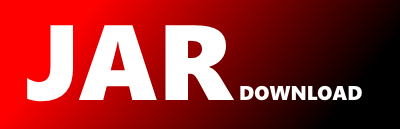
org.bidib.jbidibc.decoder.decoderdb.AbstractDecoderDbAccess Maven / Gradle / Ivy
The newest version!
package org.bidib.jbidibc.decoder.decoderdb;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.nio.charset.StandardCharsets;
import java.util.function.Function;
import org.apache.hc.client5.http.fluent.Content;
import org.apache.hc.client5.http.fluent.Request;
import org.apache.hc.core5.util.Timeout;
import org.bidib.jbidibc.decoder.exception.DecoderDbAccessException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.xml.JacksonXmlModule;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.datatype.jdk8.Jdk8Module;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.module.jaxb.JaxbAnnotationModule;
public abstract class AbstractDecoderDbAccess {
private static final Logger LOGGER = LoggerFactory.getLogger(AbstractDecoderDbAccess.class);
protected static final String REST_SERVICE_URI = "https://www.decoderdb.de";
public enum DataFormat {
json, xml;
}
/**
* Fetch the data from the decoderDB.
*
* @param login
* the login name
* @param password
* the password
* @param urlPath
* the path
* @return the fetched instance
* @throws URISyntaxException
* @throws MalformedURLException
*/
public static T fetch(String login, char[] password, String urlPath, Class responseType, DataFormat format) {
Request request = Request.get(urlPath).connectTimeout(Timeout.ofMilliseconds(5000));
// check if we have a proxy
ProxyUtils.getProxy(urlPath).ifPresent(proxy -> {
LOGGER.debug("Use proxy: {}", proxy);
request.viaProxy(proxy);
});
try {
final Content content = request.execute().returnContent();
String received = content.asString(StandardCharsets.UTF_8);
LOGGER.debug("Received: {}", received);
ObjectMapper objectMapper = null;
if (DataFormat.xml == format) {
final JacksonXmlModule xmlModule = new JacksonXmlModule();
xmlModule.setDefaultUseWrapper(false);
objectMapper = new XmlMapper(xmlModule);
objectMapper.registerModule(new JaxbAnnotationModule());
}
else {
objectMapper = new ObjectMapper().registerModule(new Jdk8Module()).registerModule(new JavaTimeModule());
}
T responseBody = objectMapper.readValue(received, responseType);
LOGGER.info("Retrieved responseBody: {}", responseBody);
return responseBody;
}
catch (Exception ex) {
LOGGER.warn("Fetch data from decoderDb failed.", ex);
throw new DecoderDbAccessException("Fetch data from decoderDb failed.", ex);
}
}
/**
* Fetch the data from the decoderDB.
*
* @param login
* the login name
* @param password
* the password
* @param urlPath
* the path
* @return the fetched instance
* @throws URISyntaxException
* @throws MalformedURLException
*/
public static T fetch(String login, char[] password, String urlPath, final Function mapper) {
Request request = Request.get(urlPath).connectTimeout(Timeout.ofMilliseconds(5000));
// check if we have a proxy
ProxyUtils.getProxy(urlPath).ifPresent(proxy -> {
LOGGER.debug("Use proxy: {}", proxy);
request.viaProxy(proxy);
});
try {
final Content content = request.execute().returnContent();
String received = content.asString(StandardCharsets.UTF_8);
LOGGER.debug("Received: {}", received);
T responseBody = mapper.apply(received);
LOGGER.info("Retrieved responseBody: {}", responseBody);
return responseBody;
}
catch (Exception ex) {
LOGGER.warn("Fetch data from decoderDb failed.", ex);
throw new DecoderDbAccessException("Fetch data from decoderDb failed.", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy