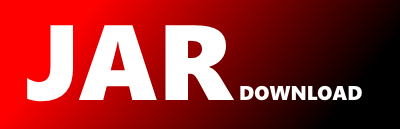
org.bidib.wizard.mvc.ping.model.NodePingModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.ping.model;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import org.apache.commons.lang.StringUtils;
import org.bidib.jbidibc.core.Node;
import org.bidib.jbidibc.core.StringData;
import org.bidib.wizard.mvc.main.model.NodeLabels;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class NodePingModel extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(NodePingModel.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTY_NODE_PING_STATUS = "nodePingStatus";
public static final String PROPERTY_PING_INTERVAL = "pingInterval";
public static final String PROPERTY_LAST_PING = "lastPing";
private final Node node;
private NodeLabels nodeLabels;
private String nodeLabel;
private NodePingState nodePingState;
private Integer pingInterval;
private long lastPing;
private PropertyChangeListener propertyChangeListener;
public NodePingModel(final Node node, final NodeLabels nodeLabels) {
this.node = node;
this.nodeLabels = nodeLabels;
LOGGER.info("Create new NodePingModel for node: {}", node);
}
public void registerNode() {
propertyChangeListener = new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
// update the label with the name ...
LOGGER.debug("Property has changed, name: {}, value: {}", evt.getPropertyName(), evt.getNewValue());
String nodeLabel = prepareNodeLabel();
setNodeLabel(nodeLabel);
}
};
this.node.addPropertyChangeListener(propertyChangeListener);
}
public void freeNode() {
this.node.removePropertyChangeListener(propertyChangeListener);
}
/**
* @return the node
*/
public Node getNode() {
return node;
}
/**
* @return the nodePingState
*/
public NodePingState getNodePingState() {
return nodePingState;
}
/**
* @param nodePingState
* the nodePingState to set
*/
public void setNodePingState(NodePingState nodePingState) {
NodePingState oldValue = this.nodePingState;
this.nodePingState = nodePingState;
firePropertyChange(PROPERTY_NODE_PING_STATUS, oldValue, this.nodePingState);
}
/**
* @param nodeLabel
* the node label to set
*/
public void setNodeLabel(String nodeLabel) {
this.nodeLabel = nodeLabel;
}
/**
* @return the node label
*/
public String getNodeLabel() {
return nodeLabel;
}
/**
* @return the pingInterval in seconds
*/
public Integer getPingInterval() {
return pingInterval;
}
/**
* @param pingInterval
* the pingInterval in seconds to set
*/
public void setPingInterval(Integer pingInterval) {
Integer oldValue = this.pingInterval;
this.pingInterval = pingInterval;
firePropertyChange(PROPERTY_PING_INTERVAL, oldValue, this.pingInterval);
}
/**
* @return the lastPing
*/
public long getLastPing() {
return lastPing;
}
/**
* @param lastPing
* the lastPing to set
*/
public void setLastPing(long lastPing) {
long oldValue = this.lastPing;
this.lastPing = lastPing;
firePropertyChange(PROPERTY_LAST_PING, oldValue, this.lastPing);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof NodePingModel) {
NodePingModel other = (NodePingModel) obj;
if (node.equals(other.getNode())) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
return node.hashCode();
}
public String prepareNodeLabel() {
String nodeLabel = nodeLabels.getLabel(node.getUniqueId());
if (StringUtils.isBlank(nodeLabel)) {
// try to get the product name
String productString = node.getStoredString(StringData.INDEX_PRODUCTNAME);
if (StringUtils.isNotBlank(productString)) {
nodeLabel = productString;
}
}
return nodeLabel;
}
@Override
public String toString() {
return node.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy