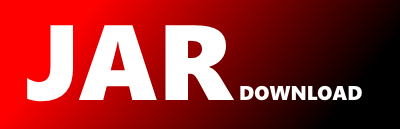
org.bidib.jbidibc.decoder.version.PatternCVersion Maven / Gradle / Ivy
package org.bidib.jbidibc.decoder.version;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This version is for T4T decoders.
*
*/
public class PatternCVersion extends AbstractDecoderVersion {
private static final Logger LOGGER = LoggerFactory.getLogger(PatternCVersion.class);
private static final String PATTERN_C_VERSION = "V([0-9]\\.?){1,2}";
private static final String PATTERN_C_REVISION = "R[0-9]+";
public static final String PATTERN_B = "[0-9]+";
private Integer minorVersion;
private Integer microVersion;
public PatternCVersion(String pattern) {
super(pattern);
// parsePattern(pattern);
}
@Override
public Integer getMinorVersion() {
return minorVersion;
}
@Override
public Integer getMicroVersion() {
return microVersion;
}
public Integer getRevision() {
return microVersion;
}
protected void setMinorVersion(Integer minorVersion) {
this.minorVersion = minorVersion;
}
protected void setMicroVersion(Integer microVersion) {
this.microVersion = microVersion;
}
public void parse(String versionString) {
parseVersion(versionString);
parseRevision(versionString);
}
protected void parseVersion(String versionString) {
Matcher m = Pattern.compile(PATTERN_C_VERSION).matcher(versionString);
while (m.find()) {
String text = m.group(0);
Matcher m1 = Pattern.compile(PATTERN_B).matcher(text);
int index = 0;
while (m1.find()) {
String textVersion = m1.group(0);
switch (index) {
case 0:
setMajorVersion(Integer.parseInt(textVersion));
break;
case 1:
setMinorVersion(Integer.parseInt(textVersion));
break;
default:
setMicroVersion(Integer.parseInt(textVersion));
break;
}
index++;
}
}
}
protected void parseRevision(String versionString) {
Matcher m = Pattern.compile(PATTERN_C_REVISION).matcher(versionString);
while (m.find()) {
String text = m.group(0);
Matcher m1 = Pattern.compile(PATTERN_B).matcher(text);
int index = 0;
while (m1.find()) {
String textVersion = m1.group(0);
switch (index) {
case 0:
setMicroVersion(Integer.parseInt(textVersion));
break;
default:
break;
}
index++;
}
}
}
private static final String PATTERN_C = "^V(\\{([0-9]){1,2}\\:M([0-9]|x){1,4}\\}\\.?){1,2}R\\{([0-9]){1,2}\\}$";
public static PatternCVersion parsePattern(String pattern) {
// must get some bits by mask
// ^V(\{([0-9]){1,2}\:M([0-9]|x){1,4}\}\.?){1,2}R\{([0-9]){1,2}\}$
// pattern to get the part of the version
final String versionPatternStart = "V(\\{([0-9]){1,2}\\:M([0-9]|x){1,4}\\}\\.?){1,2}";
final String versionPattern = "\\{([0-9]){1,2}\\:M([0-9]|x){1,4}\\}";
// pattern to get the part of the revision
final String revisionPatternStart = "R\\{([0-9]){1,2}\\}";
final String revisionPattern = "([0-9]){1,2}";
Matcher m = Pattern.compile(PATTERN_C).matcher(pattern);
if (m.matches()) {
LOGGER.info("The pattern matches! Current group: {}", m.group());
int groupCount = m.groupCount();
LOGGER.info("Current groupCount: {}", groupCount);
for (int i = 0; i < groupCount; i++) {
LOGGER.info("Current index: {}, group: {}", i, m.group(i));
}
Matcher versionStartMatcher = Pattern.compile(versionPatternStart).matcher(pattern);
if (versionStartMatcher.find()) {
String versionGroup = versionStartMatcher.group();
LOGGER.info("VersionStart, current group: {}", versionGroup);
int index = 0;
Matcher versionMatcher = Pattern.compile(versionPattern).matcher(versionGroup);
while (versionMatcher.find()) {
String currentGroup = versionMatcher.group();
LOGGER.info("Version, current group: {}, index: {}", currentGroup, index);
int groupCnt = versionMatcher.groupCount();
for (int group = 0; group < groupCnt; group++) {
LOGGER.info("Current group: {}", versionMatcher.group(group));
}
// parse the index of the value and the mask
index++;
}
}
Matcher revisionStartMatcher = Pattern.compile(revisionPatternStart).matcher(pattern);
if (revisionStartMatcher.find()) {
String currentRevisionGroup = revisionStartMatcher.group();
LOGGER.info("RevisionStart, current group: {}", currentRevisionGroup);
Matcher revisionMatcher = Pattern.compile(revisionPattern).matcher(currentRevisionGroup);
if (revisionMatcher.find()) {
String currentGroup = revisionMatcher.group();
LOGGER.info("Revision, current group: {}", currentGroup);
// Assert.assertEquals(currentGroup, "1");
}
}
}
else {
LOGGER.warn("The pattern does not match!");
}
return null;
}
@Override
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
result = prime * result + ((microVersion == null) ? 0 : microVersion.hashCode());
result = prime * result + ((minorVersion == null) ? 0 : minorVersion.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (!super.equals(obj))
return false;
if (getClass() != obj.getClass())
return false;
PatternCVersion other = (PatternCVersion) obj;
if (microVersion == null) {
if (other.microVersion != null)
return false;
}
else if (!microVersion.equals(other.microVersion))
return false;
if (minorVersion == null) {
if (other.minorVersion != null)
return false;
}
else if (!minorVersion.equals(other.minorVersion))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy