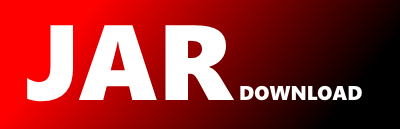
org.bidib.wizard.mvc.main.controller.DefaultNodeScripting Maven / Gradle / Ivy
package org.bidib.wizard.mvc.main.controller;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.bidib.jbidibc.core.node.ConfigurationVariable;
import org.bidib.jbidibc.core.port.PortConfigValue;
import org.bidib.wizard.comm.CommunicationFactory;
import org.bidib.wizard.main.DefaultApplicationContext;
import org.bidib.wizard.mvc.main.model.Accessory;
import org.bidib.wizard.mvc.main.model.Macro;
import org.bidib.wizard.mvc.main.model.MainModel;
import org.bidib.wizard.mvc.main.model.Node;
import org.bidib.wizard.mvc.script.view.NodeScripting;
import org.bidib.wizard.mvc.script.view.listener.NodeLabelScriptingListener;
import org.bidib.wizard.mvc.script.view.listener.NodeTreeScriptingListener;
import org.bidib.wizard.script.node.types.CvType;
import org.bidib.wizard.script.node.types.TargetType;
import org.bidib.wizard.utils.NodeUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DefaultNodeScripting implements NodeScripting {
private static final Logger LOGGER = LoggerFactory.getLogger(DefaultNodeScripting.class);
private final MainModel mainModel;
private final MainControllerInterface mainController;
public DefaultNodeScripting(MainModel mainModel, MainControllerInterface mainController) {
this.mainModel = mainModel;
this.mainController = mainController;
}
@Override
public void setLabel(Long uuid, TargetType portType) {
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Set CV can only be performed on the selected node!");
return;
}
NodeLabelScriptingListener tabPanel =
DefaultApplicationContext.getInstance().get("tabPanel", NodeLabelScriptingListener.class);
if (tabPanel != null) {
// Node selectedNode = mainModel.getSelectedNode();
// if (selectedNode != null && selectedNode.getUniqueId() == uuid.longValue()) {
LOGGER.info("Change the label on the selected node.");
tabPanel.setLabel(uuid, portType);
// }
}
}
@Override
public void setCv(Long uuid, CvType cvType) {
LOGGER.info("Set the CV, uuid: {}, cvType: {}", uuid, cvType);
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Set CV can only be performed on the selected node!");
return;
}
List cvList = new LinkedList<>();
cvList.add(new ConfigurationVariable(cvType.getCvNumber().toString(), cvType.getCvValue()));
mainModel.setConfigurationVariables(cvList);
// TODO transfer to node
List configVars =
CommunicationFactory.getInstance().writeConfigurationVariables(selectedNode.getNode(), cvList);
// iterate over the collection of stored variables in the model and update the values.
// After that notify the tree and delete the new values that are now stored in the node
mainModel.updateConfigurationVariableValues(configVars);
}
@Override
public void setMacro(Long uuid, Macro macro) {
LOGGER.info("Set the macro, uuid: {}, macro: {}", uuid, macro);
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Set macro can only be performed on the selected node!");
return;
}
mainController.replaceMacro(macro, true);
}
@Override
public void resetNode(Long uuid) {
LOGGER.info("Reset node with uuid: {}", uuid);
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Reset node can only be performed on the selected node!");
return;
}
// let the main controller reset the node
mainController.resetNode(selectedNode);
}
@Override
public void reselectNode(Long uuid) {
LOGGER.info("Reselect node with uuid: {}", uuid);
Node node = NodeUtils.findNodeByUuid(mainModel.getNodes(), uuid);
if (node != null) {
// select the node
LOGGER.info("Set the selected node in the mainModel: {}", node);
NodeTreeScriptingListener nodeListPanel =
DefaultApplicationContext.getInstance().get("nodeListPanel", NodeTreeScriptingListener.class);
if (nodeListPanel != null) {
try {
LOGGER.info("Change the selected node.");
nodeListPanel.setSelectedNode(node);
}
catch (IllegalArgumentException ex) {
LOGGER.warn("Select node failed.", ex);
throw ex;
}
}
}
}
@Override
public void setAccessory(Long uuid, Accessory accessory) {
LOGGER.info("Set the accessory, uuid: {}, accessory: {}", uuid, accessory);
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Set accessory can only be performed on the selected node!");
return;
}
// let the main controller replace the accessory
mainController.replaceAccessory(accessory, true);
}
@Override
public void setPortConfig(Long uuid, TargetType portType, final Map> portConfig) {
LOGGER.info("Set the port config, uuid: {}, portConfig: {}", uuid, portConfig);
Node selectedNode = mainModel.getSelectedNode();
if (selectedNode != null && selectedNode.getUniqueId() != uuid.longValue()) {
LOGGER.warn("Set port config can only be performed on the selected node!");
return;
}
// TODO support all port types
switch (portType.getScriptingTargetType()) {
case ANALOGPORT:
mainModel.setAnalogPortConfig(portType.getPortNum(), portConfig);
break;
case BACKLIGHTPORT:
mainModel.setBacklightPortConfig(portType.getPortNum(), portConfig);
break;
case LIGHTPORT:
mainModel.setLightPortConfig(portType.getPortNum(), portConfig);
break;
case SERVOPORT:
mainModel.setServoPortConfig(portType.getPortNum(), portConfig);
break;
case SWITCHPORT:
mainModel.setSwitchPortConfig(portType.getPortNum(), portConfig);
break;
default:
LOGGER.error("Unsupported port type detected: {}", portType);
break;
}
// write config to node
mainController.replacePortConfig(portType, portConfig);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy