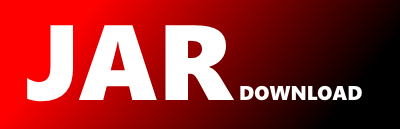
org.bidib.jbidibc.decoderdetection.DecoderDetectionFactory Maven / Gradle / Ivy
package org.bidib.jbidibc.decoderdetection;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.util.List;
import javax.xml.XMLConstants;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.UnmarshalException;
import javax.xml.bind.Unmarshaller;
import javax.xml.transform.Source;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.validation.Validator;
import org.apache.commons.lang3.StringUtils;
import org.bidib.jbidibc.core.schema.exception.InvalidContentException;
import org.bidib.jbidibc.core.schema.exception.InvalidSchemaException;
import org.bidib.jbidibc.core.schema.validation.XsdValidationLoggingErrorHandler;
import org.bidib.jbidibc.decoder.schema.decoder.DecoderDefinitionFactory;
import org.bidib.jbidibc.decoder.schema.decoderdetection.DecoderDetection;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
public class DecoderDetectionFactory {
private static final Logger LOGGER = LoggerFactory.getLogger(DecoderDetectionFactory.class);
private static JAXBContext jaxbContext;
private static final String JAXB_PACKAGE = "org.bidib.jbidibc.decoder.schema.decoderdetection";
public static final String XSD_LOCATION = "/xsd/decoderDetection.xsd";
public static final String XSD_LOCATION_COMMON_TYPES = "/xsd/commonTypes.xsd";
public DecoderDetection parseDecoderDetection(String content) {
if (StringUtils.isBlank(content)) {
LOGGER.error("The DecoderDetection value is empty!");
return null;
}
LOGGER.info("Load DecoderDetection from provided string value.");
DecoderDetection decoderDetection = null;
try (ByteArrayInputStream is = new ByteArrayInputStream(content.getBytes(StandardCharsets.UTF_8))) {
decoderDetection = loadDecoderDetectionFile(is);
LOGGER.trace("Loaded decoderDetection: {}", decoderDetection);
}
catch (InvalidSchemaException ex) {
LOGGER.warn("Load saved DecoderDetection from file failed with schema exception: {}", ex.getMessage());
try (ByteArrayInputStream fis = new ByteArrayInputStream(content.getBytes(StandardCharsets.UTF_8))) {
List errors = validateDecoderDetectionFile(fis, null);
// LOGGER.warn("The following validation errors were detected: {}", errors);
throw new InvalidContentException("Validation of decoderDetection from string content failed.", errors);
}
catch (IOException e) {
LOGGER.warn("Load saved DecoderDetection for validation from file failed: {}", e.getMessage());
}
}
catch (InvalidContentException ex) {
// rethrow exception
throw ex;
}
catch (Exception ex) {
LOGGER.warn("Load saved DecoderDetection from string content failed: {}", ex.getMessage());
}
return decoderDetection;
}
public DecoderDetection loadDecoderDetection(String filePath) {
if (StringUtils.isBlank(filePath)) {
LOGGER.error("The DecoderDetection file must be specified!");
return null;
}
LOGGER.info("Load DecoderDetection from file: {}", filePath);
DecoderDetection decoderDetection = null;
FileInputStream is = null;
File file = null;
try {
file = new File(filePath);
is = new FileInputStream(file);
decoderDetection = loadDecoderDetectionFile(is);
LOGGER.trace("Loaded decoderDetection: {}", decoderDetection);
}
catch (InvalidSchemaException ex) {
LOGGER.warn("Load saved DecoderDetection from file failed with schema exception: {}", ex.getMessage());
if (is != null) {
try {
is.close();
}
catch (IOException e) {
LOGGER.warn("Close input stream failed.", e);
}
is = null;
}
if (file != null) {
try (FileInputStream fis = new FileInputStream(file)) {
List errors = validateDecoderDetectionFile(fis, filePath);
// LOGGER.warn("The following validation errors were detected: {}", errors);
throw new InvalidContentException("Validation of DecoderDetection file failed: " + filePath,
errors);
}
catch (IOException e) {
LOGGER.warn("Load saved DecoderDetection for validation from file failed: {}", e.getMessage());
}
}
}
catch (IllegalArgumentException | FileNotFoundException ex) {
LOGGER.warn("Load saved DecoderDetection from file failed: {}", ex.getMessage());
}
catch (InvalidContentException ex) {
// rethrow exception
throw ex;
}
catch (Exception ex) {
LOGGER.warn("Load saved DecoderDetection from file failed: {}", ex.getMessage());
}
return decoderDetection;
}
protected String getDecoderDetectionFileName() {
// TODO change it
return "test";
}
/**
* Validate the decoder detection from the provided input stream.
*
* @param is
* the input stream
* @param filePath
* the file path
* @return the list of errors
*/
private List validateDecoderDetectionFile(final InputStream is, String filePath) {
LOGGER.info("Validate decoderDetection file: {}", filePath);
List errors = null;
try {
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
// Schema schema =
// schemaFactory.newSchema(new File(DecoderDefinitionFactory.class.getResource(XSD_LOCATION).getPath()));
StreamSource streamSourceSchemaCommonTypes =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LOCATION_COMMON_TYPES));
StreamSource streamSourceSchema =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(new Source[] { streamSourceSchemaCommonTypes, streamSourceSchema });
StreamSource streamSource = new StreamSource(is);
Validator validator = schema.newValidator();
XsdValidationLoggingErrorHandler errorHandler = new XsdValidationLoggingErrorHandler();
validator.setErrorHandler(errorHandler);
validator.validate(streamSource);
errors = errorHandler.getErrors();
}
catch (Exception ex) {
LOGGER.warn("Validate DecoderDetection from file failed: {}", getDecoderDetectionFileName(), ex);
throw new InvalidSchemaException("Load DecoderDetection from file failed.");
}
return errors;
}
/**
* Load the decoder detection from the provided input stream.
*
* @param is
* the input stream
* @return the decoder defintion
*/
private DecoderDetection loadDecoderDetectionFile(final InputStream is) {
LOGGER.info("Load DecoderDetection file.");
DecoderDetection decoderDetection = null;
try {
if (jaxbContext == null) {
LOGGER.info("Create the jaxb context for DecoderDetection.");
jaxbContext = JAXBContext.newInstance(JAXB_PACKAGE);
}
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
StreamSource streamSourceSchemaCommonTypes =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LOCATION_COMMON_TYPES));
StreamSource streamSourceSchema =
new StreamSource(DecoderDefinitionFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(new Source[] { streamSourceSchemaCommonTypes, streamSourceSchema });
// Schema schema =
// schemaFactory.newSchema(new File(DecoderDefinitionFactory.class.getResource(XSD_LOCATION).getPath()));
unmarshaller.setSchema(schema);
decoderDetection = (DecoderDetection) unmarshaller.unmarshal(is);
}
catch (UnmarshalException ex) {
LOGGER.warn("Load DecoderDetection from file failed with UnmarshalException.", ex);
if (ex.getCause() instanceof SAXParseException) {
throw new InvalidSchemaException("Load DecoderDetection from file failed");
}
throw new IllegalArgumentException("Load DecoderDetection from file failed with UnmarshalException.");
}
catch (JAXBException | SAXException ex) {
LOGGER.warn("Load DecoderDetection from file failed: {}", getDecoderDetectionFileName(), ex);
throw new InvalidSchemaException(
"Load DecoderDetection from file failed: " + getDecoderDetectionFileName());
}
catch (Exception ex) {
LOGGER.warn("Load DecoderDetection from file failed: {}", getDecoderDetectionFileName(), ex);
throw new RuntimeException("Load DecoderDetection from file failed: " + getDecoderDetectionFileName());
}
return decoderDetection;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy