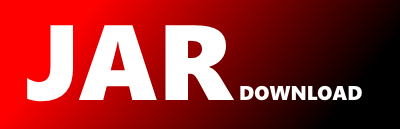
org.bidib.wizard.utils.NodeUtils Maven / Gradle / Ivy
package org.bidib.wizard.utils;
import java.util.Collection;
import org.apache.commons.collections4.Predicate;
import org.apache.commons.lang.StringUtils;
import org.bidib.jbidibc.core.StringData;
import org.bidib.wizard.mvc.main.model.Node;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class NodeUtils {
private static final Logger LOGGER = LoggerFactory.getLogger(NodeUtils.class);
/**
* Find a node by its node uuid in the provided list of nodes.
*
* @param nodes
* the list of nodes
* @param uuid
* the uuid of the node to find
* @return the found node or null
if no node was found with the provided node uuid
*/
public static Node findNodeByUuid(Collection nodes, final long uuid) {
Node node = org.apache.commons.collections4.CollectionUtils.find(nodes, new Predicate() {
@Override
public boolean evaluate(final Node node) {
if (node.getUniqueId() == uuid) {
LOGGER.debug("Found node: {}", node);
return true;
}
return false;
}
});
return node;
}
/**
* Prepare the node label based on the user string with fallback to node.toString().
*
* @param node
* the node
* @return the node label
*/
public static String prepareLabel(Node node) {
String nodeLabel = null;
if (node != null) {
if (node.getNode().hasStoredStrings()) {
// the node has stored strings, prepare the label with the stored strings
String userString = node.getNode().getStoredString(StringData.INDEX_USERNAME);
if (StringUtils.isNotBlank(userString)) {
// user string is available
String label = node.toString();
if (label.startsWith(userString)) {
nodeLabel = label;
}
else {
nodeLabel = userString;
}
}
}
if (StringUtils.isBlank(nodeLabel)) {
nodeLabel = node.toString();
}
}
return nodeLabel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy