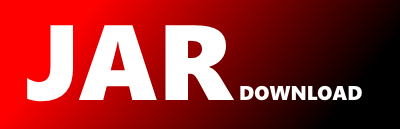
org.bidib.wizard.mvc.stepcontrol.model.StepControlAspect Maven / Gradle / Ivy
package org.bidib.wizard.mvc.stepcontrol.model;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import com.jgoodies.binding.beans.Model;
public class StepControlAspect extends Model {
private static final long serialVersionUID = 1L;
public static final String PROPERTYNAME_STEP_NUMBER = "stepNumber";
public static final String PROPERTYNAME_POSITION = "position";
public static final String PROPERTYNAME_POLARITY = "polarity";
public static final String PROPERTYNAME_STATUS = "status";
public enum Polarity {
normal(0), inverted(1);
int cvValue;
Polarity(int cvValue) {
this.cvValue = cvValue;
}
public int getCvValue() {
return cvValue;
}
public static Polarity valueOf(int cvValue) {
Polarity polarity = null;
for (Polarity e : values()) {
if (e.getCvValue() == cvValue) {
polarity = e;
break;
}
}
if (polarity == null) {
throw new IllegalArgumentException("Cannot map " + cvValue + " to a polarity.");
}
return polarity;
}
}
private Integer stepNumber;
private long position;
private Polarity polarity;
public StepControlAspect(Integer stepNumber, long position, Polarity polarity) {
this.stepNumber = stepNumber;
this.position = position;
this.polarity = polarity;
}
/**
* @return the stepNumber
*/
public Integer getStepNumber() {
return stepNumber;
}
/**
* @param stepNumber
* the stepNumber to set
*/
public void setStepNumber(Integer stepNumber) {
Integer oldValue = this.stepNumber;
this.stepNumber = stepNumber;
firePropertyChange(PROPERTYNAME_STEP_NUMBER, oldValue, stepNumber);
}
/**
* @return the position
*/
public long getPosition() {
return position;
}
/**
* @param position
* the position to set
*/
public void setPosition(long position) {
long oldValue = this.position;
this.position = position;
firePropertyChange(PROPERTYNAME_POSITION, oldValue, position);
}
/**
* @return the polarity
*/
public Polarity getPolarity() {
return polarity;
}
/**
* @param polarity
* the polarity to set
*/
public void setPolarity(Polarity polarity) {
Polarity oldValue = this.polarity;
this.polarity = polarity;
firePropertyChange(PROPERTYNAME_POLARITY, oldValue, polarity);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy