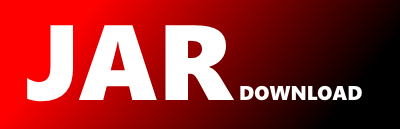
org.bidib.wizard.mvc.firmware.model.FirmwareUpdateModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.firmware.model;
import java.util.List;
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class FirmwareUpdateModel extends Model {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = LoggerFactory.getLogger(FirmwareUpdateModel.class);
public static final String PROPERTYNAME_NODE_NAME = "nodeName";
public static final String PROPERTYNAME_UUID = "uuid";
public static final String PROPERTYNAME_INSTALLED_VERSION = "installedVersion";
public static final String PROPERTYNAME_SELECTED_ARCHIVE = "firmwareArchivePath";
public static final String PROPERTYNAME_SELECTED_ARCHIVE_NAME = "firmwareArchiveName";
public static final String PROPERTYNAME_SELECTED_UPDATES = "selectedUpdates";
public static final String PROPERTYNAME_EXPERT_MODE = "expertMode";
public static final String PROPERTYNAME_CAN_UPDATE = "canUpdate";
public static final String PROPERTYNAME_NODE_LOST = "nodeLost";
private String nodeName;
private String uuid;
private String installedVersion;
private String firmwareArchivePath;
private String firmwareArchiveName;
private List selectedUpdates;
private boolean expertMode;
private boolean firmwareUpdateInProgress;
private boolean firmwareFilesSelected;
private boolean nodeLost;
/**
* @return the firmwareUpdateInProgress
*/
public boolean isFirmwareUpdateInProgress() {
return firmwareUpdateInProgress;
}
/**
* @param firmwareUpdateInProgress
* the firmwareUpdateInProgress to set
*/
public void setFirmwareUpdateInProgress(boolean firmwareUpdateInProgress) {
boolean oldCanUpdate = isCanUpdate();
LOGGER.info("Set the new firmwareUpdateInProgress value: {}, old: {} ", firmwareUpdateInProgress,
this.firmwareUpdateInProgress);
this.firmwareUpdateInProgress = firmwareUpdateInProgress;
fireCanUpdate(oldCanUpdate);
}
/**
* @return the firmwareFilesSelected
*/
public boolean isFirmwareFilesSelected() {
return firmwareFilesSelected;
}
/**
* @param firmwareFilesSelected
* the firmwareFilesSelected to set
*/
public void setFirmwareFilesSelected(boolean firmwareFilesSelected) {
boolean oldCanUpdate = isCanUpdate();
this.firmwareFilesSelected = firmwareFilesSelected;
fireCanUpdate(oldCanUpdate);
}
private void fireCanUpdate(boolean oldValue) {
LOGGER.info("fire canUpdate, oldValue: {}", oldValue);
firePropertyChange(PROPERTYNAME_CAN_UPDATE, oldValue, isCanUpdate());
}
/**
* @return the firmware update process can be started
*/
public boolean isCanUpdate() {
boolean isCanUpdate =
StringUtils.isNotBlank(firmwareArchiveName) && !firmwareUpdateInProgress && firmwareFilesSelected
&& !nodeLost;
LOGGER.info("Current isCanUpdate: {}", isCanUpdate);
return isCanUpdate;
}
/**
* @return the nodeName
*/
public String getNodeName() {
return nodeName;
}
/**
* @param nodeName
* the nodeName to set
*/
public void setNodeName(String nodeName) {
this.nodeName = nodeName;
String oldValue = this.nodeName;
this.nodeName = nodeName;
firePropertyChange(PROPERTYNAME_NODE_NAME, oldValue, nodeName);
}
/**
* @return the uuid
*/
public String getUuid() {
return uuid;
}
/**
* @param uuid
* the uuid to set
*/
public void setUuid(String uuid) {
String oldValue = this.uuid;
this.uuid = uuid;
firePropertyChange(PROPERTYNAME_UUID, oldValue, uuid);
}
/**
* @return the installedVersion
*/
public String getInstalledVersion() {
return installedVersion;
}
/**
* @param installedVersion
* the installedVersion to set
*/
public void setInstalledVersion(String installedVersion) {
String oldValue = this.installedVersion;
this.installedVersion = installedVersion;
firePropertyChange(PROPERTYNAME_INSTALLED_VERSION, oldValue, installedVersion);
}
/**
* @return the firmwareArchive
*/
public String getFirmwareArchivePath() {
return firmwareArchivePath;
}
/**
* @param firmwareArchivePath
* the firmwareArchive to set
*/
public void setFirmwareArchivePath(String firmwareArchivePath) {
String oldValue = this.firmwareArchivePath;
this.firmwareArchivePath = firmwareArchivePath;
firePropertyChange(PROPERTYNAME_SELECTED_ARCHIVE, oldValue, firmwareArchivePath);
// update the name
setFirmwareArchiveName(FilenameUtils.getName(firmwareArchivePath));
}
/**
* @return the firmwareArchiveName
*/
public String getFirmwareArchiveName() {
return firmwareArchiveName;
}
/**
* @param firmwareArchiveName
* the firmwareArchiveName to set
*/
public void setFirmwareArchiveName(String firmwareArchiveName) {
boolean oldCanUpdate = isCanUpdate();
String oldValue = this.firmwareArchiveName;
this.firmwareArchiveName = firmwareArchiveName;
firePropertyChange(PROPERTYNAME_SELECTED_ARCHIVE_NAME, oldValue, firmwareArchiveName);
fireCanUpdate(oldCanUpdate);
}
/**
* @return the selectedUpdates
*/
public List getSelectedUpdates() {
return selectedUpdates;
}
/**
* @param selectedUpdates
* the selectedUpdates to set
*/
public void setSelectedUpdates(List selectedUpdates) {
boolean oldCanUpdate = isCanUpdate();
List oldValue = this.selectedUpdates;
this.selectedUpdates = selectedUpdates;
firePropertyChange(PROPERTYNAME_SELECTED_UPDATES, oldValue, selectedUpdates);
fireCanUpdate(oldCanUpdate);
}
/**
* @return the expertMode
*/
public boolean isExpertMode() {
return expertMode;
}
/**
* @param expertMode
* the expertMode to set
*/
public void setExpertMode(boolean expertMode) {
boolean oldValue = this.expertMode;
this.expertMode = expertMode;
firePropertyChange(PROPERTYNAME_EXPERT_MODE, oldValue, expertMode);
}
/**
* @return the nodeLost
*/
public boolean isNodeLost() {
return nodeLost;
}
/**
* @param nodeLost
* the nodeLost to set
*/
public void setNodeLost(boolean nodeLost) {
boolean oldCanUpdate = isCanUpdate();
boolean oldValue = this.nodeLost;
this.nodeLost = nodeLost;
firePropertyChange(PROPERTYNAME_NODE_LOST, oldValue, selectedUpdates);
fireCanUpdate(oldCanUpdate);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy