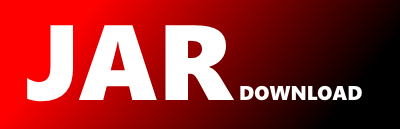
org.bidib.wizard.simulation.OneStepControlSimulator Maven / Gradle / Ivy
package org.bidib.wizard.simulation;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
import org.bidib.jbidibc.core.AddressData;
import org.bidib.jbidibc.core.BidibLibrary;
import org.bidib.jbidibc.core.BidibPort;
import org.bidib.jbidibc.core.Feature;
import org.bidib.jbidibc.core.LcMacro;
import org.bidib.jbidibc.core.enumeration.LcMacroOperationCode;
import org.bidib.jbidibc.core.enumeration.LcMacroState;
import org.bidib.jbidibc.core.enumeration.LcOutputType;
import org.bidib.jbidibc.core.exception.ProtocolException;
import org.bidib.jbidibc.core.message.AccessoryGetMessage;
import org.bidib.jbidibc.core.message.AccessoryParaGetMessage;
import org.bidib.jbidibc.core.message.AccessoryParaResponse;
import org.bidib.jbidibc.core.message.AccessoryParaSetMessage;
import org.bidib.jbidibc.core.message.AccessorySetMessage;
import org.bidib.jbidibc.core.message.AccessoryStateResponse;
import org.bidib.jbidibc.core.message.BidibCommand;
import org.bidib.jbidibc.core.message.BidibMessage;
import org.bidib.jbidibc.core.message.FeedbackAddressResponse;
import org.bidib.jbidibc.core.message.FeedbackConfidenceResponse;
import org.bidib.jbidibc.core.message.FeedbackFreeResponse;
import org.bidib.jbidibc.core.message.FeedbackGetRangeMessage;
import org.bidib.jbidibc.core.message.FeedbackMultipleResponse;
import org.bidib.jbidibc.core.message.FeedbackOccupiedResponse;
import org.bidib.jbidibc.core.message.LcMacroGetMessage;
import org.bidib.jbidibc.core.message.LcMacroHandleMessage;
import org.bidib.jbidibc.core.message.LcMacroParaGetMessage;
import org.bidib.jbidibc.core.message.LcMacroParaResponse;
import org.bidib.jbidibc.core.message.LcMacroParaSetMessage;
import org.bidib.jbidibc.core.message.LcMacroResponse;
import org.bidib.jbidibc.core.message.LcMacroSetMessage;
import org.bidib.jbidibc.core.message.LcMacroStateResponse;
import org.bidib.jbidibc.core.node.MacroFunctionsNode;
import org.bidib.jbidibc.core.utils.ByteUtils;
import org.bidib.jbidibc.core.utils.MacroUtils;
import org.bidib.jbidibc.simulation.SwitchingFunctionsNode;
import org.bidib.jbidibc.simulation.net.SimulationBidibMessageProcessor;
import org.bidib.jbidibc.simulation.nodes.DefaultNodeSimulator;
import org.bidib.jbidibc.simulation.nodes.PortType;
import org.bidib.wizard.comm.FeedbackPortStatus;
import org.bidib.wizard.mvc.main.model.FeedbackAddressData;
import org.bidib.wizard.mvc.main.model.FeedbackPort;
import org.bidib.wizard.mvc.main.model.Port;
import org.bidib.wizard.simulation.events.FeedbackConfidenceSetEvent;
import org.bidib.wizard.simulation.events.FeedbackConfidenceStatusEvent;
import org.bidib.wizard.simulation.events.FeedbackPortSetStatusEvent;
import org.bidib.wizard.simulation.events.FeedbackPortStatusEvent;
import org.bidib.wizard.simulation.macro.MacroContainer;
import org.bushe.swing.event.EventBus;
import org.bushe.swing.event.annotation.AnnotationProcessor;
import org.bushe.swing.event.annotation.EventSubscriber;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class OneStepControlSimulator extends DefaultNodeSimulator implements SwitchingFunctionsNode, MacroFunctionsNode {
private static final Logger LOGGER = LoggerFactory.getLogger(OneStepControlSimulator.class);
private static final String SIMULATION_PANEL_CLASS =
"org.bidib.wizard.mvc.simulation.view.panel.OneStepControlSimulatorPanel";
protected final Map macros = new HashMap();
private final Map feedbackPorts = new HashMap();
private final AtomicBoolean statusFreeze = new AtomicBoolean();
private final AtomicBoolean statusValid = new AtomicBoolean();
private final AtomicBoolean statusSignal = new AtomicBoolean();
public OneStepControlSimulator(byte[] nodeAddress, long uniqueId, boolean autoAddFeature,
SimulationBidibMessageProcessor messageReceiver) {
super(nodeAddress, uniqueId, autoAddFeature, messageReceiver);
}
@Override
public String getSimulationPanelClass() {
return SIMULATION_PANEL_CLASS;
}
@Override
protected void prepareFeatures() {
super.prepareFeatures();
features.add(new Feature(BidibLibrary.FEATURE_BM_SIZE, 4));
// features.add(new Feature(BidibLibrary.FEATURE_BM_ADDR_DETECT_ON, 1));
features.add(new Feature(BidibLibrary.FEATURE_BM_ON, 1));
}
@Override
protected void prepareCVs() {
super.prepareCVs();
configurationVariables.put("1", "1");
configurationVariables.put("2", "13");
configurationVariables.put("3", "120");
configurationVariables.put("4", "0");
// firmware
configurationVariables.put("5", "0");
configurationVariables.put("6", "0");
configurationVariables.put("7", "1");
configurationVariables.put("113", "0"); // current aspect
configurationVariables.put("114", "1"); // type
configurationVariables.put("115", "0"); // reference invalid
configurationVariables.put("116", "32"); // number of steps
configurationVariables.put("117", "0");
configurationVariables.put("118", "0");
configurationVariables.put("119", "0");
configurationVariables.put("120", "112"); // current position
configurationVariables.put("121", "11");
configurationVariables.put("122", "0");
configurationVariables.put("123", "0");
// exit 1
configurationVariables.put("161", "0"); // polarity
configurationVariables.put("162", "0");
configurationVariables.put("163", "0");
configurationVariables.put("164", "0");
configurationVariables.put("165", "0");
// exit 2
configurationVariables.put("166", "0"); // polarity
configurationVariables.put("167", "20");
configurationVariables.put("168", "0");
configurationVariables.put("169", "0");
configurationVariables.put("170", "0");
// exit 3
configurationVariables.put("171", "1"); // polarity
configurationVariables.put("172", "20");
configurationVariables.put("173", "255");
configurationVariables.put("174", "255");
configurationVariables.put("175", "0");
// exit 4
configurationVariables.put("176", "1"); // polarity
configurationVariables.put("177", "0");
configurationVariables.put("178", "0");
configurationVariables.put("179", "255");
configurationVariables.put("180", "255");
// exit 5
configurationVariables.put("181", "1"); // polarity
configurationVariables.put("182", "255");
configurationVariables.put("183", "255");
configurationVariables.put("184", "0");
configurationVariables.put("185", "0");
// exit 6
configurationVariables.put("186", "1"); // polarity
configurationVariables.put("187", "255");
configurationVariables.put("188", "255");
configurationVariables.put("189", "255");
configurationVariables.put("190", "255");
// exit 7
configurationVariables.put("191", "1"); // polarity
configurationVariables.put("192", "0");
configurationVariables.put("193", "255");
configurationVariables.put("194", "0");
configurationVariables.put("195", "0");
// exit 8
configurationVariables.put("196", "1"); // polarity
configurationVariables.put("197", "255");
configurationVariables.put("198", "255");
configurationVariables.put("199", "255");
configurationVariables.put("200", "255");
}
@Override
public void start() {
LOGGER.info("Start the simulator for address: {}", getAddress());
AnnotationProcessor.process(this);
// prepare the feedback ports
setupFeedbackPorts();
super.start();
}
@Override
public void stop() {
AnnotationProcessor.unprocess(this);
super.stop();
}
private void setupFeedbackPorts() {
for (int id = 0; id < 4; id++) {
FeedbackPort port = new FeedbackPort();
port.setId(id);
port.setStatus(id % 2 == 0 ? FeedbackPortStatus.FREE : FeedbackPortStatus.OCCUPIED);
feedbackPorts.put(id, port);
}
}
@Override
protected byte[] prepareResponse(BidibCommand bidibMessage) {
byte[] response = null;
switch (ByteUtils.getInt(bidibMessage.getType())) {
case BidibLibrary.MSG_LC_MACRO_HANDLE:
response = processLcMacroHandleRequest(bidibMessage);
break;
case BidibLibrary.MSG_LC_MACRO_PARA_GET:
response = processLcMacroParaGetRequest(bidibMessage);
break;
case BidibLibrary.MSG_LC_MACRO_PARA_SET:
response = processLcMacroParaSetRequest(bidibMessage);
break;
case BidibLibrary.MSG_LC_MACRO_GET:
response = processLcMacroGetRequest(bidibMessage);
break;
case BidibLibrary.MSG_LC_MACRO_SET:
response = processLcMacroSetRequest(bidibMessage);
break;
case BidibLibrary.MSG_ACCESSORY_SET:
response = processAccessorySetRequest(bidibMessage);
break;
case BidibLibrary.MSG_ACCESSORY_GET:
response = processAccessoryGetRequest(bidibMessage);
break;
case BidibLibrary.MSG_ACCESSORY_PARA_SET:
response = processAccessoryParaSetRequest(bidibMessage);
break;
case BidibLibrary.MSG_ACCESSORY_PARA_GET:
response = processAccessoryParaGetRequest(bidibMessage);
break;
// occupancy
case BidibLibrary.MSG_BM_GET_RANGE:
response = processBmGetRangeRequest(bidibMessage);
break;
case BidibLibrary.MSG_BM_MIRROR_MULTIPLE:
processBmMirrorMultipleRequest(bidibMessage);
break;
case BidibLibrary.MSG_BM_ADDR_GET_RANGE:
processBmAddrGetRangeRequest(bidibMessage);
break;
case BidibLibrary.MSG_BM_GET_CONFIDENCE:
response = processBmGetConfidenceRequest(bidibMessage);
break;
default:
response = super.prepareResponse(bidibMessage);
break;
}
return response;
}
protected byte[] processLcMacroHandleRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the LcMacroHandle request: {}", bidibMessage);
byte[] response = null;
try {
LcMacroHandleMessage lcMacroHandleMessage = (LcMacroHandleMessage) bidibMessage;
Integer macroNumber = lcMacroHandleMessage.getMacroNumber();
LcMacroOperationCode lcMacroOperationCode = lcMacroHandleMessage.getMacroOperationCode();
LOGGER.info("Handle macro request, macroNumber: {}, lcMacroOperationCode: {}", macroNumber,
lcMacroOperationCode);
// TODO store the macro state value
LcMacroState macroState = null;
switch (lcMacroOperationCode) {
case START:
macroState = LcMacroState.RUNNING;
break;
case DELETE:
macroState = LcMacroState.DELETE;
LOGGER.info("Remove macro with number: {}", macroNumber);
macros.remove(macroNumber);
break;
case OFF:
macroState = LcMacroState.OFF;
break;
case RESTORE:
macroState = LcMacroState.RESTORE;
break;
case SAVE:
macroState = LcMacroState.SAVE;
break;
default:
macroState = LcMacroState.NOTEXIST;
break;
}
LcMacroStateResponse lcMacroStateResponse =
new LcMacroStateResponse(bidibMessage.getAddr(), getNextSendNum(), ByteUtils.getLowByte(macroNumber),
macroState);
response = lcMacroStateResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create LcMacroState response failed.", ex);
}
return response;
}
protected byte[] processLcMacroParaGetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the LcMacroParaGet request: {}", bidibMessage);
byte[] response = null;
try {
LcMacroParaGetMessage lcMacroParaGetMessage = (LcMacroParaGetMessage) bidibMessage;
int macroNumber = lcMacroParaGetMessage.getMacroNumber();
int paramId = lcMacroParaGetMessage.getParameterIndex();
LOGGER.info("Process macroNumber: {}, paramId: {}", macroNumber, paramId);
// return the stored parameter value
MacroContainer container = macros.get(macroNumber);
if (container == null) {
LOGGER.info("Create new MacroContainer for macro number: {}", macroNumber);
// initialize a new macro container
container = new MacroContainer(macroNumber);
macros.put(macroNumber, container);
}
byte[] parameter = container.getMacroParameter(paramId);
LcMacroParaResponse lcMacroParaResponse =
new LcMacroParaResponse(bidibMessage.getAddr(), getNextSendNum(), (byte) macroNumber, (byte) paramId,
parameter);
response = lcMacroParaResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create LcMacroPara response failed.", ex);
}
return response;
}
protected byte[] processLcMacroParaSetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the LcMacroParaSet request: {}", bidibMessage);
byte[] response = null;
try {
LcMacroParaSetMessage lcMacroParaSetMessage = (LcMacroParaSetMessage) bidibMessage;
int macroNumber = lcMacroParaSetMessage.getMacroNumber();
int paramId = lcMacroParaSetMessage.getParameterIndex();
byte[] parameter = lcMacroParaSetMessage.getValue();
// store the parameter value
MacroContainer container = macros.get(macroNumber);
if (container == null) {
// initialize a new macro container
container = new MacroContainer(macroNumber);
macros.put(macroNumber, container);
}
container.setMacroParameter(paramId, parameter);
LcMacroParaResponse lcMacroParaResponse =
new LcMacroParaResponse(bidibMessage.getAddr(), getNextSendNum(), (byte) macroNumber, (byte) paramId,
parameter);
response = lcMacroParaResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create LcMacroPara response failed.", ex);
}
return response;
}
protected byte[] processLcMacroGetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the LcMacroGet request: {}", bidibMessage);
byte[] response = null;
try {
LcMacroGetMessage lcMacroGetMessage = (LcMacroGetMessage) bidibMessage;
Integer macroNumber = lcMacroGetMessage.getMacroNumber();
int stepNumber = lcMacroGetMessage.getStep();
// fetch the macro content and test if the macro is available
MacroContainer container = macros.get(macroNumber);
if (container == null) {
// initialize a new macro container
container = new MacroContainer(macroNumber);
macros.put(macroNumber, container);
}
LcMacro macroStep = container.getMacroStep(stepNumber);
LcMacro value = macroStep;
LcMacroResponse lcMacroResponse =
new LcMacroResponse(bidibMessage.getAddr(), getNextSendNum(), ByteUtils.getLowByte(macroNumber),
ByteUtils.getLowByte(stepNumber), value);
response = lcMacroResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create LcMacro response failed.", ex);
}
return response;
}
protected byte[] processLcMacroSetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the LcMacroSet request: {}", bidibMessage);
byte[] response = null;
try {
LcMacroSetMessage lcMacroSetMessage = (LcMacroSetMessage) bidibMessage;
int macroNumber = lcMacroSetMessage.getMacroNumber();
int stepNumber = lcMacroSetMessage.getStep();
LcMacro macroStep = MacroUtils.getMacro(this, lcMacroSetMessage.getData());
LOGGER.info("Current macroNumber: {}, stepNumber: {}, macroStep: {}", macroNumber, stepNumber, macroStep);
// fetch the macro content and test if the macro container is available
MacroContainer container = macros.get(macroNumber);
if (container == null) {
container = new MacroContainer(macroNumber);
macros.put(macroNumber, container);
}
container.setMacroStep(stepNumber, macroStep);
try {
LcMacroResponse lcMacroResponse =
new LcMacroResponse(bidibMessage.getAddr(), getNextSendNum(), ByteUtils.getLowByte(macroNumber),
ByteUtils.getLowByte(stepNumber), macroStep);
response = lcMacroResponse.getContent();
}
catch (NullPointerException npe) {
LOGGER.warn("create response failed.", npe);
}
}
catch (ProtocolException ex) {
LOGGER.warn("Create LcMacro response failed.", ex);
}
return response;
}
protected byte[] processAccessorySetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the AccessorySet request: {}", bidibMessage);
byte[] response = null;
try {
AccessorySetMessage accessorySetMessage = (AccessorySetMessage) bidibMessage;
int accessoryNumber = accessorySetMessage.getAccessoryNumber();
int aspect = accessorySetMessage.getAspect();
byte[] value = new byte[] { 0, 0, 0 };
AccessoryStateResponse accessoryStateResponse =
new AccessoryStateResponse(bidibMessage.getAddr(), getNextSendNum(), (byte) accessoryNumber,
(byte) aspect, value);
response = accessoryStateResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create AccessoryState response failed.", ex);
}
return response;
}
protected byte[] processAccessoryGetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the AccessoryGet request: {}", bidibMessage);
byte[] response = null;
try {
AccessoryGetMessage accessoryGetMessage = (AccessoryGetMessage) bidibMessage;
int accessoryNumber = accessoryGetMessage.getAccessoryNumber();
int aspect = 0;
byte[] value = new byte[] { 0, 0, 0 };
AccessoryStateResponse accessoryStateResponse =
new AccessoryStateResponse(bidibMessage.getAddr(), getNextSendNum(), (byte) accessoryNumber,
(byte) aspect, value);
response = accessoryStateResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create AccessoryState response failed.", ex);
}
return response;
}
protected byte[] processAccessoryParaSetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the AccessoryParaSet request: {}", bidibMessage);
byte[] response = null;
try {
AccessoryParaSetMessage accessoryParaSetMessage = (AccessoryParaSetMessage) bidibMessage;
int accessoryNumber = accessoryParaSetMessage.getAccessoryNumber();
int paraNumber = accessoryParaSetMessage.getParaNumber();
byte[] value = accessoryParaSetMessage.getValue();
AccessoryParaResponse accessoryParaResponse =
new AccessoryParaResponse(bidibMessage.getAddr(), getNextSendNum(),
ByteUtils.getLowByte(accessoryNumber), ByteUtils.getLowByte(paraNumber), value);
response = accessoryParaResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create AccessoryPara response failed.", ex);
}
return response;
}
protected byte[] processAccessoryParaGetRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the AccessoryParaGet request: {}", bidibMessage);
byte[] response = null;
try {
AccessoryParaGetMessage accessoryParaGetMessage = (AccessoryParaGetMessage) bidibMessage;
int accessoryNumber = accessoryParaGetMessage.getAccessoryNumber();
int paraNumber = accessoryParaGetMessage.getParaNumber();
// TODO provide the correct data here ...
byte[] value = new byte[] { 0, 0, 0, 0 };
AccessoryParaResponse accessoryParaResponse =
new AccessoryParaResponse(bidibMessage.getAddr(), getNextSendNum(),
ByteUtils.getLowByte(accessoryNumber), ByteUtils.getLowByte(paraNumber), value);
response = accessoryParaResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create AccessoryPara response failed.", ex);
}
return response;
}
protected byte[] processBmGetRangeRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the FeedbackGetRangeMessage: {}", bidibMessage);
byte[] response = null;
try {
FeedbackGetRangeMessage feedbackGetRangeMessage = (FeedbackGetRangeMessage) bidibMessage;
byte baseAddress = feedbackGetRangeMessage.getBegin();
int end = feedbackGetRangeMessage.getEnd();
byte feedbackSize =
ByteUtils.getLowByte(feedbackGetRangeMessage.getEnd() - feedbackGetRangeMessage.getBegin());
byte value = 0x00;
int index = 0;
int feedbackByteSize = feedbackSize / 8 + (feedbackSize % 8 > 0 ? 1 : 0);
byte[] feedbackMultiple = new byte[feedbackByteSize];
int position = feedbackMultiple.length;
for (int portNum = end; portNum > baseAddress; portNum--) {
value = (byte) ((value & 0xFF) << 1);
FeedbackPort fbp = feedbackPorts.get(portNum - 1);
LOGGER.info("Current status of feedback port: {}", fbp.getStatus());
byte status = (byte) (fbp.getStatus().getType().getType() & 0x01);
value |= status;
feedbackMultiple[position - 1] = value;
index++;
if (index > 7) {
value = 0;
index = 0;
position--;
}
}
LOGGER.info("Prepared feedback multiple: {}", ByteUtils.bytesToHex(feedbackMultiple));
FeedbackMultipleResponse feedbackMultipleResponse =
new FeedbackMultipleResponse(bidibMessage.getAddr(), getNextSendNum(), baseAddress, feedbackSize,
feedbackMultiple);
response = feedbackMultipleResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create feedbackMultiple response failed.", ex);
}
return response;
}
protected void processBmMirrorMultipleRequest(BidibCommand bidibMessage) {
LOGGER.info("Process the FeedbackMirrorMultipleMessage: {}, do nothing ...", bidibMessage);
}
protected void processBmAddrGetRangeRequest(BidibCommand bidibMessage) {
try {
for (FeedbackPort port : feedbackPorts.values()) {
int detectorNumber = port.getId();
Collection bidibAddresses = new ArrayList<>();
Collection addresses = port.getAddresses();
for (FeedbackAddressData addressData : addresses) {
AddressData bidibAddress = new AddressData(addressData.getAddress(), addressData.getType());
bidibAddresses.add(bidibAddress);
}
FeedbackAddressResponse feedbackAddressResponse =
new FeedbackAddressResponse(bidibMessage.getAddr(), getNextSendNum(), detectorNumber,
bidibAddresses);
byte[] response = feedbackAddressResponse.getContent();
LOGGER.info("Prepare feedbackAddressResponse: {}", ByteUtils.bytesToHex(response));
sendSpontanousResponse(response);
}
}
catch (ProtocolException ex) {
LOGGER.warn("Create feedbackAddress response failed.", ex);
}
}
protected byte[] processBmGetConfidenceRequest(BidibCommand bidibMessage) {
byte[] response = null;
try {
byte valid = (byte) (statusValid.get() ? 1 : 0);
byte freeze = (byte) (statusFreeze.get() ? 1 : 0);
byte signal = (byte) (statusSignal.get() ? 1 : 0);
// TODO if more than a single GBM16T is attached we must set more bits? See 4.7.4. Uplink: Nachrichten für
// Belegtmelder --> MSG_BM_CONFIDENCE
// Test with real system: See MainMessageListener.confidence()
FeedbackConfidenceResponse feedbackConfidenceResponse =
new FeedbackConfidenceResponse(bidibMessage.getAddr(), getNextSendNum(), valid, freeze, signal);
response = feedbackConfidenceResponse.getContent();
}
catch (ProtocolException ex) {
LOGGER.warn("Create feedbackConfidence response failed.", ex);
}
return response;
}
private void publishFeedbackPortChange(Port> port) {
FeedbackPort feedbackPort = (FeedbackPort) port;
FeedbackPortStatus status = feedbackPort.getStatus();
LOGGER.info("The feedbackport status has changed, notify the listeners, nodeAddress: {}", nodeAddress);
EventBus.publish(new FeedbackPortStatusEvent(ByteUtils.bytesToHex(nodeAddress), feedbackPort, status));
}
@Override
public void queryStatus(Class> portClass) {
if (FeedbackPort.class.equals(portClass)) {
for (FeedbackPort feedbackPort : feedbackPorts.values()) {
publishFeedbackPortChange(feedbackPort);
}
// publish the confidence
publishFeedbackConfidenceStatusEvent(statusValid.get(), statusFreeze.get(), statusSignal.get());
}
}
@EventSubscriber(eventClass = FeedbackConfidenceSetEvent.class)
public void feedbackConfidenceSetEvent(FeedbackConfidenceSetEvent feedbackConfidenceEvent) {
String nodeAddress = feedbackConfidenceEvent.getNodeAddr();
LOGGER.info("The change of the feedback confidence was requested, nodeAddress: {}", nodeAddress);
// check if the node is addressed
if (!isAddressEqual(nodeAddress)) {
LOGGER.trace("Another node is addressed.");
return;
}
statusValid.set(feedbackConfidenceEvent.getValid());
statusFreeze.set(feedbackConfidenceEvent.getFreeze());
statusSignal.set(feedbackConfidenceEvent.getSignal());
byte valid = (byte) (statusValid.get() ? 1 : 0);
byte freeze = (byte) (statusFreeze.get() ? 1 : 0);
byte signal = (byte) (statusSignal.get() ? 1 : 0);
try {
FeedbackConfidenceResponse feedbackConfidenceResponse =
new FeedbackConfidenceResponse(this.nodeAddress, getNextSendNum(), valid, freeze, signal);
LOGGER.info("Prepared feedbackConfidenceResponse: {}", feedbackConfidenceResponse);
sendSpontanousResponse(feedbackConfidenceResponse.getContent());
}
catch (ProtocolException ex) {
LOGGER.warn("Send feedbackConfidenceResponse failed.", ex);
}
publishFeedbackConfidenceStatusEvent(statusValid.get(), statusFreeze.get(), statusSignal.get());
}
private void publishFeedbackConfidenceStatusEvent(boolean valid, boolean freeze, boolean signal) {
LOGGER.info("The feedbackport confidence status has changed, notify the listeners, nodeAddress: {}",
nodeAddress);
EventBus.publish(new FeedbackConfidenceStatusEvent(ByteUtils.bytesToHex(nodeAddress), valid, freeze, signal));
}
@EventSubscriber(eventClass = FeedbackPortSetStatusEvent.class)
public void feedbackPortSetStatus(FeedbackPortSetStatusEvent setStatusEvent) {
LOGGER.info("The change of the feedback port was requested.");
String nodeAddress = setStatusEvent.getNodeAddr();
// check if the node is addressed
if (!isAddressEqual(nodeAddress)) {
LOGGER.trace("Another node is addressed.");
return;
}
int portNum = setStatusEvent.getPortNum();
try {
changeFeedbackPortStatus(portNum);
}
catch (ProtocolException ex) {
LOGGER.warn("Publish feedback status failed.", ex);
}
}
protected void changeFeedbackPortStatus(int portNum) throws ProtocolException {
FeedbackPort port = feedbackPorts.get(portNum);
if (port != null) {
BidibMessage response = null;
switch (port.getStatus()) {
case FREE:
port.setStatus(FeedbackPortStatus.OCCUPIED);
response = new FeedbackOccupiedResponse(getNodeAddress(), getNextSendNum(), portNum);
break;
default:
port.setStatus(FeedbackPortStatus.FREE);
response = new FeedbackFreeResponse(getNodeAddress(), getNextSendNum(), portNum);
break;
}
sendSpontanousResponse(response.getContent());
publishFeedbackPortChange(port);
}
else {
LOGGER.warn("The requested feedback port is not available: {}", portNum);
}
}
@Override
public void setPortsConfig(PortType portType) {
// TODO Auto-generated method stub
}
@Override
public boolean isPortFlatModelAvailable() {
return false;
}
@Override
public LcOutputType getPortType(BidibPort bidibPort) {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy