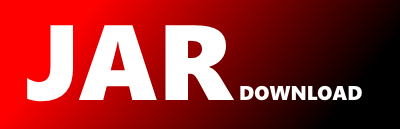
org.bidib.wizard.mvc.dmx.controller.DmxModelerController Maven / Gradle / Ivy
package org.bidib.wizard.mvc.dmx.controller;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
import org.apache.commons.collections.CollectionUtils;
import org.bidib.jbidibc.core.node.ConfigurationVariable;
import org.bidib.jbidibc.core.utils.ProductUtils;
import org.bidib.wizard.comm.CommunicationFactory;
import org.bidib.wizard.mvc.dmx.controller.listener.DmxModelerControllerListener;
import org.bidib.wizard.mvc.dmx.model.DmxChannel;
import org.bidib.wizard.mvc.dmx.model.DmxLightPort;
import org.bidib.wizard.mvc.dmx.model.DmxScenery;
import org.bidib.wizard.mvc.dmx.model.DmxSceneryModel;
import org.bidib.wizard.mvc.dmx.view.DmxModelerView;
import org.bidib.wizard.mvc.dmx.view.DmxSceneryView;
import org.bidib.wizard.mvc.main.model.BacklightPort;
import org.bidib.wizard.mvc.main.model.LightPort;
import org.bidib.wizard.mvc.main.model.Macro;
import org.bidib.wizard.mvc.main.model.MainModel;
import org.bidib.wizard.mvc.main.model.Node;
import org.bidib.wizard.mvc.main.model.Port;
import org.bidib.wizard.mvc.main.model.listener.NodeListListener;
import org.bidib.wizard.mvc.main.view.cvdef.NodeNode;
import org.bidib.wizard.mvc.main.view.panel.CvDefinitionTreeHelper;
import org.bidib.wizard.utils.DockUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.vlsolutions.swing.docking.Dockable;
import com.vlsolutions.swing.docking.DockingConstants;
import com.vlsolutions.swing.docking.DockingDesktop;
import com.vlsolutions.swing.docking.RelativeDockablePosition;
public class DmxModelerController implements DmxModelerControllerListener, NodeListListener {
private static final Logger LOGGER = LoggerFactory.getLogger(DmxModelerController.class);
private final JFrame parent;
private final DockingDesktop desktop;
private MainModel mainModel;
private DmxSceneryModel dmxSceneryModel;
private DmxModelerView view;
private Node node;
public DmxModelerController(final JFrame parent, final DockingDesktop desktop, final Node node) {
this.parent = parent;
this.desktop = desktop;
this.node = node;
dmxSceneryModel = new DmxSceneryModel();
}
public void start(MainModel mainModel) {
this.mainModel = mainModel;
LOGGER.info("Start controller.");
List dmxChannels = new LinkedList<>();
for (int channelId = 0; channelId < 64; channelId++) {
// + 1 because DMX channel id starts from 1
dmxChannels.add(new DmxChannel(channelId + 1));
}
dmxSceneryModel.setDmxChannels(dmxChannels);
List macros = new LinkedList<>();
macros.add(null);
macros.addAll(mainModel.getMacros());
dmxSceneryModel.setMacros(macros);
// get some CV values from OneDMX
if (ProductUtils.isOneDMX(node.getUniqueId())) {
LOGGER.info("The current node is a OneDMX.");
// do not get all CV values from the OneDMX because the other values are loaded via MSG_LC_CONFIG messages
// get the initial state for the DMX channels
if (CollectionUtils.isNotEmpty(dmxChannels)) {
Map dmxChannelMap = null;
dmxChannelMap =
new CvDefinitionTreeHelper().getNodes(node, "Config\\DMX channels (init)", NodeNode.COLUMN_KEY,
false, null);
if (dmxChannelMap == null) {
dmxChannelMap =
new CvDefinitionTreeHelper().getNodes(node, "Config\\DMX-Channels", NodeNode.COLUMN_KEY, false,
null);
}
LOGGER.info("Fetched dmxChannelMap: {}", dmxChannelMap);
List cvList = new LinkedList(dmxChannelMap.values());
cvList = CommunicationFactory.getInstance().getConfigurationVariables(node.getNode(), cvList);
LOGGER.info("Fetched cvList: {}", cvList);
for (DmxChannel dmxChannel : dmxChannels) {
int channelId = dmxChannel.getChannelId();
// -1 because the DMX channelId starts from 1
ConfigurationVariable cv = cvList.get(channelId - 1);
if (!cv.isTimeout()) {
int initialState = Integer.parseInt(cv.getValue());
if (initialState > -1 && initialState < 256) {
dmxChannel.setInitialState(initialState);
}
}
}
}
// get the DMX target channel of the lightports
Map lightPortMap = null;
lightPortMap =
new CvDefinitionTreeHelper().getNodes(node, "Config\\Light ports", NodeNode.COLUMN_KEY, false, null);
LOGGER.trace("returned lightPortMap: {}", lightPortMap);
if (lightPortMap != null && lightPortMap.size() > 0) {
List cvList = new LinkedList(lightPortMap.values());
cvList = CommunicationFactory.getInstance().getConfigurationVariables(node.getNode(), cvList);
LOGGER.info("Fetched cvList: {}", cvList);
// the list of configured light ports
List lightPorts = mainModel.getLightPorts();
// the list of DMX wrapped light ports
List dmxLightPorts = new LinkedList<>();
// assign the mapped DMX channel
for (int index = 0; index < lightPorts.size(); index++) {
ConfigurationVariable cv = cvList.get(index);
LOGGER.info("Current cv: {}", cv);
LightPort lightPort = lightPorts.get(index);
// create a new wrapper around the lightport
DmxLightPort dmxLightPort = new DmxLightPort(lightPort);
try {
int channelId = Integer.parseInt(cv.getValue());
if (channelId > -1 && channelId < dmxChannels.size()) {
// channelId because DMX channels starts from 0 in CVs
DmxChannel dmxTargetChannel = dmxChannels.get(channelId);
dmxLightPort.setDmxTargetChannel(dmxTargetChannel);
}
else {
LOGGER.debug("Invalid channel id for dmxChannel: {}", channelId);
}
}
catch (IndexOutOfBoundsException | NumberFormatException ex) {
LOGGER.warn("Set DMX target channel failed.", ex);
}
dmxLightPorts.add(dmxLightPort);
}
dmxSceneryModel.setLightPorts(dmxLightPorts);
}
else {
LOGGER.warn("No lightports configured.");
}
}
dmxSceneryModel.setBacklightPorts(new LinkedList<>(mainModel.getBacklightPorts()));
// check if we must open the scenery panel
Dockable dmxScenery = desktop.getContext().getDockableByKey("DmxSceneryView");
if (dmxScenery == null) {
LOGGER.info("Open the DMX scenery panel.");
DmxSceneryView dmxSceneryView = new DmxSceneryView(desktop, node, dmxSceneryModel);
dmxSceneryView.setDmxModelerControllerListener(this);
dmxSceneryView.createPanel();
Dockable nodeListPanel = desktop.getContext().getDockableByKey("nodeListPanel");
desktop.split(nodeListPanel, dmxSceneryView, DockingConstants.SPLIT_BOTTOM, 0.2);
}
else {
LOGGER.info("DMX scenery is already available.");
}
mainModel.addNodeListListener(this);
}
@Override
public void openView(final DmxScenery dmxScenery) {
// check if the scenery is already opened
String searchKey = DmxModelerView.prepareKey(dmxScenery);
LOGGER.info("Search for view with key: {}", searchKey);
Dockable dmxModelerView = desktop.getContext().getDockableByKey(searchKey);
if (dmxModelerView == null) {
LOGGER.info("Create new DmxModelerView.");
// TODO check if it would be better to provide the DmxSceneryModel instead of the node
// because the DmxSceneryModel has the macros of the node
view = new DmxModelerView(desktop, dmxScenery, dmxSceneryModel);
Dockable tabPanel = desktop.getContext().getDockableByKey("tabPanel");
if (tabPanel != null) {
desktop.createTab(tabPanel, view, 1, true);
}
else {
desktop.addDockable(view, RelativeDockablePosition.RIGHT);
}
// load the scenery points
view.loadSceneryPoints();
}
else {
LOGGER.info("Select the existing modeler view.");
DockUtils.selectWindow(dmxModelerView);
}
}
@Override
public void closeView(final DmxSceneryModel dmxSceneryModel) {
// check if the scenery is opened
for (DmxScenery dmxScenery : dmxSceneryModel.getSceneries()) {
String searchKey = DmxModelerView.prepareKey(dmxScenery);
LOGGER.info("Search for view with key: {}", searchKey);
Dockable dmxModelerView = desktop.getContext().getDockableByKey(searchKey);
if (dmxModelerView != null) {
LOGGER.info("Close the dmxModelerView: {}", dmxModelerView);
desktop.close(dmxModelerView);
}
}
}
@Override
public void listChanged() {
LOGGER.info("The node list has changed.");
}
@Override
public void nodeChanged() {
LOGGER.debug("The node has changed, current node in model: {}", node);
if (SwingUtilities.isEventDispatchThread()) {
internalNodeChanged();
}
else {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
internalNodeChanged();
}
});
}
}
private void internalNodeChanged() {
LOGGER.debug("handle node has changed, node: {}", node);
if (node != null && node.equals(mainModel.getSelectedNode())) {
LOGGER.debug("The node in the model has not changed.");
return;
}
List lightPorts = dmxSceneryModel.getLightPorts();
if (CollectionUtils.isNotEmpty(lightPorts)) {
for (DmxLightPort lightPort : lightPorts) {
lightPort.freeLightPort();
}
}
for (DmxScenery dmxScenery : dmxSceneryModel.getSceneries()) {
// check if the scenery is opened
String searchKey = DmxModelerView.prepareKey(dmxScenery);
LOGGER.info("Search for view with key: {}", searchKey);
Dockable dmxModelerViewDockable = desktop.getContext().getDockableByKey(searchKey);
if (dmxModelerViewDockable != null && dmxModelerViewDockable instanceof DmxModelerView) {
LOGGER.info("Close the DmxModelerView: {}", dmxModelerViewDockable);
desktop.close(dmxModelerViewDockable);
}
}
// check if we must close the scenery panel
Dockable dmxSceneryDockable = desktop.getContext().getDockableByKey("DmxSceneryView");
if (dmxSceneryDockable != null) {
LOGGER.info("Close the DMX scenery panel: {}", dmxSceneryDockable);
desktop.close(dmxSceneryDockable);
}
// unregister node list listener
mainModel.removeNodeListListener(this);
}
@Override
public void nodeStateChanged() {
}
@Override
public void listNodeAdded(Node node) {
}
@Override
public void listNodeRemoved(Node node) {
}
@Override
public void portConfigChanged(Port> port) {
LOGGER.info("The port config has changed, port: {}", port);
try {
if (port instanceof LightPort) {
LightPort lightPort = (LightPort) port;
CommunicationFactory.getInstance().setLightPortParameters(mainModel.getSelectedNode().getNode(),
port.getId(), lightPort.getPwmMin(), lightPort.getPwmMax(), lightPort.getDimMin(),
lightPort.getDimMax(), lightPort.getRgbValue());
}
else if (port instanceof BacklightPort) {
BacklightPort backlightPort = (BacklightPort) port;
LOGGER.info("Current backlightPort: {}", backlightPort);
CommunicationFactory.getInstance().setBacklightPortParameters(mainModel.getSelectedNode().getNode(),
port.getId(), backlightPort.getDimSlopeDown(), backlightPort.getDimSlopeUp(),
backlightPort.getDmxMapping());
}
}
catch (Exception ex) {
LOGGER.warn("Set the port parameters failed.", ex);
// model.setNodeHasError(model.getSelectedNode(), true);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy