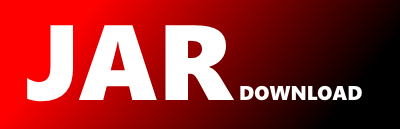
org.bidib.wizard.mvc.main.model.ChildLabels Maven / Gradle / Ivy
package org.bidib.wizard.mvc.main.model;
import java.util.HashMap;
import java.util.Map.Entry;
import org.apache.commons.collections4.MapUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public abstract class ChildLabels extends Labels {
private static final Logger LOGGER = LoggerFactory.getLogger(ChildLabels.class);
private class ChildNodeMap extends HashMap {
private static final long serialVersionUID = 1L;
}
public ChildLabels() {
labelMap = new ChildNodeMap();
}
public String getLabel(long uuid, int id) {
String result = null;
ChildLabelMap childLabelMap = labelMap.get(uuid);
if (childLabelMap != null) {
result = childLabelMap.get(id);
}
return result;
}
public HashMap getLabelMap(long uuid) {
HashMap result = null;
ChildLabelMap childLabelMap = labelMap.get(uuid);
if (childLabelMap != null) {
result = childLabelMap;
}
return result;
}
public void removeLabel(long uuid, int id) {
ChildLabelMap childLabelMap = labelMap.get(uuid);
if (childLabelMap != null) {
childLabelMap.remove(id);
modified = true;
}
}
public void setLabel(long uuid, int id, String value) {
LOGGER.info("Set the label, id: {}, value: {}", id, value);
ChildLabelMap childLabelMap = labelMap.get(uuid);
if (childLabelMap == null) {
childLabelMap = new ChildLabelMap();
labelMap.put(uuid, childLabelMap);
}
String oldValue = childLabelMap.put(id, value);
modified = true;
fireLabelsChanged(oldValue, value);
}
public Integer getIdByLabel(long uuid, String label) {
Integer id = null;
HashMap labelMap = getLabelMap(uuid);
if (MapUtils.isNotEmpty(labelMap)) {
for (Entry entry : labelMap.entrySet()) {
if (entry.getValue().equalsIgnoreCase(label)) {
// found corresponding entry
LOGGER.info("Found corresponding entry: {}", entry);
id = entry.getKey();
break;
}
}
}
return id;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy