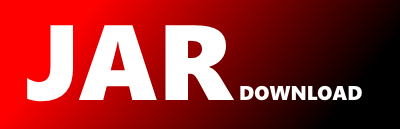
org.bidib.jbidibc.GetMacros Maven / Gradle / Ivy
package org.bidib.jbidibc;
import org.bidib.jbidibc.core.node.AccessoryNode;
import org.bidib.jbidibc.messages.BidibLibrary;
import org.bidib.jbidibc.messages.Feature;
import org.bidib.jbidibc.messages.LcMacro;
import org.bidib.jbidibc.messages.Node;
import org.bidib.jbidibc.messages.enums.LcOutputType;
import org.bidib.jbidibc.messages.enums.PortModelEnum;
import org.bidib.jbidibc.messages.exception.PortNotFoundException;
import picocli.CommandLine.Command;
/**
* This commands gets the list of macros from the specified node.
*
*/
@Command
public class GetMacros extends BidibNodeCommand {
public static void main(String[] args) {
run(new GetMacros(), args);
}
@Override
public Integer call() {
int result = 20;
try {
openPort(getPortName(), null);
Node node = findNode();
if (node != null) {
AccessoryNode accessoryNode = getBidib().getAccessoryNode(node);
if (accessoryNode != null) {
Feature feature = accessoryNode.getBidibNode().getFeature(BidibLibrary.FEATURE_CTRL_MAC_COUNT);
int macroCount = feature != null ? feature.getValue() : 0;
feature = accessoryNode.getBidibNode().getFeature(BidibLibrary.FEATURE_CTRL_MAC_SIZE);
int macroLength = feature != null ? feature.getValue() : 0;
for (int macroNumber = 0; macroNumber < macroCount; macroNumber++) {
int stepNumber = 0;
System.out.println("Macro " + macroNumber + ":"); // NOSONAR
System.out
.println("\tcycles: " // NOSONAR
+ accessoryNode.getMacroParameter(macroNumber, BidibLibrary.BIDIB_MACRO_PARA_REPEAT));
System.out
.println("\tspeed: " // NOSONAR
+ accessoryNode.getMacroParameter(macroNumber, BidibLibrary.BIDIB_MACRO_PARA_SLOWDOWN));
System.out.println("\tsteps:"); // NOSONAR
for (;;) {
final LcMacro macroStep = accessoryNode.getMacroStep(macroNumber, stepNumber++);
if (macroStep.getBidibPort().getPortType(PortModelEnum.type) == LcOutputType.END_OF_MACRO
|| stepNumber > macroLength) {
break;
}
System.out.println("\t\t" + stepNumber + ". " + macroStep); // NOSONAR
}
}
result = 0;
}
else {
System.err.println("node with unique id \"" + getNodeIdentifier() + "\" doesn't have macros"); // NOSONAR
}
}
else {
System.err.println("node with unique id \"" + getNodeIdentifier() + "\" not found"); // NOSONAR
}
getBidib().close();
}
catch (PortNotFoundException ex) {
System.err
.println("The provided port was not found: " + ex.getMessage() // NOSONAR
+ ". Verify that the BiDiB device is connected.");
}
catch (Exception ex) {
System.err.println("Execute command failed: " + ex); // NOSONAR
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy