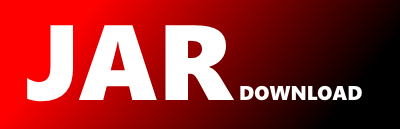
org.bidib.wizard.utils.AccessoryLabelFactory Maven / Gradle / Ivy
package org.bidib.wizard.utils;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Map.Entry;
import java.util.zip.GZIPOutputStream;
import javax.xml.XMLConstants;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.io.IOUtils;
import org.bidib.wizard.labels.LabelNodeType;
import org.bidib.wizard.labels.LabelType;
import org.bidib.wizard.labels.Labels;
import org.bidib.wizard.mvc.main.model.AccessoryLabels;
import org.bidib.wizard.mvc.main.model.ChildLabelMap;
import org.bidib.wizard.mvc.preferences.model.Preferences;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.SAXException;
public class AccessoryLabelFactory {
private static final Logger LOGGER = LoggerFactory.getLogger(AccessoryLabelFactory.class);
private static final String JAXB_PACKAGE = "org.bidib.wizard.labels";
public static final String XSD_LOCATION = "/xsd/wizard-labels.xsd";
public static final String JAXB_SCHEMA_LOCATION = "http://www.bidib.org/wizard/labels xsd/wizard-labels.xsd";
private Labels accessoryLabels;
private Object lock = new Object();
public String getDefaultFileName() {
String result = null;
File baseDir = new File(Preferences.getInstance().getLabelPath());
if (!baseDir.exists()) {
baseDir.mkdir();
}
if (baseDir.exists()) {
result = new File(baseDir, "AccessoryLabels.labels").toString();
}
return result;
}
private Labels loadAccessoryLabelsFile(InputStream is) {
Labels labels = null;
try {
JAXBContext jaxbContext = JAXBContext.newInstance(JAXB_PACKAGE);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
StreamSource streamSource = new StreamSource(AccessoryLabelFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(streamSource);
unmarshaller.setSchema(schema);
labels = (Labels) unmarshaller.unmarshal(is);
}
catch (JAXBException | SAXException ex) {
LOGGER.warn("Load Accessory labels from file failed.", ex);
throw new RuntimeException("Load Accessory labels from file failed.");
}
return labels;
}
/**
* Load the accessory labels from the provided file.
*
* @param filePath
* the file
* @return the accessory labels
*/
public Labels getAccessoryLabels(String filePath) {
synchronized (lock) {
if (accessoryLabels == null) {
LOGGER.info("Load accessoryAspectsLabels from file: {}", filePath);
InputStream is = null;
try {
File file = new File(filePath);
is = new FileInputStream(file);
// else if (filePath.startsWith("/")) {
// LOGGER.info("Try to load accessory labels from resources.");
// is = AccessoryLabelFactory.class.getResourceAsStream(filePath);
//
// if (is == null) {
// throw new FileNotFoundException("The file is not available: " + filePath);
// }
// }
Labels accessoryLabels = loadAccessoryLabelsFile(is);
LOGGER.trace("Loaded accessoryLabels: {}", accessoryLabels);
this.accessoryLabels = accessoryLabels;
}
catch (IllegalArgumentException | FileNotFoundException ex) {
LOGGER.warn("Load saved accessoryLabels from file failed: {}", ex.getMessage());
// check if we can migrate old labels
Labels accessoryLabels = migrateLabels();
// create default labels
if (accessoryLabels == null) {
accessoryLabels = new Labels();
LOGGER.info("Created new default accessoryLabels: {}", accessoryLabels);
}
this.accessoryLabels = accessoryLabels;
// initially save the labels
saveAccessoryLabels(accessoryLabels);
}
}
}
return accessoryLabels;
}
protected Labels migrateLabels() {
LOGGER.info("Try to migrate exisiting accessory labels.");
Labels labels = null;
try {
AccessoryLabels legacyLabels = new AccessoryLabels();
legacyLabels.load();
if (MapUtils.isEmpty(legacyLabels.getLabelMap())) {
LOGGER.info("No legacy labels to migrate found.");
return labels;
}
labels = new Labels();
for (Entry entry : legacyLabels.getLabelMap().entrySet()) {
long currentUniqueId = entry.getKey();
LabelNodeType labelNode = new LabelNodeType();
labelNode.setType("node");
labelNode.setUniqueId(currentUniqueId);
labels.getLabelNode().add(labelNode);
ChildLabelMap childLabels = entry.getValue();
if (MapUtils.isEmpty(childLabels)) {
LOGGER.info("No legacy child labels to migrate found.");
continue;
}
for (Entry currentChildLabel : childLabels.entrySet()) {
int accessoryId = currentChildLabel.getKey();
String labelString = currentChildLabel.getValue();
// create default label
LabelType accessoryLabel = new LabelType();
accessoryLabel.setType("accessory");
accessoryLabel.setIndex(accessoryId);
accessoryLabel.setLabelString(labelString);
labelNode.getLabel().add(accessoryLabel);
}
}
LOGGER.info("Migration of legacy labels passed.");
}
catch (Exception ex) {
LOGGER.warn("Load legacy accessory labels failed.", ex);
}
return labels;
}
/**
* Save the accessory labels
*
* @param accessoryLabels
* the accessory labels
*/
public void saveAccessoryLabels(final Labels accessoryLabels) {
saveAccessoryLabels(accessoryLabels, getDefaultFileName(), true);
}
/**
* Save the accessory labels
*
* @param accessoryLabels
* the accessory labels
* @param fileName
* the filename
* @param gzip
* use gzip
*/
public void saveAccessoryLabels(final Labels accessoryLabels, String fileName, boolean gzip) {
// TODO remove gzip = false
gzip = false;
// TODO implement
LOGGER.info("Save accessoryLabels content to file: {}, accessoryLabels: {}", fileName, accessoryLabels);
ByteArrayOutputStream os = null;
OutputStream fos = null;
try {
JAXBContext jaxbContext = JAXBContext.newInstance(JAXB_PACKAGE);
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.setProperty(Marshaller.JAXB_ENCODING, "UTF-8");
marshaller.setProperty(Marshaller.JAXB_SCHEMA_LOCATION, JAXB_SCHEMA_LOCATION);
// TODO make it work
marshaller.setProperty("com.sun.xml.bind.xmlHeaders",
"\n");
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
StreamSource streamSource = new StreamSource(AccessoryLabelFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(streamSource);
marshaller.setSchema(schema);
os = new ByteArrayOutputStream();
// marshal could fail
marshaller.marshal(accessoryLabels, os);
os.flush();
fos = new BufferedOutputStream(new FileOutputStream(fileName));
if (gzip) {
LOGGER.debug("Use gzip to compress accessoryLabels.");
fos = new GZIPOutputStream(fos);
}
IOUtils.write(os.toByteArray(), fos);
LOGGER.info("Save accessoryLabels content to file passed: {}", fileName);
}
catch (Exception ex) {
// TODO add better exception handling
LOGGER.warn("Save accessoryLabels failed.", ex);
throw new RuntimeException("Save accessoryLabels failed.", ex);
}
finally {
if (fos != null) {
try {
fos.close();
}
catch (IOException ex) {
LOGGER.warn("Close file outputstream failed.", ex);
}
}
if (os != null) {
try {
os.close();
}
catch (IOException ex) {
LOGGER.warn("Close outputstream failed.", ex);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy