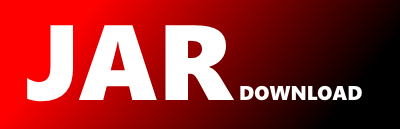
org.bidib.jbidibc.decoder.external.EsuXReader Maven / Gradle / Ivy
package org.bidib.jbidibc.decoder.external;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringReader;
import javax.xml.XMLConstants;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.UnmarshalException;
import javax.xml.bind.Unmarshaller;
import javax.xml.transform.Source;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import org.bidib.jbidibc.core.schema.exception.InvalidSchemaException;
import org.bidib.jbidibc.decoder.schema.decoder.DecoderDefinitionFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import eu.esu._2010.lokprogrammer.metadata.MetaType;
public class EsuXReader {
private static final Logger LOGGER = LoggerFactory.getLogger(EsuXReader.class);
private static JAXBContext jaxbContext;
private static final String JAXB_PACKAGE = "eu.esu._2010.lokprogrammer.metadata";
public static final String XSD_LOCATION = "/xsd/external/esuMetaData.xsd";
public static final String XSD_LANGUAGE_LOCATION = "/xsd/external/xml.xsd";
public String getMetaData(final InputStream esuxFile) throws FileNotFoundException, IOException {
final StringBuilder sb = new StringBuilder();
try (BufferedReader br = new BufferedReader(new InputStreamReader(esuxFile))) {
char[] prolog = new char[3];
if (br.read(prolog, 0, 3) == 3) {
// check the content
if (!String.valueOf(prolog).equals("ESU")) {
LOGGER.warn("The prolog is invalid. Expected 'ESU'.");
throw new IllegalArgumentException("The prolog is invalid. Expected 'ESU'.");
}
}
// search the start of the xml declaration
int last = -1;
while ((last = br.read()) != 0x3C && last != -1) {
}
if (last == 0x3C) {
LOGGER.info("Found the start of the xml declaration.");
sb.append("<");
String line = null;
do {
line = br.readLine();
LOGGER.info("Current line: {}", line);
int endIndex = -1;
if ((endIndex = line.indexOf("")) > 0) {
LOGGER.info("Found the end of the meta data at endIndex: {}", endIndex);
sb.append(line.substring(0, endIndex + "".length()));
break;
}
sb.append(line);
}
while (line != null);
}
else {
LOGGER.warn("No start of the xml declaration found.");
}
}
return sb.toString();
}
public MetaType getMetaType(final String source) {
MetaType meta = null;
try {
if (jaxbContext == null) {
LOGGER.info("Create the jaxb context for Meta.");
jaxbContext = JAXBContext.newInstance(JAXB_PACKAGE);
}
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
StreamSource streamSourceSchemaLanguage =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LANGUAGE_LOCATION));
StreamSource streamSourceSchema =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(new Source[] { streamSourceSchemaLanguage, streamSourceSchema });
unmarshaller.setSchema(schema);
JAXBElement metaElement = (JAXBElement) unmarshaller.unmarshal(new StringReader(source));
meta = metaElement.getValue();
}
catch (UnmarshalException ex) {
LOGGER.warn("Load Meta from provided source failed with UnmarshalException.", ex);
if (ex.getCause() instanceof SAXParseException) {
throw new InvalidSchemaException("Load Meta from provided source failed.");
}
throw new IllegalArgumentException("Load Meta from provided source failed with UnmarshalException.");
}
catch (JAXBException | SAXException ex) {
LOGGER.warn("Load Meta from provided source failed: {}", ex);
throw new InvalidSchemaException("Load Meta from provided source failed.");
}
catch (Exception ex) {
LOGGER.warn("Load Meta from provided source failed: {}", ex);
throw new RuntimeException("Load Meta from provided source failed.");
}
return meta;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy