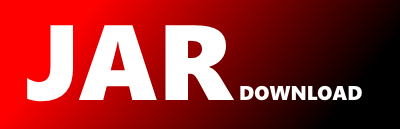
org.bidib.wizard.mvc.railcomplus.model.RailcomPlusDecoderModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.railcomplus.model;
import java.util.Objects;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class RailcomPlusDecoderModel extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(RailcomPlusDecoderModel.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTY_DECODER_MANUFACTURER = "decoderManufacturer";
public static final String PROPERTY_DECODER_ADDRESS = "decoderAddress";
private Integer decoderManufacturer;
private Integer decoderAddress;
private Integer decoderMun;
public RailcomPlusDecoderModel() {
}
/**
* @return the decoderManufacturer
*/
public Integer getDecoderManufacturer() {
return decoderManufacturer;
}
/**
* @param decoderManufacturer
* the decoderManufacturer to set
*/
public void setDecoderManufacturer(Integer decoderManufacturer) {
LOGGER.info("Set the decoder manufacturer: {}", decoderManufacturer);
this.decoderManufacturer = decoderManufacturer;
}
/**
* @return the decoderAddress
*/
public Integer getDecoderAddress() {
return decoderAddress;
}
/**
* @param decoderAddress
* the decoderAddress to set
*/
public void setDecoderAddress(Integer decoderAddress) {
LOGGER.info("Set the decoder address: {}", decoderAddress);
this.decoderAddress = decoderAddress;
}
/**
* @return the decoderMun
*/
public Integer getDecoderMun() {
return decoderMun;
}
/**
* @param decoderMun
* the decoderMun to set
*/
public void setDecoderMun(Integer decoderMun) {
this.decoderMun = decoderMun;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof RailcomPlusDecoderModel) {
RailcomPlusDecoderModel other = (RailcomPlusDecoderModel) obj;
if (Objects.equals(decoderManufacturer, other.decoderManufacturer)
&& Objects.equals(decoderMun, other.decoderMun) /*
* && Objects.equals(decoderAddress,
* other.decoderAddress)
*/) {
return true;
}
}
return false;
}
@Override
public int hashCode() {
int hashCode = super.hashCode();
if (decoderManufacturer != null) {
hashCode += decoderManufacturer.hashCode();
}
if (decoderMun != null) {
hashCode += decoderMun.hashCode();
}
/*
* if (decoderAddress != null) { hashCode += decoderAddress.hashCode(); }
*/
return hashCode;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy