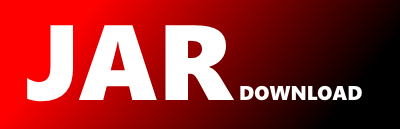
org.bidib.wizard.mvc.railcomplus.model.RailcomPlusModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.railcomplus.model;
import java.util.LinkedList;
import java.util.List;
import org.bidib.jbidibc.core.TidData;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
import com.jgoodies.common.collect.ArrayListModel;
public class RailcomPlusModel extends Model {
private static final long serialVersionUID = 1L;
private static final Logger LOGGER = LoggerFactory.getLogger(RailcomPlusModel.class);
public static final String PROPERTY_DECODERS = "decoders";
public static final String PROPERTY_TID = "tid";
private TidData tid;
private ArrayListModel decoderList = new ArrayListModel<>();
public RailcomPlusModel() {
}
/**
* @return the decoderList model
*/
public ArrayListModel getDecoderListModel() {
return decoderList;
}
/**
* @return the tid
*/
public TidData getTid() {
return tid;
}
/**
* @param tid
* the tid to set
*/
public void setTid(TidData tid) {
LOGGER.info("Set the TID: {}", tid);
TidData oldValue = this.tid;
this.tid = tid;
firePropertyChange(PROPERTY_TID, oldValue, this.tid);
}
public void addDecoder(RailcomPlusDecoderModel decoder) {
synchronized (decoderList) {
if (!decoderList.contains(decoder)) {
LOGGER.info("Add decoder to decoder list: {}", decoder);
List oldValue = new LinkedList<>(decoderList);
decoderList.add(decoder);
firePropertyChange(PROPERTY_DECODERS, oldValue, decoderList);
}
else {
LOGGER.warn("Decoder is already in decoder list: {}", decoder);
}
}
}
public void removeDecoder(RailcomPlusDecoderModel decoder) {
synchronized (decoderList) {
LOGGER.info("Remove decoder from decoder list: {}", decoder);
List oldValue = new LinkedList<>(decoderList);
boolean removed = decoderList.remove(decoder);
if (removed) {
firePropertyChange(PROPERTY_DECODERS, oldValue, decoderList);
}
else {
LOGGER.warn("Decoder was not removed from decoder list: {}", decoder);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy