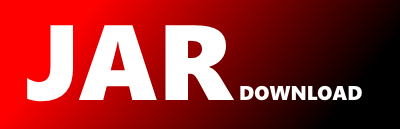
org.bidib.wizard.mvc.dmx.model.DmxChannel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.dmx.model;
import java.awt.Color;
import java.util.LinkedList;
import java.util.List;
import org.bidib.jbidibc.core.utils.CollectionUtils;
import org.bidib.jbidibc.exchange.dmxscenery.BacklightPortType;
import org.bidib.jbidibc.exchange.dmxscenery.DmxChannelType;
import org.bidib.jbidibc.exchange.dmxscenery.LightPortType;
import org.bidib.jbidibc.exchange.dmxscenery.LineColorUtils;
import org.bidib.jbidibc.exchange.dmxscenery.PortType;
import org.bidib.wizard.locale.Resources;
import org.bidib.wizard.mvc.main.model.BacklightPort;
import org.bidib.wizard.mvc.main.model.Port;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class DmxChannel extends Model {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = LoggerFactory.getLogger(DmxChannel.class);
public static final String PROPERTY_CHANNEL_ID = "channelId";
public static final String PROPERTY_INITIAL_STATE = "intialState";
public static final String PROPERTY_LINE_COLOR = "lineColor";
public static final String PROPERTY_ASSIGNED_PORTS = "assignedPorts";
private final int channelId;
private int initialState;
private Color lineColor/* = Color.GREEN */;
private List> assignedPorts = new LinkedList<>();
public DmxChannel(int channelId) {
this.channelId = channelId;
}
/**
* @return the channelId
*/
public int getChannelId() {
return channelId;
}
/**
* @return the initialState
*/
public int getInitialState() {
return initialState;
}
/**
* @param initialState
* the initialState to set
*/
public void setInitialState(int initialState) {
int oldValue = this.initialState;
this.initialState = initialState;
firePropertyChange(PROPERTY_INITIAL_STATE, oldValue, this.initialState);
}
/**
* @return the lineColor
*/
public Color getLineColor() {
return lineColor;
}
/**
* @param lineColor
* the lineColor to set
*/
public void setLineColor(Color lineColor) {
Color oldValue = this.lineColor;
this.lineColor = lineColor;
firePropertyChange(PROPERTY_LINE_COLOR, oldValue, this.lineColor);
}
public void addPort(Port> port) {
List> oldValue = this.assignedPorts;
// create new list because property change must fire
List> newAssignedPorts = new LinkedList<>(oldValue);
newAssignedPorts.add(port);
this.assignedPorts = newAssignedPorts;
firePropertyChange(PROPERTY_ASSIGNED_PORTS, oldValue, this.assignedPorts);
}
public List> getAssignedPorts() {
return assignedPorts;
}
/**
* @param assignedPorts
* the assigned ports to set
*/
public void setAssignedPorts(List> assignedPorts) {
List> oldValue = this.assignedPorts;
this.assignedPorts = assignedPorts;
firePropertyChange(PROPERTY_ASSIGNED_PORTS, oldValue, this.assignedPorts);
}
/**
* @param assignedPorts
* the assigned ports to set
*/
public void addAssignedPorts(List> assignedPorts) {
LOGGER.info("Add new assigned ports: {}", assignedPorts);
List> oldValue = this.assignedPorts;
// create new list because property change must fire
List> newAssignedPorts = new LinkedList<>(oldValue);
newAssignedPorts.addAll(assignedPorts);
this.assignedPorts = newAssignedPorts;
firePropertyChange(PROPERTY_ASSIGNED_PORTS, oldValue, this.assignedPorts);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof DmxChannel) {
return (channelId == ((DmxChannel) obj).getChannelId());
}
return false;
}
@Override
public int hashCode() {
return channelId;
}
public String toString() {
String result = null;
if (channelId != -1) {
result = Resources.getString(getClass(), "label") + " " + channelId;
}
else {
result = "";
}
return result;
}
public DmxChannel withDmxChannel(DmxEnvironmentProvider dmxEnvironmentProvider, DmxChannelType dmxChannelType) {
setLineColor(LineColorUtils.getColor(dmxChannelType.getLineColor()));
// TODO check if the DMX target channel of all imported ports are configured correct
int dmxTargetChannel = dmxChannelType.getChannelNumber();
// add the ports
if (CollectionUtils.hasElements(dmxChannelType.getPort())) {
List> ports = new LinkedList<>();
for (PortType portType : dmxChannelType.getPort()) {
LOGGER.info("Current port: {}", portType);
if (portType instanceof BacklightPortType) {
int portNum = portType.getPortNumber();
for (BacklightPort port : dmxEnvironmentProvider.getBacklightPorts()) {
if (port.getId() == portNum) {
// found matching port
ports.add(port);
break;
}
}
}
else if (portType instanceof LightPortType) {
int portNum = portType.getPortNumber();
for (DmxLightPort port : dmxEnvironmentProvider.getLightPorts()) {
if (port.getId() == portNum) {
// found matching port
ports.add(port);
break;
}
}
}
else {
LOGGER.warn("Unsupported port type detected: {}", portType);
}
}
// TODO if we have autoAdd of ports enabled all ports that are mapped to the DMX channel are added
// automatically
boolean autoAddPorts = false;
if (autoAddPorts) {
// check if the OneDMX has more ports assigned to the current channel
for (DmxLightPort dmxLightPort : dmxEnvironmentProvider.getLightPorts()) {
// check if the DMX target channel matches the id of the current DMX channel
if (dmxLightPort.getDmxTargetChannel() != null
&& dmxLightPort.getDmxTargetChannel().getChannelId() == dmxTargetChannel) {
// check if we must assign this port
if (!ports.contains(dmxLightPort)) {
LOGGER.info("Add new dmxLightPort that is configured in the OneDMX: {}", dmxLightPort);
ports.add(dmxLightPort);
}
}
}
for (BacklightPort backlightPort : dmxEnvironmentProvider.getBacklightPorts()) {
// check if the DMX target channel matches the id of the current DMX channel
if (backlightPort.getDmxMapping() == dmxTargetChannel) {
// check if we must assign this port
if (!ports.contains(backlightPort)) {
LOGGER.info("Add new backlightPort that is configured in the OneDMX: {}", backlightPort);
ports.add(backlightPort);
}
}
}
}
LOGGER.info("Set the assigned ports: {}", ports);
setAssignedPorts(ports);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy