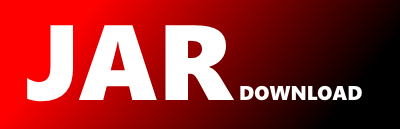
org.bidib.wizard.mvc.simulation.view.SimulationView Maven / Gradle / Ivy
package org.bidib.wizard.mvc.simulation.view;
import java.awt.Component;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.LinkedHashMap;
import java.util.Map;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import org.bidib.jbidibc.simulation.SimulatorNode;
import org.bidib.wizard.mvc.main.model.Node;
import org.bidib.wizard.mvc.simulation.view.panel.SimulationViewContainer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.vlsolutions.swing.docking.DockKey;
import com.vlsolutions.swing.docking.Dockable;
import com.vlsolutions.swing.docking.DockableState;
import com.vlsolutions.swing.docking.DockingDesktop;
import com.vlsolutions.swing.docking.DockingUtilities;
import com.vlsolutions.swing.docking.RelativeDockablePosition;
import com.vlsolutions.swing.docking.TabbedDockableContainer;
public class SimulationView implements Dockable, SimulationViewContainer {
private static final Logger LOGGER = LoggerFactory.getLogger(SimulationView.class);
private final DockKey DOCKKEY;
private Map mapUUIDtoSimulation = new LinkedHashMap<>();
private final SimulatorProvider simulatorProvider;
private final DockingDesktop desktop;
private JPanel simulationPanel;
public SimulationView(SimulatorProvider simulatorProvider, final DockingDesktop desktop) {
this.simulatorProvider = simulatorProvider;
this.desktop = desktop;
DOCKKEY = new DockKey("simulationView");
// enable floating
DOCKKEY.setFloatEnabled(true);
simulationPanel = new JPanel();
simulationPanel.add(new JLabel("This is the simulation view."));
}
@Override
public DockKey getDockKey() {
return DOCKKEY;
}
@Override
public Component getComponent() {
return simulationPanel;
}
private void createSimulationNode(Node node, DockingDesktop desktop) {
LOGGER.info("Create new simulation node: {}", node);
SimulationNodePanel simulationNodePanel = mapUUIDtoSimulation.get(node);
if (simulationNodePanel == null) {
LOGGER.info("Create new simulationNodePanel for node: {}", node);
SimulatorNode simulator = simulatorProvider.getSimulator(node);
String simulationPanelClass = simulator.getSimulationPanelClass();
simulationNodePanel = createSimulationNodePanel(simulationPanelClass, node);
if (simulationNodePanel == null) {
LOGGER.warn("No simulationNodePanel available for node: {}", node);
return;
}
simulationNodePanel.createComponents(simulator);
mapUUIDtoSimulation.put(node, simulationNodePanel);
}
LOGGER.info("Adding the new simulationNodePanel.");
addSimulationNodeTab(desktop, simulationNodePanel);
}
private SimulationNodePanel createSimulationNodePanel(String className, Node node) {
LOGGER.info("Create new SimulationNodePanel of class: {}, node: {}", className, node);
SimulationNodePanel simulationNodePanel = null;
try {
Class clazz = (Class) Class.forName(className);
Constructor ctor =
clazz.getDeclaredConstructor(SimulationViewContainer.class, Node.class);
simulationNodePanel = (SimulationNodePanel) ctor.newInstance(this, node);
}
catch (ClassNotFoundException | NoSuchMethodException | SecurityException | InstantiationException
| IllegalAccessException | IllegalArgumentException | InvocationTargetException e) {
LOGGER.warn("Create instance of SimulationNodePanel failed.", e);
}
return simulationNodePanel;
}
private void addSimulationNodeTab(DockingDesktop desktop, SimulationNodePanel simulationNodePanel) {
LOGGER.info("Create new tab to add the simulationNodePanel.");
int order = 1;
TabbedDockableContainer container = DockingUtilities.findTabbedDockableContainer(this);
if (container != null) {
order = container.getTabCount();
}
LOGGER.info("Add new simulationNodePanel at order: {}", order);
Dockable base = this;
DockableState dockable = desktop.getDockableState(base);
if (dockable == null || dockable.isClosed()) {
simulatorProvider.restoreSimulationView();
}
desktop.addDockable(simulationNodePanel, RelativeDockablePosition.BOTTOM_RIGHT);
desktop.addDockableStateChangeListener(simulationNodePanel);
// desktop.createTab(this, simulationNodePanel, order, true);
}
public void activate(Node node, DockingDesktop desktop) {
SimulationNodePanel simulationNodePanel = mapUUIDtoSimulation.get(node);
if (simulationNodePanel != null) {
DockableState dockable = desktop.getDockableState(simulationNodePanel);
if (dockable == null || dockable.isClosed()) {
LOGGER.info("simulationNodePanel is closed or hidden.");
addSimulationNodeTab(desktop, simulationNodePanel);
}
else if (dockable.isHidden()) {
LOGGER.info("Restore the hidden dockable.");
desktop.setAutoHide(dockable.getDockable(), false);
}
else {
selectWindow(simulationNodePanel);
}
}
else {
createSimulationNode(node, desktop);
}
}
private void selectWindow(Dockable dockable) {
TabbedDockableContainer container = DockingUtilities.findTabbedDockableContainer(dockable);
if (container != null) {
container.setSelectedDockable(dockable);
}
else {
LOGGER.warn("Container not available, select component directly.");
dockable.getComponent().requestFocusInWindow();
}
}
@Override
public void close(final SimulationNodePanel simulationNodePanel) {
LOGGER.info("Close simulationNodePanel: {}", simulationNodePanel);
SwingUtilities.invokeLater(new Runnable() {
public void run() {
mapUUIDtoSimulation.remove(simulationNodePanel.getNode());
DockableState dockable = desktop.getDockableState(simulationNodePanel);
if (dockable != null && !dockable.isClosed()) {
desktop.removeDockableStateChangeListener(simulationNodePanel);
desktop.unregisterDockable(simulationNodePanel);
}
else {
LOGGER.info("The current simulation panel is not registered on the desktop.");
}
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy