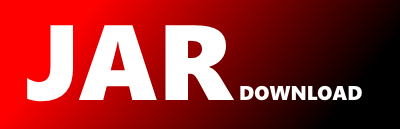
org.bidib.jbidibc.exchange.firmwarerepo.FirmwareRepoFactory Maven / Gradle / Ivy
package org.bidib.jbidibc.exchange.firmwarerepo;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import javax.xml.XMLConstants;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.XMLStreamReader;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import org.bidib.jbidibc.exchange.bidib.FirmwareFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xml.sax.SAXException;
public class FirmwareRepoFactory {
private static final Logger LOGGER = LoggerFactory.getLogger(FirmwareRepoFactory.class);
private static final String JAXB_PACKAGE = "org.bidib.jbidibc.exchange.firmwarerepo";
private static final String XSD_LOCATION = "/xsd/firmware-repo.xsd";
protected FirmwareRepoFactory() {
}
public static FirmwareRepoType getFirmwareRepo(String path) {
LOGGER.info("Load the Firmware Repo info from path: {}", path);
return new FirmwareRepoFactory().loadFirmwareRepo(path);
}
public static FirmwareRepoType getFirmwareRepo(StringBuffer definition) {
LOGGER.info("Load the Firmware Repo info from definition: {}", definition);
return new FirmwareRepoFactory().loadFirmwareRepo(definition);
}
protected FirmwareRepoType loadFirmwareRepo(StringBuffer definition) {
LOGGER.info("Load the firmware repo definition: {}", definition);
ByteArrayInputStream bais = new ByteArrayInputStream(definition.toString().getBytes());
FirmwareRepoType firmwareRepo = loadFirmwareRepoFile(bais);
return firmwareRepo;
}
protected FirmwareRepoType loadFirmwareRepo(String path) {
LOGGER.info("Load the firmware definition, path: {}", path);
FirmwareRepoType firmwareRepo = null;
File firmwareFile = new File(path);
if (firmwareFile.exists()) {
LOGGER.info("Found firmware file: {}", firmwareFile.getAbsolutePath());
// try to load products
firmwareRepo = loadFirmwareRepoFile(firmwareFile);
}
else {
LOGGER.info("File does not exist: {}", firmwareFile.getAbsolutePath());
}
return firmwareRepo;
}
private FirmwareRepoType loadFirmwareRepoFile(File firmwareRepoFile) {
FirmwareRepoType firmwareRepo = null;
InputStream is = null;
try {
is = new FileInputStream(firmwareRepoFile);
firmwareRepo = loadFirmwareRepoFile(is);
}
catch (FileNotFoundException ex) {
LOGGER.info("No firmware repo file found.");
}
return firmwareRepo;
}
private FirmwareRepoType loadFirmwareRepoFile(InputStream is) {
FirmwareRepoType firmwareRepo = null;
try {
JAXBContext jaxbContext = JAXBContext.newInstance(JAXB_PACKAGE);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
SchemaFactory schemaFactory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
StreamSource streamSource = new StreamSource(FirmwareFactory.class.getResourceAsStream(XSD_LOCATION));
Schema schema = schemaFactory.newSchema(streamSource);
unmarshaller.setSchema(schema);
XMLInputFactory factory = XMLInputFactory.newInstance();
XMLStreamReader xmlr = factory.createXMLStreamReader(is);
JAXBElement jaxbElement =
(JAXBElement) unmarshaller.unmarshal(xmlr, FirmwareRepoType.class);
firmwareRepo = jaxbElement.getValue();
}
catch (JAXBException | XMLStreamException | SAXException ex) {
LOGGER.warn("Load firmware repo from file failed.", ex);
}
return firmwareRepo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy