the maxCurrent to set
*/
public void setMaxCurrent(int maxCurrent) {
int oldValue = this.maxCurrent;
// do not convert value
this.maxCurrent = maxCurrent;
firePropertyChange(PROPERTY_BOOSTER_MAX_CURRENT, oldValue, this.maxCurrent);
}
/**
* @return the current
*/
public int getCurrent() {
return current;
}
/**
* @param current
* the current to set
*/
public void setCurrent(int current) {
int oldValue = this.current;
this.current = current;
// set the last current update time
setLastCurrentUpdate(System.currentTimeMillis());
firePropertyChange(PROPERTY_BOOSTER_CURRENT, oldValue, this.current);
}
/**
* @return the lastCurrentUpdate
*/
public long getLastCurrentUpdate() {
return lastCurrentUpdate;
}
/**
* @param lastCurrentUpdate
* the lastCurrentUpdate to set
*/
public void setLastCurrentUpdate(long lastCurrentUpdate) {
this.lastCurrentUpdate = lastCurrentUpdate;
}
/**
* @return the voltage
*/
public int getVoltage() {
return voltage;
}
/**
* @param voltage
* the voltage to set
*/
public void setVoltage(int voltage) {
int oldValue = this.voltage;
this.voltage = voltage;
firePropertyChange(PROPERTY_BOOSTER_VOLTAGE, oldValue, this.voltage);
}
/**
* @return the temperature
*/
public int getTemperature() {
return temperature;
}
/**
* @param temperature
* the temperature to set
*/
public void setTemperature(int temperature) {
int oldValue = this.temperature;
this.temperature = temperature;
firePropertyChange(PROPERTY_BOOSTER_TEMPERATURE, oldValue, this.temperature);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof BoosterModel) {
BoosterModel other = (BoosterModel) obj;
if (booster.equals(other.getBooster())) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
return booster.hashCode();
}
public String prepareNodeLabel() {
String nodeLabel = nodeLabels.getLabel(booster.getUniqueId());
if (StringUtils.isBlank(nodeLabel)) {
// try to get the product name
String productString = booster.getStoredString(StringData.INDEX_PRODUCTNAME);
if (StringUtils.isNotBlank(productString)) {
nodeLabel = productString;
}
}
return nodeLabel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy