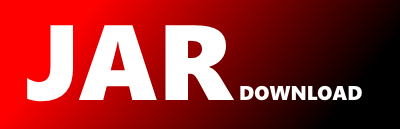
org.bidib.wizard.script.node.AddAspectCommand Maven / Gradle / Ivy
package org.bidib.wizard.script.node;
import java.util.Map;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.lang3.StringUtils;
import org.bidib.wizard.highlight.BidibScriptScanner;
import org.bidib.wizard.highlight.Scanner;
import org.bidib.wizard.highlight.Token;
import org.bidib.wizard.highlight.TokenTypes;
import org.bidib.wizard.mvc.script.view.NodeScripting;
import org.bidib.wizard.mvc.script.view.ScriptParser;
import org.bidib.wizard.script.AbstractScriptCommand;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class AddAspectCommand extends AbstractScriptCommand {
private static final Logger LOGGER = LoggerFactory.getLogger(AddAspectCommand.class);
public static final String KEY = "setLabel";
private Long uuid;
private Integer macroNumber;
private String macroLabel;
protected AddAspectCommand() {
super(KEY);
}
public AddAspectCommand(Long uuid) {
super(KEY);
this.uuid = uuid;
}
/**
* @return the macroNumber
*/
public Integer getMacroNumber() {
return macroNumber;
}
/**
* @param macroNumber
* the macroNumber to set
*/
public void setMacroNumber(Integer macroNumber) {
this.macroNumber = macroNumber;
}
/**
* @return the macroLabel
*/
public String getMacroLabel() {
return macroLabel;
}
/**
* @param macroLabel
* the macroLabel to set
*/
public void setMacroLabel(String macroLabel) {
this.macroLabel = macroLabel;
}
@Override
public void parse(String commandLine) {
// TODO Auto-generated method stub
LOGGER.info("Set the aspect: {}", commandLine);
// label = commandLine;
}
@Override
protected void internalExecute(final NodeScripting scripting, final Map context) {
}
public void scan(Scanner scanner, int index, final Map context) {
// add aspect 1 macroname=%weiche1Gerade%
for (int i = index + 1; i < scanner.size(); i++) {
Token token = scanner.getToken(i);
LOGGER.info("scan, current index: {}, token symbol: {}, name: {}", i, token.symbol.type, token.symbol.name);
switch (token.symbol.type) {
case TokenTypes.KEYWORD2:
switch (token.symbol.name) {
case BidibScriptScanner.KEY2_MACRONAME:
// macroname detected
StringValueCallback labelAware = new StringValueCallback() {
@Override
public void setString(String value) {
LOGGER.info("Set the macro name: {}", value);
setMacroLabel(value);
}
};
i = NodeScriptUtils.parseLabel(scanner, i, labelAware, context);
break;
case BidibScriptScanner.KEY2_MACRONUMBER:
NumberAware numberAware = new NumberAware() {
@Override
public void setNumber(Integer number) {
LOGGER.info("Set the macronumber: {}", number);
setMacroNumber(number);
}
};
i = NodeScriptUtils.parseNumber(scanner, i, numberAware, context);
break;
default:
break;
}
break;
default:
break;
}
LOGGER.info("scan, i after parse: {}, last symbol name: {}", i, token.symbol.name);
}
}
public void prepareAccessory(final Map context) {
if (macroNumber == null) {
// resolve the macro
if (StringUtils.isNotBlank(macroLabel)) {
// get the corresponding macro number
// MacroLabels macroLabels =
// DefaultApplicationContext.getInstance().get(DefaultApplicationContext.KEY_MACRO_LABELS,
// MacroLabels.class);
// Integer macroId = macroLabels.getIdByLabel(uuid, macroLabel);
Map macroLabels = (Map) context.get(ScriptParser.KEY_MACRO_LABELS);
Integer macroId = MapUtils.invertMap(macroLabels).get(macroLabel);
if (macroId != null) {
setMacroNumber(macroId);
}
else {
LOGGER.warn("No corresponding macro number found for macroName: {}", macroLabel);
}
}
else {
LOGGER.warn("No macro defined for aspect: {}", this);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy