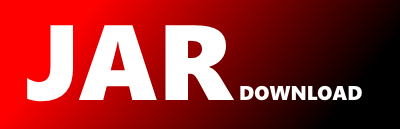
org.bidib.jbidibc.usbhid.UsbDevice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbidibc-usb Show documentation
Show all versions of jbidibc-usb Show documentation
jBiDiB jbidibc USB POM
Install the libusb drivers for using the libusb access. Under Windows OS you must install the Zadig driver as described here: https://github.com/libusb/libusb/wiki/Windows.
Zadic can be downloaded from: http://zadig.akeo.ie/
In the Zadig UI you must check Options > List all devices and the select the 'USB-EPP /I2C... CH341A' device. Then select the target driver with the spinners (I used libusb-win32 (v1.2.6.0))
and click the 'Install driver' button.
The newest version!
package org.bidib.jbidibc.usbhid;
import org.usb4java.DeviceHandle;
public class UsbDevice {
private DeviceHandle handle;
private final int vendorId;
private final int productId;
private final String deviceName;
// private final String mName;
private final String mManufacturerName;
private final String mProductName;
private final String mSerialNumber;
// private final String mVersion;
// private boolean detachKernelDriver;
private UsbInterface[] mInterfaces;
private final UsbConfiguration[] mConfigurations;
public UsbDevice(int vendorId, int productId, String deviceName, UsbConfiguration[] configurations,
String mManufacturerName, String mProductName, String mSerialNumber) {
this.vendorId = vendorId;
this.productId = productId;
this.deviceName = deviceName;
this.mConfigurations = configurations;
this.mManufacturerName = mManufacturerName;
this.mProductName = mProductName;
this.mSerialNumber = mSerialNumber;
}
public int getVendorId() {
return this.vendorId;
}
public int getProductId() {
return this.productId;
}
public String getDeviceName() {
return this.deviceName;
}
public String getManufacturerName() {
return this.mManufacturerName;
}
public String getProductName() {
return this.mProductName;
}
public String getSerialNumberName() {
return this.mSerialNumber;
}
private UsbInterface[] getInterfaceList() {
if (mInterfaces == null) {
int configurationCount = mConfigurations.length;
int interfaceCount = 0;
for (int i = 0; i < configurationCount; i++) {
UsbConfiguration configuration = mConfigurations[i];
interfaceCount += configuration.getInterfaceCount();
}
mInterfaces = new UsbInterface[interfaceCount];
int offset = 0;
for (int i = 0; i < configurationCount; i++) {
UsbConfiguration configuration = mConfigurations[i];
interfaceCount = configuration.getInterfaceCount();
for (int j = 0; j < interfaceCount; j++) {
mInterfaces[offset++] = configuration.getInterface(j);
}
}
}
return mInterfaces;
}
public int getInterfaceCount() {
return getInterfaceList().length;
}
public UsbInterface getInterface(int index) {
return getInterfaceList()[index];
}
public void setHandle(final DeviceHandle handle) {
this.handle = handle;
}
public DeviceHandle getHandle() {
return this.handle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy