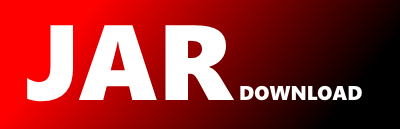
org.bidib.jbidibc.BulkReadCv Maven / Gradle / Ivy
package org.bidib.jbidibc;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.bidib.jbidibc.core.node.BidibNode;
import org.bidib.jbidibc.messages.Node;
import org.bidib.jbidibc.messages.VendorData;
import org.bidib.jbidibc.messages.VendorGetData;
import org.bidib.jbidibc.messages.exception.PortNotFoundException;
import picocli.CommandLine.Command;
import picocli.CommandLine.Option;
/**
* This commands reads the value of the specified CV from the specified node.
*
*/
@Command
public class BulkReadCv extends BidibNodeCommand {
@Option(names = { "-cv" }, description = "The CV numbers, separated with ','", required = true)
private String cvNumbers;
public static void main(String[] args) {
run(new BulkReadCv(), args);
}
@Override
public Integer call() {
int result = 20;
if (StringUtils.isBlank(cvNumbers)) {
System.err.println("No cvNumbers provided!"); // NOSONAR
return -1;
}
try {
openPort(getPortName(), null);
Node node = findNode();
if (node != null) {
BidibNode bidibNode = getBidib().getNode(node);
if (bidibNode.vendorEnable(node.getUniqueId())) {
final List vendorGetDatas = new ArrayList<>();
for (String cvNumber : Arrays.asList(StringUtils.splitPreserveAllTokens(cvNumbers, ","))) {
vendorGetDatas.add(new VendorGetData(cvNumber, false));
}
final List vendorDataList = new LinkedList<>();
bidibNode.vendorGetBulk(vendorGetDatas, vendorDataList, null);
if (CollectionUtils.isNotEmpty(vendorDataList)) {
for (VendorData vendorData : vendorDataList) {
System.out.println("CV" + vendorData.getName() + "=" + vendorData.getValue()); // NOSONAR
}
}
bidibNode.vendorDisable();
result = 0;
}
}
else {
System.err.println("node with unique id \"" + getNodeIdentifier() + "\" not found"); // NOSONAR
}
getBidib().close();
}
catch (PortNotFoundException ex) {
System.err
.println("The provided port was not found: " + ex.getMessage() // NOSONAR
+ ". Verify that the BiDiB device is connected.");
}
catch (Exception ex) {
System.err.println("Execute command failed: " + ex); // NOSONAR
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy