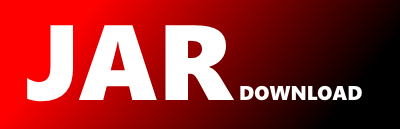
org.bidib.wizard.mvc.loco.controller.LocoController Maven / Gradle / Ivy
package org.bidib.wizard.mvc.loco.controller;
import java.util.BitSet;
import javax.swing.JFrame;
import org.bidib.jbidibc.core.AddressData;
import org.bidib.jbidibc.core.DefaultMessageListener;
import org.bidib.jbidibc.core.DriveState;
import org.bidib.jbidibc.core.MessageListener;
import org.bidib.wizard.comm.Communication;
import org.bidib.wizard.comm.CommunicationFactory;
import org.bidib.wizard.comm.Direction;
import org.bidib.wizard.comm.SpeedSteps;
import org.bidib.wizard.comm.listener.CommunicationListener;
import org.bidib.wizard.mvc.common.view.ViewCloseListener;
import org.bidib.wizard.mvc.loco.model.LocoModel;
import org.bidib.wizard.mvc.loco.model.listener.LocoModelListener;
import org.bidib.wizard.mvc.loco.view.LocoView;
import org.bidib.wizard.mvc.main.model.Node;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LocoController {
private static final Logger LOGGER = LoggerFactory.getLogger(LocoController.class);
private final Node node;
private final JFrame parent;
private final int x;
private final int y;
private final LocoModel model = new LocoModel();
private LocoView locoView;
private LocoModelListener locoModelListener;
public LocoController(Node node, JFrame parent, int x, int y) {
this.node = node;
this.parent = parent;
this.x = x;
this.y = y;
}
public void start() {
final Communication communication = CommunicationFactory.getInstance();
final MessageListener messageListener = new DefaultMessageListener() {
@Override
public void speed(byte[] address, AddressData addressData, int speed) {
if (addressData.getAddress() == model.getAddress()) {
model.setCurrentSpeed(speed);
}
}
@Override
public void csDriveManual(byte[] address, DriveState driveState) {
LOGGER.info("Received csDriveManual, address: {}, driveState: {}", address, driveState);
// TODO process csDriveManual message
}
};
communication.addMessageListener(messageListener);
communication.addCommunicationListener(new CommunicationListener() {
@Override
public void status(String statusText, int displayDuration) {
// TODO Auto-generated method stub
}
@Override
public void opened(String port) {
// TODO Auto-generated method stub
}
@Override
public void initialized() {
// TODO Auto-generated method stub
}
@Override
public void closed(String port) {
// TODO Auto-generated method stub
LOGGER.info("The communication port was closed, close the locoView: {}", locoView);
if (locoView != null) {
locoView.close();
locoView = null;
}
try {
communication.removeCommunicationListener(this);
}
catch (Exception ex) {
LOGGER.warn("Remove communication listener failed.", ex);
}
}
});
locoModelListener = new LocoModelListener() {
@Override
public void addressChanged(int address) {
}
@Override
public void currentSpeedChanged(int currentSpeed) {
}
@Override
public void directionChanged(Direction direction) {
}
@Override
public void functionChanged(int index, boolean value) {
// Prepare the function group index
int functionGroupIndex = 0;
if (index < 5) {
functionGroupIndex = 0;
}
else if (index < 9) {
functionGroupIndex = 1;
}
else if (index < 13) {
functionGroupIndex = 2;
}
else if (index < 21) {
functionGroupIndex = 3;
}
else {
functionGroupIndex = 4;
}
BitSet activeFunctions = new BitSet(functionGroupIndex + 1);
LOGGER.debug("functions have changed, functionGroupIndex: {}", functionGroupIndex);
activeFunctions.set(functionGroupIndex, true);
communication.setSpeed(node.getNode(), model.getAddress(), model.getSpeedSteps(), null,
model.getDirection(), activeFunctions, model.getFunctions());
}
@Override
public void speedChanged(int speed) {
communication.setSpeed(node.getNode(), model.getAddress(), model.getSpeedSteps(), speed,
model.getDirection(), null, null);
}
@Override
public void speedStepsChanged(SpeedSteps speedSteps) {
}
};
model.addLocoModelListener(locoModelListener);
LOGGER.info("Create new instance of LocoView.");
locoView = new LocoView(parent, model, x, y);
locoView.addViewCloseListener(new ViewCloseListener() {
@Override
public void close() {
LOGGER.info("The locoView is closed.");
unregisterMessageListener(messageListener, communication);
// release locoView
if (locoView != null) {
locoView = null;
}
if (locoModelListener != null) {
LOGGER.info("Remove locoModelListener from model.");
model.removeLocoModelListener(locoModelListener);
locoModelListener = null;
}
}
});
}
private void unregisterMessageListener(MessageListener messageListener, final Communication communication) {
LOGGER.info("Unregister message listener.");
if (messageListener != null) {
LOGGER.info("Remove the LocoController as message listener.");
try {
communication.removeMessageListener(messageListener);
}
catch (Exception ex) {
LOGGER.warn("Remove the LocoController as message listener failed.", ex);
}
messageListener = null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy