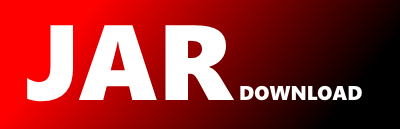
org.bidib.wizard.mvc.stepcontrol.model.StepControlModel Maven / Gradle / Ivy
package org.bidib.wizard.mvc.stepcontrol.model;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.collections4.CollectionUtils;
import org.bidib.jbidibc.core.utils.ByteUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
import com.jgoodies.common.collect.ArrayListModel;
public class StepControlModel extends Model {
private static final long serialVersionUID = 1L;
public enum TurnTableType {
linear(0), round(1);
private byte type;
TurnTableType(int type) {
this.type = ByteUtils.getLowByte(type);
}
public byte getType() {
return type;
}
public int getCvValue() {
return ByteUtils.getInt(type);
}
public static TurnTableType fromValue(byte type) {
TurnTableType result = null;
for (TurnTableType e : values()) {
if (e.type == type) {
result = e;
break;
}
}
if (result == null) {
throw new IllegalArgumentException("cannot map " + type + " to a turnTableType enum");
}
return result;
}
}
private static final Logger LOGGER = LoggerFactory.getLogger(StepControlModel.class);
public static final String PROPERTYNAME_SELECTED_ASPECT = "selectedAspect";
public static final String PROPERTYNAME_STEPCONTROL_ASPECTS = "stepControlAspects";
public static final String PROPERTYNAME_CURRENT_POSITION = "currentPosition";
public static final String PROPERTYNAME_TURNTABLE_TYPE = "turnTableType";
private ArrayListModel stepControlAspects = new ArrayListModel<>();
private StepControlAspect selectedAspect;
private TurnTableType turnTableType;
private long currentPosition;
/**
* @return the selectedAspect
*/
public StepControlAspect getSelectedAspect() {
return selectedAspect;
}
/**
* @param selectedAspect
* the selectedAspect to set
*/
public void setSelectedAspect(StepControlAspect selectedAspect) {
StepControlAspect oldValue = this.selectedAspect;
this.selectedAspect = selectedAspect;
firePropertyChange(PROPERTYNAME_SELECTED_ASPECT, oldValue, selectedAspect);
}
/**
* @return the stepControlAspects list model
*/
public ArrayListModel getStepControlAspectsListModel() {
return stepControlAspects;
}
/**
* @return the stepControlAspects
*/
public List getStepControlAspects() {
return stepControlAspects;
}
/**
* @param stepControlAspect
* the single stepControlAspect to add
*/
public void addStepControlAspect(StepControlAspect stepControlAspect) {
List oldValue = new ArrayList<>();
oldValue.addAll(this.stepControlAspects);
// TODO fix this hack
if (stepControlAspect.getStepNumber() == null) {
int size = stepControlAspects.size();
stepControlAspect.setStepNumber(size + 1);
}
this.stepControlAspects.add(stepControlAspect);
firePropertyChange(PROPERTYNAME_STEPCONTROL_ASPECTS, oldValue, stepControlAspects);
}
/**
* @param stepControlAspect
* the single stepControlAspect to remove
*/
public void removeStepControlAspect(StepControlAspect stepControlAspect) {
List oldValue = new ArrayList<>();
oldValue.addAll(this.stepControlAspects);
this.stepControlAspects.remove(stepControlAspect);
firePropertyChange(PROPERTYNAME_STEPCONTROL_ASPECTS, oldValue, stepControlAspects);
}
/**
* @param stepControlAspects
* the stepControlAspects to set
*/
public void setStepControlAspects(List stepControlAspects) {
List oldValue = new ArrayList<>();
oldValue.addAll(this.stepControlAspects);
this.stepControlAspects.clear();
if (CollectionUtils.isNotEmpty(stepControlAspects)) {
this.stepControlAspects.addAll(stepControlAspects);
}
firePropertyChange(PROPERTYNAME_STEPCONTROL_ASPECTS, oldValue, this.stepControlAspects);
}
/**
* @return the turnTableType
*/
public TurnTableType getTurnTableType() {
return turnTableType;
}
/**
* @param turnTableType
* the turnTableType to set
*/
public void setTurnTableType(TurnTableType turnTableType) {
LOGGER.info("Set the turnTableType: {}", turnTableType);
TurnTableType oldValue = this.turnTableType;
this.turnTableType = turnTableType;
firePropertyChange(PROPERTYNAME_TURNTABLE_TYPE, oldValue, turnTableType);
}
/**
* @return the currentPosition
*/
public long getCurrentPosition() {
return currentPosition;
}
/**
* @param currentPosition
* the currentPosition to set
*/
public void setCurrentPosition(long currentPosition) {
LOGGER.info("Set the current position: {}", currentPosition);
long oldValue = this.currentPosition;
this.currentPosition = currentPosition;
firePropertyChange(PROPERTYNAME_CURRENT_POSITION, oldValue, currentPosition);
}
/**
* Clear all values from the model.
*/
public void clearModel() {
setStepControlAspects(null);
setCurrentPosition(0);
setSelectedAspect(null);
setTurnTableType(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy