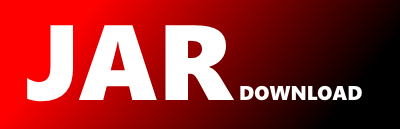
org.bidib.wizard.mvc.dmx.model.DmxScenery Maven / Gradle / Ivy
package org.bidib.wizard.mvc.dmx.model;
import java.util.LinkedList;
import java.util.List;
import org.bidib.jbidibc.core.utils.ByteUtils;
import org.bidib.jbidibc.core.utils.CollectionUtils;
import org.bidib.jbidibc.exchange.dmxscenery.BacklightPortType;
import org.bidib.jbidibc.exchange.dmxscenery.DmxChannelType;
import org.bidib.jbidibc.exchange.dmxscenery.DmxSceneryPointType;
import org.bidib.jbidibc.exchange.dmxscenery.DmxSceneryPointsType;
import org.bidib.jbidibc.exchange.dmxscenery.DmxSceneryType;
import org.bidib.jbidibc.exchange.dmxscenery.LightPortType;
import org.bidib.jbidibc.exchange.dmxscenery.LineColorUtils;
import org.bidib.jbidibc.exchange.dmxscenery.PortType;
import org.bidib.wizard.mvc.main.model.BacklightPort;
import org.bidib.wizard.mvc.main.model.LightPort;
import org.bidib.wizard.mvc.main.model.Port;
import org.bidib.wizard.mvc.main.model.function.MacroFunction;
import org.bidib.wizard.utils.PortUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
/**
*
* The DmxScenery
holds the data of a single scenery. The single scenery contains points of one or more DMX
* channels.
*/
public class DmxScenery extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(DmxScenery.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTY_NAME = "name";
public static final String PROPERTY_MACRO_NUMBER = "macroNumber";
public static final String PROPERTY_DIMM_STRETCH = "dimmStretch";
public static final String PROPERTY_USED_CHANNELS = "usedChannels";
private final String id;
private String name;
private List usedChannels = new LinkedList<>();
private List sceneryPoints = new LinkedList<>();
private Integer macroNumber;
/**
* Creates a new instance of DmxScenery with the provided scenery id.
*
* @param id
* the scenery id
*/
public DmxScenery(final String id) {
this.id = id;
}
/**
* @return the scenery id
*/
public String getId() {
return id;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name
* the name to set
*/
public void setName(String name) {
String oldValue = this.name;
this.name = name;
firePropertyChange(PROPERTY_NAME, oldValue, name);
}
/**
* @return the usedChannels
*/
public List getUsedChannels() {
return usedChannels;
}
/**
* @param usedChannels
* the usedChannels to set
*/
public void setUsedChannels(List usedChannels) {
List oldValue = this.usedChannels;
// assign new list of channels
this.usedChannels = usedChannels;
firePropertyChange(PROPERTY_USED_CHANNELS, oldValue, this.usedChannels);
}
/**
* @param usedChannels
* the usedChannels to add
*/
public void addUsedChannels(List usedChannels) {
List oldValue = this.usedChannels;
// create new list because property change must fire
List newUsedChannels = new LinkedList<>(oldValue);
newUsedChannels.addAll(usedChannels);
this.usedChannels = newUsedChannels;
firePropertyChange(PROPERTY_USED_CHANNELS, oldValue, this.usedChannels);
}
/**
* @param usedChannel
* the usedChannel to add
*/
public void addUsedChannel(DmxChannel usedChannel) {
List oldValue = this.usedChannels;
// create new list because property change must fire
List newUsedChannels = new LinkedList<>(oldValue);
newUsedChannels.add(usedChannel);
this.usedChannels = newUsedChannels;
firePropertyChange(PROPERTY_USED_CHANNELS, oldValue, this.usedChannels);
}
/**
* @param usedChannel
* the usedChannel to remove
*/
public void removeUsedChannel(DmxChannel usedChannel) {
List oldValue = this.usedChannels;
// create new list because property change must fire
List newUsedChannels = new LinkedList<>(oldValue);
newUsedChannels.remove(usedChannel);
this.usedChannels = newUsedChannels;
firePropertyChange(PROPERTY_USED_CHANNELS, oldValue, this.usedChannels);
}
/**
* @return the sceneryPoints
*/
public List getSceneryPoints() {
return sceneryPoints;
}
/**
* @param sceneryPoints
* the sceneryPoints to set
*/
public void setSceneryPoints(List sceneryPoints) {
this.sceneryPoints = sceneryPoints;
}
/**
* @return the macroNumber
*/
public Integer getMacroNumber() {
return macroNumber;
}
/**
* @param macroNumber
* the macroNumber to set
*/
public void setMacroNumber(Integer macroNumber) {
Integer oldValue = this.macroNumber;
this.macroNumber = macroNumber;
firePropertyChange(PROPERTY_MACRO_NUMBER, oldValue, this.macroNumber);
}
// /**
// * @return the dimmStretch
// */
// public Integer getDimmStretch() {
// return dimmStretch;
// }
//
// /**
// * @param dimmStretch
// * the dimmStretch to set
// */
// public void setDimmStretch(Integer dimmStretch) {
// Integer oldValue = this.dimmStretch;
//
// this.dimmStretch = dimmStretch;
//
// firePropertyChange(PROPERTY_DIMM_STRETCH, oldValue, this.dimmStretch);
// }
@Override
public String toString() {
return name;
}
public DmxScenery withDmxScenery(DmxEnvironmentProvider dmxEnvironmentProvider, DmxSceneryType dmxScenery) {
setName(dmxScenery.getSceneryName());
setMacroNumber(dmxScenery.getMacroNumber());
if (CollectionUtils.hasElements(dmxScenery.getDmxChannel())) {
List dmxChannels = new LinkedList<>();
for (DmxChannelType dmxChannelType : dmxScenery.getDmxChannel()) {
// check if the DMX channel is available
int channelNumber = dmxChannelType.getChannelNumber();
int index = dmxEnvironmentProvider.getDmxChannels().indexOf(new DmxChannel(channelNumber));
if (index > -1) {
DmxChannel dmxChannel = dmxEnvironmentProvider.getDmxChannels().get(index);
// TODO we must reconfigure the OneDMX if a port is not assigned to a channel
// configure the assigned channels
dmxChannel.withDmxChannel(dmxEnvironmentProvider, dmxChannelType);
dmxChannels.add(dmxChannel);
}
else {
LOGGER.warn("No DMX channel found in system with channel number: {}", channelNumber);
}
}
// set the channels
addUsedChannels(dmxChannels);
}
if (dmxScenery.getDmxSceneryPoints() != null) {
// handle the scenery points
for (DmxSceneryPointType dmxSceneryPointType : dmxScenery.getDmxSceneryPoints().getDmxSceneryPoint()) {
DmxSceneryPoint dmxSceneryPoint =
new DmxSceneryPoint()
.withTimeOffset(dmxSceneryPointType.getTimeOffset())
.withBrightness(dmxSceneryPointType.getBrightness())
.withUniqueId(dmxSceneryPointType.getUniqueId())
.withDmxChannelNumber(dmxSceneryPointType.getDmxChannelNumber());
// set the macro number
if (dmxSceneryPointType.getMacroNumber() != null) {
MacroFunction macro = new MacroFunction();
macro.setMacroId(dmxSceneryPointType.getMacroNumber());
dmxSceneryPoint.setMacro(macro);
}
// set the port and action
if (dmxSceneryPointType.getPort() != null) {
dmxSceneryPoint.withPort(fromPortType(dmxEnvironmentProvider, dmxSceneryPointType.getPort()));
dmxSceneryPoint.withAction(PortUtils.actionFromPortType(dmxSceneryPointType.getPort(),
dmxSceneryPointType.getBidibAction()));
}
sceneryPoints.add(dmxSceneryPoint);
}
}
return this;
}
private Port> fromPortType(DmxEnvironmentProvider dmxEnvironmentProvider, PortType portType) {
if (portType != null) {
int portNumber = portType.getPortNumber();
// search the ports ...
if (portType instanceof LightPortType) {
// return get the lightport
for (DmxLightPort lightPort : dmxEnvironmentProvider.getLightPorts()) {
if (lightPort.getId() == portNumber) {
return lightPort;
}
}
}
else if (portType instanceof BacklightPortType) {
// return get the backlightport
for (BacklightPort backlightPort : dmxEnvironmentProvider.getBacklightPorts()) {
if (backlightPort.getId() == portNumber) {
return backlightPort;
}
}
}
}
LOGGER.warn("No port found for portType: {}", portType);
return null;
}
private PortType fromPort(Port> port) {
PortType portType = null;
if (port instanceof DmxLightPort) {
LightPort lightPort = ((DmxLightPort) port).getLightPort();
portType = new LightPortType().withPortNumber(lightPort.getId());
}
else if (port instanceof BacklightPort) {
portType = new BacklightPortType().withPortNumber(port.getId());
}
else {
LOGGER.warn("Unsupported port detected: {}", port);
}
return portType;
}
public DmxSceneryType fromDmxScenery() {
DmxSceneryType dmxSceneryType =
new DmxSceneryType().withSceneryName(getName()).withMacroNumber(getMacroNumber());
// store the channels
for (DmxChannel dmxChannel : getUsedChannels()) {
DmxChannelType dmxChannelType =
new DmxChannelType()
.withChannelName(dmxChannel.toString()).withChannelNumber(dmxChannel.getChannelId())
.withLineColor(LineColorUtils.getColorType(dmxChannel.getLineColor()));
// add the ports
for (Port> port : dmxChannel.getAssignedPorts()) {
PortType portType = fromPort(port);
if (portType != null) {
dmxChannelType.getPort().add(portType);
}
}
dmxSceneryType.getDmxChannel().add(dmxChannelType);
}
if (CollectionUtils.hasElements(sceneryPoints)) {
DmxSceneryPointsType dmxSceneryPoints = new DmxSceneryPointsType();
for (DmxSceneryPoint dmxSceneryPoint : sceneryPoints) {
// check if the port is available
PortType portType = fromPort(dmxSceneryPoint.getPort());
DmxSceneryPointType dmxSceneryPointType =
new DmxSceneryPointType()
.withTimeOffset(dmxSceneryPoint.getTimeOffset())
.withDmxChannelNumber(dmxSceneryPoint.getDmxChannelNumber())
.withBrightness(dmxSceneryPoint.getBrightness()).withUniqueId(dmxSceneryPoint.getUniqueId());
if (portType != null) {
dmxSceneryPointType.withPort(portType);
if (dmxSceneryPoint.getAction() != null) {
dmxSceneryPointType.withBidibAction(ByteUtils.getInt(dmxSceneryPoint
.getAction().getType().getType()));
}
}
else if (dmxSceneryPoint.getMacro() != null) {
dmxSceneryPointType.withMacroNumber(dmxSceneryPoint.getMacro().getMacroId());
}
else {
LOGGER.warn("Incomplete scenery point without port assigned is stored.");
}
dmxSceneryPoints.getDmxSceneryPoint().add(dmxSceneryPointType);
}
dmxSceneryType.setDmxSceneryPoints(dmxSceneryPoints);
}
return dmxSceneryType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy