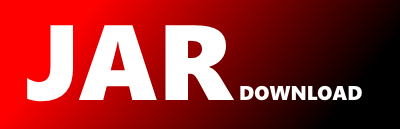
org.bidib.wizard.utils.AccessoryLabelUtils Maven / Gradle / Ivy
package org.bidib.wizard.utils;
import org.apache.commons.collections4.CollectionUtils;
import org.bidib.wizard.labels.ChildLabelType;
import org.bidib.wizard.labels.LabelNodeType;
import org.bidib.wizard.labels.LabelType;
import org.bidib.wizard.labels.Labels;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class AccessoryLabelUtils {
private static final Logger LOGGER = LoggerFactory.getLogger(AccessoryLabelUtils.class);
/**
* Get the accessory labels for the node with the provided uniqueId and the provided accessory id.
*
* @param filePath
* the file path with the label information
* @param uniqueId
* the unique id
* @param accessoryId
* the accessory id
* @return the accessory labels
*/
public static LabelType getAccessoryLabel(Labels labels, long uniqueId, int accessoryId) {
LabelType accessoryLabel = null;
for (LabelNodeType labelNode : labels.getLabelNode()) {
if (labelNode.getUniqueId() == uniqueId) {
// found the node -> search the accessory
for (LabelType label : labelNode.getLabel()) {
if (label.getIndex() == accessoryId) {
// found the accessory
accessoryLabel = label;
break;
}
}
break;
}
}
if (accessoryLabel == null) {
// create default value
accessoryLabel = new LabelType();
accessoryLabel.setType("accessory");
accessoryLabel.setIndex(accessoryId);
}
return accessoryLabel;
}
/**
* Replace the accessory label.
*
* @param labels
* the accessory labels
* @param uniqueId
* the unique id of the node
* @param accessoryId
* the id of the accessory
* @param labelString
* the new label string
*/
public static void replaceLabel(Labels labels, long uniqueId, int accessoryId, String labelString) {
LabelType accessoryLabel = null;
LabelNodeType labelNode = null;
for (LabelNodeType currentLabelNode : labels.getLabelNode()) {
if (currentLabelNode.getUniqueId() == uniqueId) {
// found the node -> search the accessory
labelNode = currentLabelNode;
// search the accessory
for (LabelType label : currentLabelNode.getLabel()) {
if (label.getIndex() == accessoryId) {
// found the accessory
accessoryLabel = label;
break;
}
}
break;
}
}
// make sure we have a labelNode
if (labelNode == null) {
// create new labelNode
labelNode = new LabelNodeType();
labelNode.setType("node");
labelNode.setUniqueId(uniqueId);
labels.getLabelNode().add(labelNode);
}
if (accessoryLabel == null) {
// create default label
accessoryLabel = new LabelType();
accessoryLabel.setType("accessory");
accessoryLabel.setIndex(accessoryId);
accessoryLabel.setLabelString(labelString);
labelNode.getLabel().add(accessoryLabel);
}
else {
// update the label
accessoryLabel.setLabelString(labelString);
}
}
/**
* Replace the accessory label.
*
* @param labels
* the accessory labels
* @param uniqueId
* the unique id of the node
* @param accessoryId
* the id of the accessory
* @param aspectId
* the id of the aspect
* @param labelString
* the new label string
*/
public static void replaceLabel(Labels labels, long uniqueId, int accessoryId, int aspectId, String labelString) {
LabelType accessoryLabel = null;
LabelNodeType labelNode = null;
LabelType aspectLabel = null;
for (LabelNodeType currentLabelNode : labels.getLabelNode()) {
if (currentLabelNode.getUniqueId() == uniqueId) {
// found the node -> search the accessory
labelNode = currentLabelNode;
// search the accessory
for (LabelType label : currentLabelNode.getLabel()) {
if (label.getIndex() == accessoryId) {
// found the accessory
accessoryLabel = label;
LOGGER.info("Found accessoryLabel: {}", accessoryLabel);
// search the aspect
if (accessoryLabel.getChildLabels() != null
&& CollectionUtils.isNotEmpty(accessoryLabel.getChildLabels().getLabel())) {
for (LabelType currentAspectLabel : accessoryLabel.getChildLabels().getLabel()) {
if (currentAspectLabel.getIndex() == aspectId) {
// found the aspect
aspectLabel = currentAspectLabel;
LOGGER.info("Found aspectLabel: {}", aspectLabel);
break;
}
}
}
break;
}
}
break;
}
}
// make sure we have a labelNode
if (labelNode == null) {
// create new labelNode
labelNode = new LabelNodeType();
labelNode.setType("node");
labelNode.setUniqueId(uniqueId);
labels.getLabelNode().add(labelNode);
}
if (accessoryLabel == null) {
// create default label
accessoryLabel = new LabelType();
accessoryLabel.setType("accessory");
accessoryLabel.setIndex(accessoryId);
accessoryLabel.setLabelString(labelString);
labelNode.getLabel().add(accessoryLabel);
}
if (aspectLabel == null) {
// create default label
aspectLabel = new LabelType();
aspectLabel.setType("aspect");
aspectLabel.setIndex(aspectId);
aspectLabel.setLabelString(labelString);
if (accessoryLabel.getChildLabels() == null) {
accessoryLabel.setChildLabels(new ChildLabelType());
}
accessoryLabel.getChildLabels().getLabel().add(aspectLabel);
}
else {
// update the label
aspectLabel.setLabelString(labelString);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy