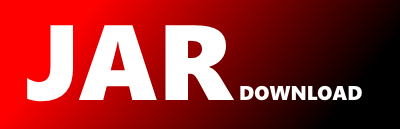
org.bidib.wizard.mvc.script.model.NodeScriptModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.script.model;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.Map;
import org.bidib.wizard.mvc.main.model.Node;
import org.bidib.wizard.script.node.NodeScriptCommandList;
import org.bidib.wizard.script.node.NodeScriptCommandList.ExecutionStatus;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class NodeScriptModel extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(NodeScriptModel.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTYNAME_NODE_SCRIPT_NAME = "nodeScriptName";
public static final String PROPERTYNAME_SELECTED_NODE = "selectedNode";
public static final String PROPERTYNAME_NODE_SCRIPT_COMMAND_LIST = "nodeScriptCommandList";
public static final String PROPERTYNAME_CAN_EXECUTE_SCRIPT = "canExecuteScript";
private String nodeScriptName;
private Node selectedNode;
private NodeScriptCommandList nodeScriptCommandList;
private Map context;
private boolean canExecuteScript;
/**
* @return the nodeScriptName
*/
public String getNodeScriptName() {
return nodeScriptName;
}
/**
* @param nodeScriptName
* the nodeScriptName to set
*/
public void setNodeScriptName(String nodeScriptName) {
String oldValue = this.nodeScriptName;
this.nodeScriptName = nodeScriptName;
firePropertyChange(PROPERTYNAME_NODE_SCRIPT_NAME, oldValue, nodeScriptName);
}
/**
* @return the selectedNode
*/
public Node getSelectedNode() {
return selectedNode;
}
/**
* @param selectedNode
* the selectedNode to set
*/
public void setSelectedNode(Node selectedNode) {
Node oldValue = this.selectedNode;
this.selectedNode = selectedNode;
firePropertyChange(PROPERTYNAME_SELECTED_NODE, oldValue, selectedNode);
updateCanExecuteScript();
}
/**
* @return the nodeScriptCommandList
*/
public NodeScriptCommandList getNodeScriptCommandList() {
return nodeScriptCommandList;
}
private PropertyChangeListener nodeScriptCommandListListner = new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
updateCanExecuteScript();
}
};
/**
* @param nodeScriptCommands
* the nodeScriptCommands to set
*/
public void setNodeScriptCommandList(NodeScriptCommandList nodeScriptCommandList, final Map context) {
NodeScriptCommandList oldValue = this.nodeScriptCommandList;
if (this.nodeScriptCommandList != null) {
this.nodeScriptCommandList.removePropertyChangeListener(
NodeScriptCommandList.PROPERTYNAME_EXECUTION_STATUS, nodeScriptCommandListListner);
}
this.nodeScriptCommandList = nodeScriptCommandList;
this.context = context;
if (this.nodeScriptCommandList != null) {
this.nodeScriptCommandList.addPropertyChangeListener(NodeScriptCommandList.PROPERTYNAME_EXECUTION_STATUS,
nodeScriptCommandListListner);
}
firePropertyChange(PROPERTYNAME_NODE_SCRIPT_COMMAND_LIST, oldValue, nodeScriptCommandList);
updateCanExecuteScript();
}
private void updateCanExecuteScript() {
boolean isValidList = true;
if (nodeScriptCommandList != null) {
// if the script command list is assigned we must evaluate the execution status
LOGGER.info("Current nodeScriptCommandList: {}", nodeScriptCommandList);
isValidList = !ExecutionStatus.running.equals(nodeScriptCommandList.getExecutionStatus());
}
boolean isNodeSelected = (selectedNode != null);
LOGGER.info("updateCanExecuteScript, isValidList: {}, isNodeSelected: {}", isValidList, isNodeSelected);
setCanExecuteScript(isValidList && isNodeSelected);
}
/**
* @return the canExecuteScript
*/
public boolean isCanExecuteScript() {
return canExecuteScript;
}
/**
* @param canExecuteScript
* the canExecuteScript to set
*/
public void setCanExecuteScript(boolean canExecuteScript) {
boolean oldValue = this.canExecuteScript;
this.canExecuteScript = canExecuteScript;
firePropertyChange(PROPERTYNAME_CAN_EXECUTE_SCRIPT, oldValue, canExecuteScript);
}
public Map getContext() {
return context;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy