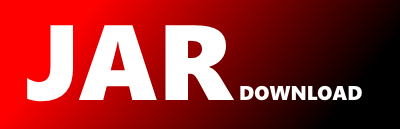
org.bidib.wizard.mvc.main.model.NodeState Maven / Gradle / Ivy
package org.bidib.wizard.mvc.main.model;
import java.beans.XMLDecoder;
import java.beans.XMLEncoder;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Collection;
import java.util.List;
import java.util.Vector;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
import org.bidib.jbidibc.core.Feature;
import org.bidib.jbidibc.core.StringData;
import org.bidib.jbidibc.core.node.ConfigurationVariable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This class is used to import and export the node.
*
*/
public class NodeState {
private static final Logger LOGGER = LoggerFactory.getLogger(NodeState.class);
private List accessories;
private List macros;
private List analogPorts;
private List backlightPorts;
private List feedbackPorts;
private List lightPorts;
private List servoPorts;
private List inputPorts;
private List switchPorts;
private List soundPorts;
private List motorPorts;
private List features;
private List configurationVariables;
private List nodeStrings;
public NodeState() {
}
public NodeState(Collection accessories, Collection analogPorts,
Collection backlightPorts, Collection feedbackPorts,
Collection lightPorts, Collection macros, Collection servoPorts,
Collection inputPorts, Collection switchPorts, Collection soundPorts,
Collection motorPorts, Collection features,
Collection configurationVariables, Collection nodeStrings) {
setMacros(macros);
setAccessories(accessories);
LOGGER.info("Number of lightports: {}", lightPorts.size());
setAnalogPorts(analogPorts);
setBacklightPorts(backlightPorts);
setFeedbackPorts(feedbackPorts);
setLightPorts(lightPorts);
setServoPorts(servoPorts);
setInputPorts(inputPorts);
setSoundPorts(soundPorts);
setSwitchPorts(switchPorts);
setMotorPorts(motorPorts);
setFeatures(features);
setConfigurationVariables(configurationVariables);
setNodeStrings(nodeStrings);
}
public Collection getAccessories() {
return accessories;
}
public void setAccessory(int index, Accessory accessory) {
accessories.set(index, accessory);
}
public void setAccessories(Collection accessories) {
this.accessories = new Vector(accessories);
}
/**
* @return the analog ports
*/
public Collection getAnalogPorts() {
return analogPorts;
}
public void setAnalogPort(int index, AnalogPort analogPort) {
analogPorts.set(index, analogPort);
}
public void setAnalogPorts(Collection analogPorts) {
this.analogPorts = new Vector(analogPorts);
}
/**
* @return the backlight ports
*/
public Collection getBacklightPorts() {
return backlightPorts;
}
public void setBacklightPort(int index, BacklightPort backlightPort) {
backlightPorts.set(index, backlightPort);
}
public void setBacklightPorts(Collection backlightPorts) {
this.backlightPorts = new Vector(backlightPorts);
}
/**
* @return the feedback ports
*/
public Collection getFeedbackPorts() {
return feedbackPorts;
}
public void setFeedbackPort(int index, FeedbackPort feedbackPort) {
feedbackPorts.set(index, feedbackPort);
}
public void setFeedbackPorts(Collection feedbackPorts) {
this.feedbackPorts = new Vector(feedbackPorts);
}
/**
* @return the light ports
*/
public Collection getLightPorts() {
return lightPorts;
}
// public void setLightPort(int index, LightPort lightPort) {
// lightPorts.set(index, lightPort);
// }
public void setLightPorts(Collection lightPorts) {
this.lightPorts = new Vector(lightPorts);
}
public Collection getMacros() {
return macros;
}
public void setMacro(int index, Macro macro) {
macros.set(index, macro);
}
public void setMacros(Collection macros) {
this.macros = new Vector(macros);
}
public Collection getServoPorts() {
return servoPorts;
}
public void setServoPort(int index, ServoPort servoPort) {
servoPorts.set(index, servoPort);
}
public void setServoPorts(Collection servoPorts) {
this.servoPorts = new Vector(servoPorts);
}
/**
* @return the inputPorts
*/
public Collection getInputPorts() {
return inputPorts;
}
public void setInputPort(int index, InputPort inputPort) {
inputPorts.set(index, inputPort);
}
/**
* @param inputPorts
* the inputPorts to set
*/
public void setInputPorts(Collection inputPorts) {
this.inputPorts = new Vector(inputPorts);
}
/**
* @return the soundPorts
*/
public Collection getSoundPorts() {
return soundPorts;
}
/**
* @param soundPorts
* the soundPorts to set
*/
public void setSoundPorts(Collection soundPorts) {
this.soundPorts = new Vector(soundPorts);
}
public void setSoundPort(int index, SoundPort soundPort) {
soundPorts.set(index, soundPort);
}
/**
* @return the switchPorts
*/
public Collection getSwitchPorts() {
return switchPorts;
}
public void setSwitchPort(int index, SwitchPort switchPort) {
switchPorts.set(index, switchPort);
}
/**
* @param switchPorts
* the switchPorts to set
*/
public void setSwitchPorts(Collection switchPorts) {
this.switchPorts = new Vector(switchPorts);
}
/**
* @return the motorPorts
*/
public Collection getMotorPorts() {
return motorPorts;
}
public void setMotorPort(int index, MotorPort motorPort) {
motorPorts.set(index, motorPort);
}
/**
* @param motorPorts
* the motorPorts to set
*/
public void setMotorPorts(Collection motorPorts) {
this.motorPorts = new Vector(motorPorts);
}
/**
* @return the features
*/
public Collection getFeatures() {
return features;
}
/**
* @param features
* the features to set
*/
public void setFeatures(Collection features) {
this.features = new Vector(features);
}
/**
* @return the configurationVariables
*/
public Collection getConfigurationVariables() {
return configurationVariables;
}
/**
* @param configurationVariables
* the configurationVariables to set
*/
public void setConfigurationVariables(Collection configurationVariables) {
this.configurationVariables = new Vector(configurationVariables);
}
/**
* @return the nodeStrings
*/
public Collection getNodeStrings() {
return nodeStrings;
}
/**
* @param nodeStrings
* the nodeStrings to set
*/
public void setNodeStrings(Collection nodeStrings) {
this.nodeStrings = new Vector(nodeStrings);
}
public static NodeState load(String fileName) {
NodeState result = null;
XMLDecoder d = null;
try {
try {
d = new XMLDecoder(new GZIPInputStream(new BufferedInputStream(new FileInputStream(fileName))));
result = (NodeState) d.readObject();
}
catch (IOException e) {
LOGGER.warn("Load zipped node file failed. Try unzipped.", e);
d = new XMLDecoder(new BufferedInputStream(new FileInputStream(fileName)));
result = (NodeState) d.readObject();
}
}
catch (FileNotFoundException e) {
LOGGER.warn("Load node file failed.", e);
}
finally {
if (d != null) {
d.close();
}
}
return result;
}
/**
* Save the configuration of the node.
*
* @param fileName
* the filename
* @throws IOException
*/
public void save(String fileName) throws IOException {
XMLEncoder e = new XMLEncoder(new GZIPOutputStream(new BufferedOutputStream(new FileOutputStream(fileName))));
e.writeObject(this);
e.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy