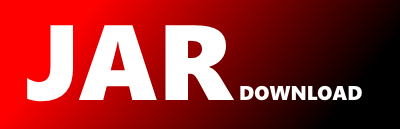
org.bidib.wizard.script.node.SelectMacroCommand Maven / Gradle / Ivy
package org.bidib.wizard.script.node;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.collections4.MapUtils;
import org.bidib.wizard.highlight.BidibScriptScanner;
import org.bidib.wizard.highlight.Scanner;
import org.bidib.wizard.highlight.Token;
import org.bidib.wizard.highlight.TokenTypes;
import org.bidib.wizard.mvc.main.model.Macro;
import org.bidib.wizard.mvc.main.model.MacroUtils;
import org.bidib.wizard.mvc.main.model.function.Function;
import org.bidib.wizard.mvc.script.view.NodeScripting;
import org.bidib.wizard.mvc.script.view.ScriptParser;
import org.bidib.wizard.script.AbstractScriptCommand;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class SelectMacroCommand extends AbstractScriptCommand {
private static final Logger LOGGER = LoggerFactory.getLogger(SelectMacroCommand.class);
public static final String KEY = "selectMacro";
private Long uuid;
private String label;
private Integer macroNumber;
private ConfigMacroCommand configMacro;
private ConfigMacroTimeCommand configMacroTime;
private List macroSteps;
protected SelectMacroCommand() {
super(KEY);
}
public SelectMacroCommand(Long uuid) {
super(KEY);
this.uuid = uuid;
}
/**
* @return the macroNumber
*/
public Integer getMacroNumber() {
return macroNumber;
}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
/**
* @param macroNumber
* the macroNumber to set
*/
public void setMacroNumber(Integer macroNumber) {
this.macroNumber = macroNumber;
}
public void addMacroStep(AddMacroStepCommand macroStep) {
if (macroSteps == null) {
macroSteps = new LinkedList<>();
}
LOGGER.info("Add new macroStep: {}", macroStep);
macroSteps.add(macroStep);
}
public List getMacroSteps() {
return macroSteps;
}
/**
* @return the configMacro
*/
public ConfigMacroCommand getConfigMacro() {
return configMacro;
}
/**
* @param configMacro
* the configMacro to set
*/
public void setConfigMacro(ConfigMacroCommand configMacro) {
this.configMacro = configMacro;
}
/**
* @param configMacroTime
* the configMacroTime to set
*/
public void setConfigMacroTime(ConfigMacroTimeCommand configMacroTime) {
this.configMacroTime = configMacroTime;
}
@Override
public void parse(String commandLine) {
// TODO Auto-generated method stub
LOGGER.info("Set the label: {}", commandLine);
// label = commandLine;
}
public void scan(Scanner scanner, int index, final Map context) {
for (int i = index + 1; i < scanner.size(); i++) {
Token token = scanner.getToken(i);
LOGGER.info("scan, current index: {}, token symbol: {}, name: {}", i, token.symbol.type, token.symbol.name);
switch (token.symbol.type) {
case TokenTypes.KEYWORD2:
switch (token.symbol.name) {
// case BidibScriptScanner.KEY2_LABEL:
case BidibScriptScanner.KEY2_NAME:
// label detected
StringValueCallback labelAware = new StringValueCallback() {
@Override
public void setString(String label) {
LOGGER.info("Set the port label: {}", label);
setLabel(label);
}
};
i += NodeScriptUtils.parseLabel(scanner, i, labelAware, context);
break;
default:
break;
}
break;
case TokenTypes.NUMBER:
// set the macro number
setMacroNumber(Integer.valueOf(token.symbol.name));
break;
case TokenTypes.VARIABLE:
if (i == 2) {
// set the macro number
LOGGER.info("Set the port number based on a variable: {}", token.symbol.name);
String variable = token.symbol.name;
Object value = context.get(variable);
if (value == null) {
// not found -> use the variable
value = variable;
}
// TODO if this should work we must have a map of macro label to macro
if (value instanceof String) {
String macroName = (String) value;
LOGGER.info("Search for macro with name: {}", macroName);
// get the corresponding macro number
// MacroLabels macroLabels =
// DefaultApplicationContext.getInstance().get(DefaultApplicationContext.KEY_MACRO_LABELS,
// MacroLabels.class);
//
// Integer macroId = macroLabels.getIdByLabel(uuid, macroName);
Map macroLabels =
(Map) context.get(ScriptParser.KEY_MACRO_LABELS);
Integer macroId = MapUtils.invertMap(macroLabels).get(macroName);
if (macroId != null) {
value = macroId;
}
else {
LOGGER.warn("No corresponding macro number found for macroName: {}", macroName);
}
}
setMacroNumber(Integer.valueOf(value.toString()));
}
default:
break;
}
}
}
@Override
protected void internalExecute(final NodeScripting scripting, final Map context) {
LOGGER.info("Select the macro: {}", this);
// TODO create a macro from the provided data
// MacroLabels macroLabels =
// DefaultApplicationContext.getInstance().get(DefaultApplicationContext.KEY_MACRO_LABELS, MacroLabels.class);
Map macroLabels = (Map) context.get(ScriptParser.KEY_MACRO_LABELS);
// TODO get the maximum number of steps from the device ...
Macro macro = new Macro(40);
macro.setLabel(macroLabels.get(macroNumber));
macro.setId(macroNumber);
if (configMacro != null) {
LOGGER.info("The macro has config to set: {}", configMacro);
Integer cycles = configMacro.getMacroConfig().get("repeat");
if (cycles != null) {
macro.setCycles(cycles);
}
Integer slowdown = configMacro.getMacroConfig().get("slowdown");
if (slowdown != null) {
macro.setSpeed(slowdown);
}
}
if (configMacroTime != null) {
LOGGER.info("The macro has config time to set: {}", configMacroTime);
Integer day = configMacroTime.getMacroTimeConfig().get("day");
Integer hour = configMacroTime.getMacroTimeConfig().get("hour");
Integer minute = configMacroTime.getMacroTimeConfig().get("minute");
if (day == null) {
day = 0;
}
if (hour == null) {
hour = 0;
}
if (minute == null) {
minute = 0;
}
MacroUtils.applyMacroStartClk(day, hour, minute, macro);
}
if (CollectionUtils.isNotEmpty(getMacroSteps())) {
List> functions = new LinkedList<>();
for (AddMacroStepCommand command : getMacroSteps()) {
// replace all placeholders in the function
command.prepareFunction(context);
Function> function = command.getFunction();
functions.add(function);
}
macro.setFunctions(functions);
}
else {
LOGGER.info("No macro steps provided.");
}
scripting.setMacro(uuid, macro);
}
@Override
public String toString() {
StringBuffer sb = new StringBuffer("[");
sb.append(getClass().getSimpleName());
sb.append(", uuid: ").append(uuid);
sb.append(", macroNumber: ").append(macroNumber);
sb.append(", steps: ");
if (CollectionUtils.isNotEmpty(macroSteps)) {
for (AddMacroStepCommand step : macroSteps) {
sb.append("\r\n").append(step);
}
}
else {
sb.append("");
}
sb.append("]");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy