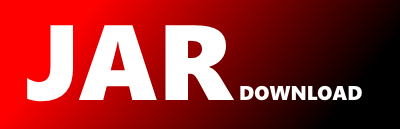
org.bidib.wizard.mvc.main.model.Labels Maven / Gradle / Ivy
package org.bidib.wizard.mvc.main.model;
import java.beans.XMLDecoder;
import java.beans.XMLEncoder;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.UnsupportedEncodingException;
import java.util.Map;
import org.bidib.jbidibc.core.exception.InvalidConfigurationException;
import org.bidib.wizard.mvc.preferences.model.Preferences;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.InputSource;
import com.jgoodies.binding.beans.Model;
public abstract class Labels extends Model {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = LoggerFactory.getLogger(Labels.class);
public static final String PROPERTY_LABELS = "labels";
protected Map labelMap;
/**
* Flag to signal if the data is modified.
*/
protected transient boolean modified = false;
protected void fireLabelsChanged(Object oldValue, Object newValue) {
firePropertyChange(PROPERTY_LABELS, oldValue, newValue);
}
private String getFileName() {
String result = null;
File baseDir = new File(Preferences.getInstance().getLabelPath());
if (!baseDir.exists()) {
LOGGER.info("Try to create new directory for labels.");
boolean created = baseDir.mkdirs();
if (!created) {
LOGGER.warn("The directory was not created!");
}
}
if (baseDir.exists()) {
result = new File(baseDir, getClass().getSimpleName()).toString();
}
return result;
}
public Map getLabelMap() {
return labelMap;
}
public void load() {
XMLDecoder d = null;
try {
String preparedFileName = getFileName();
LOGGER.info("Load labels to prepared filename: {}", preparedFileName);
d = new XMLDecoder(new InputSource(XmlLoader.changePackage(new FileReader(preparedFileName))));
Labels labels = (Labels) d.readObject();
if (labels != null) {
setLabelMap(labels.getLabelMap());
}
}
catch (FileNotFoundException e) {
LOGGER.trace("Read labels from file failed because the file is not available.", e);
}
catch (UnsupportedEncodingException e) {
LOGGER.warn("The selected encoding is not supported.", e);
}
catch (Exception ex) {
LOGGER.warn("Load labels from file failed.", ex);
}
finally {
if (d != null) {
d.close();
}
}
}
public void save() {
if (modified) {
try {
String preparedFileName = getFileName();
LOGGER.info("Save labels from prepared filename: {}", preparedFileName);
XMLEncoder e = new XMLEncoder(new BufferedOutputStream(new FileOutputStream(preparedFileName)));
e.writeObject(this);
e.close();
modified = false;
}
catch (Exception ex) {
LOGGER.warn("Save labels failed, filename: {}", getFileName(), ex);
InvalidConfigurationException ice =
new InvalidConfigurationException("Save labels failed, filename: " + getFileName());
ice.setReason(getFileName());
throw ice;
}
}
}
public void setLabelMap(Map labelMap) {
this.labelMap = labelMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy