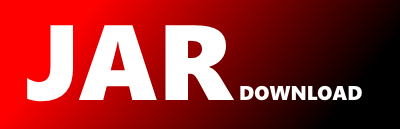
org.bidib.jbidibc.BoostQuery Maven / Gradle / Ivy
package org.bidib.jbidibc;
import org.bidib.jbidibc.core.BidibMessageProcessor;
import org.bidib.jbidibc.core.DefaultMessageListener;
import org.bidib.jbidibc.core.node.BoosterNode;
import org.bidib.jbidibc.messages.Node;
import org.bidib.jbidibc.messages.enums.BoosterControl;
import org.bidib.jbidibc.messages.enums.BoosterState;
import org.bidib.jbidibc.messages.exception.PortNotFoundException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import picocli.CommandLine.Command;
/**
* This commands reads the value of the specified CV from the specified node.
*
*/
@Command(separator = "=")
public class BoostQuery extends BidibNodeCommand {
private static final Logger LOGGER = LoggerFactory.getLogger(BoostQuery.class);
public static void main(String[] args) {
run(new BoostQuery(), args);
}
@Override
public Integer call() {
int result = 20;
try {
openPort(getPortName(), null);
Node node = findNode();
if (node != null) {
final BoosterStateHelper boosterStateHelper = new BoosterStateHelper();
final BidibMessageProcessor bidibMessageProcessor = getBidib().getBidibMessageProcessor();
if (bidibMessageProcessor != null) {
bidibMessageProcessor.addMessageListener(new DefaultMessageListener() {
@Override
public void boosterState(
byte[] address, int messageNum, BoosterState state, BoosterControl control) {
LOGGER.info("Received current booster state: {}, control: {}", state, control);
boosterStateHelper.setBoosterState(state);
boosterStateHelper.setBoosterControl(control);
synchronized (boosterStateHelper) {
boosterStateHelper.notifyAll();
}
}
});
}
// verify that the booster query returns the booster state ...
BoosterNode boosterNode = getBidib().getBoosterNode(node);
boosterNode.boosterQuery();
// wait for repsonse
synchronized (boosterStateHelper) {
LOGGER.info("Wait for response.");
if (boosterStateHelper.getBoosterState() == null) {
boosterStateHelper.wait(3000L);
}
}
LOGGER
.info("Current booster state: {}, booster control: {}", boosterStateHelper.getBoosterState(),
boosterStateHelper.getBoosterControl());
result = 0;
}
else {
LOGGER.warn("node with unique id \"" + getNodeIdentifier() + "\" not found");
}
getBidib().close();
}
catch (PortNotFoundException ex) {
LOGGER
.error("The provided port was not found: " + ex.getMessage()
+ ". Verify that the BiDiB device is connected.", ex);
}
catch (Exception ex) {
LOGGER.error("Execute command failed.", ex);
}
return result;
}
private static final class BoosterStateHelper {
private BoosterState boosterState;
private BoosterControl boosterControl;
private Object lock = new Object();
public void setBoosterState(BoosterState boosterState) {
synchronized (lock) {
this.boosterState = boosterState;
}
}
public BoosterState getBoosterState() {
synchronized (lock) {
return boosterState;
}
}
/**
* @return the boosterControl
*/
public BoosterControl getBoosterControl() {
return boosterControl;
}
/**
* @param boosterControl
* the boosterControl to set
*/
public void setBoosterControl(BoosterControl boosterControl) {
this.boosterControl = boosterControl;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy