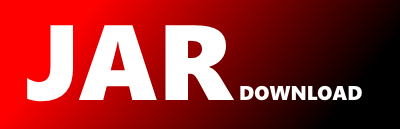
org.bidib.jbidibc.decoder.decoderdb.ProxyUtils Maven / Gradle / Ivy
The newest version!
package org.bidib.jbidibc.decoder.decoderdb;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Proxy;
import java.net.ProxySelector;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.UnknownHostException;
import java.util.Optional;
import org.apache.hc.core5.http.HttpHost;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.markusbernhardt.proxy.ProxySearch;
public class ProxyUtils {
private static final Logger LOGGER = LoggerFactory.getLogger(ProxyUtils.class);
public static Proxy findProxy(URI uri) throws URISyntaxException {
if (com.github.markusbernhardt.proxy.util.Logger.getBackend() == null) {
com.github.markusbernhardt.proxy.util.Logger.setBackend(new Slf4jLogBackEnd());
}
// Use the static factory method getDefaultProxySearch to create a proxy search instance
// configured with the default proxy search strategies for the current environment.
ProxySearch proxySearch = ProxySearch.getDefaultProxySearch();
// Invoke the proxy search. This will create a ProxySelector with the detected proxy settings.
ProxySelector proxySelector = proxySearch.getProxySelector();
if (proxySelector != null) {
// Install this ProxySelector as default ProxySelector for all connections.
ProxySelector.setDefault(proxySelector);
Proxy proxy = ProxySelector.getDefault().select(uri).iterator().next();
LOGGER.info("proxy type: {}", proxy.type());
InetSocketAddress addr = (InetSocketAddress) proxy.address();
if (addr == null) {
LOGGER.info("No Proxy");
}
else {
LOGGER.info("proxy hostname: {}", addr.getHostName());
LOGGER.info("proxy port: {}", addr.getPort());
return proxy;
}
}
else {
LOGGER.info("No proxy selector available.");
}
return Proxy.NO_PROXY;
}
public static Optional getProxy(String url) {
Proxy proxy = null;
try {
proxy = ProxyUtils.findProxy(new URL(url).toURI());
}
catch (Exception ex) {
LOGGER.warn("Find proxy failed.", ex);
}
if (!Proxy.NO_PROXY.equals(proxy)) {
try {
InetSocketAddress addr = (InetSocketAddress) proxy.address();
final String proxyUrl = addr.getHostName();
final int port = addr.getPort();
// check if the provided host is resolvable
try {
InetAddress.getByName(proxyUrl);
return Optional.of(new HttpHost(proxyUrl, port));
}
catch (UnknownHostException e) {
LOGGER.warn("The proxy host is not resolvable. Fallback to NO_PROXY.", e);
}
}
catch (Exception ex) {
LOGGER.warn("Prepare proxy HttpHost failed.", ex);
}
}
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy