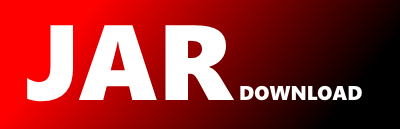
org.bidib.wizard.script.node.ConfigMacroTimeCommand Maven / Gradle / Ivy
package org.bidib.wizard.script.node;
import java.util.HashMap;
import java.util.Map;
import org.bidib.wizard.highlight.BidibScriptScanner;
import org.bidib.wizard.highlight.Scanner;
import org.bidib.wizard.highlight.Token;
import org.bidib.wizard.highlight.TokenTypes;
import org.bidib.wizard.mvc.script.view.NodeScripting;
import org.bidib.wizard.script.AbstractScriptCommand;
import org.bidib.wizard.script.InitializingCommand;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ConfigMacroTimeCommand extends AbstractScriptCommand implements InitializingCommand {
private static final Logger LOGGER = LoggerFactory.getLogger(ConfigMacroTimeCommand.class);
public static final String KEY = "configMacroTime";
private Long uuid;
private Map macroTimeConfig = new HashMap();
protected ConfigMacroTimeCommand() {
super(KEY);
}
public ConfigMacroTimeCommand(Long uuid) {
super(KEY);
this.uuid = uuid;
}
public Map getMacroTimeConfig() {
return macroTimeConfig;
}
@Override
public void parse(String commandLine) {
LOGGER.info("Parse the command line: {}", commandLine);
}
public int scan(Scanner scanner, int index, final Map context) {
int i = index + 1;
for (; i < scanner.size(); i++) {
Token token = scanner.getToken(i);
LOGGER.info("scan, current index: {}, token symbol: {}, name: {}", i, token.symbol.type, token.symbol.name);
switch (token.symbol.type) {
case TokenTypes.KEYWORD2:
switch (token.symbol.name) {
case BidibScriptScanner.KEY2_DAY:
LOGGER.info("Day detected: {}", token.symbol.name);
NumberAware dayCallback = new NumberAware() {
@Override
public void setNumber(Integer number) {
LOGGER.info("Set the day: {}", number);
macroTimeConfig.put("day", number);
}
};
i = NodeScriptUtils.parseMacroTimeDay(scanner, i, dayCallback, context);
break;
case BidibScriptScanner.KEY2_HOUR:
LOGGER.info("Hour detected: {}", token.symbol.name);
NumberAware hourCallback = new NumberAware() {
@Override
public void setNumber(Integer number) {
LOGGER.info("Set the hour: {}", number);
macroTimeConfig.put("hour", number);
}
};
i = NodeScriptUtils.parseMacroTimeHour(scanner, i, hourCallback, context);
break;
case BidibScriptScanner.KEY2_MINUTE:
LOGGER.info("Minute detected: {}", token.symbol.name);
NumberAware minuteCallback = new NumberAware() {
@Override
public void setNumber(Integer number) {
LOGGER.info("Set the minute: {}", number);
macroTimeConfig.put("minute", number);
}
};
i = NodeScriptUtils.parseMacroTimeMinute(scanner, i, minuteCallback, context);
break;
default:
break;
}
break;
default:
break;
}
LOGGER.info("scan, i after parse: {}, last symbol name: {}", i, token.symbol.name);
}
return i;
}
@Override
protected void internalExecute(final NodeScripting scripting, final Map context) {
LOGGER.info("Set the macro config: {}", this);
}
public void afterPropertiesSet() {
LOGGER.info("afterPropertiesSet is called: {}", this);
}
@Override
public String toString() {
StringBuffer sb = new StringBuffer("[");
sb.append(getClass().getSimpleName());
sb.append(", uuid: ").append(uuid).append(", macroTimeConfig: ").append(macroTimeConfig);
sb.append("]");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy