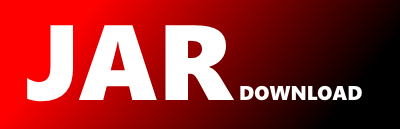
org.bidib.jbidibc.usbhid.UsbInterface Maven / Gradle / Ivy
package org.bidib.jbidibc.usbhid;
public class UsbInterface {
private final int mId;
private final int mAlternateSetting;
private final String mName;
private final int mClass;
private final int mSubclass;
private final int mProtocol;
/** All endpoints of this interface, only null during creation */
private UsbEndpoint[] mEndpoints;
/**
* UsbInterface should only be instantiated by UsbService implementation
*
* @hide
*/
public UsbInterface(int id, int alternateSetting, String name, int intClass, int subClass, int protocol) {
mId = id;
mAlternateSetting = alternateSetting;
mName = name;
mClass = intClass;
mSubclass = subClass;
mProtocol = protocol;
}
/**
* Returns the interface's bInterfaceNumber field. This is an integer that along with the alternate setting uniquely
* identifies the interface on the device.
*
* @return the interface's ID
*/
public int getId() {
return mId;
}
/**
* Returns the interface's bAlternateSetting field. This is an integer that along with the ID uniquely identifies
* the interface on the device. {@link UsbDeviceConnection#setInterface} can be used to switch between two
* interfaces with the same ID but different alternate setting.
*
* @return the interface's alternate setting
*/
public int getAlternateSetting() {
return mAlternateSetting;
}
/**
* Returns the interface's name.
*
* @return the interface's name, or {@code null} if the property could not be read
*/
public String getName() {
return mName;
}
/**
* Returns the interface's class field. Some useful constants for USB classes can be found in {@link UsbConstants}
*
* @return the interface's class
*/
public int getInterfaceClass() {
return mClass;
}
/**
* Returns the interface's subclass field.
*
* @return the interface's subclass
*/
public int getInterfaceSubclass() {
return mSubclass;
}
/**
* Returns the interface's protocol field.
*
* @return the interface's protocol
*/
public int getInterfaceProtocol() {
return mProtocol;
}
/**
* Returns the number of {@link android.hardware.usb.UsbEndpoint}s this interface contains.
*
* @return the number of endpoints
*/
public int getEndpointCount() {
return mEndpoints.length;
}
/**
* Returns the {@link android.hardware.usb.UsbEndpoint} at the given index.
*
* @return the endpoint
*/
public UsbEndpoint getEndpoint(int index) {
return mEndpoints[index];
}
/**
* Only used by UsbService implementation
*
* @hide
*/
public void setEndpoints(UsbEndpoint[] endpoints) {
mEndpoints = endpoints;
}
@Override
public String toString() {
StringBuilder builder =
new StringBuilder("UsbInterface[mId=" + mId + ",mAlternateSetting=" + mAlternateSetting + ",mName=" + mName
+ ",mClass=" + mClass + ",mSubclass=" + mSubclass + ",mProtocol=" + mProtocol + ",mEndpoints=[");
for (int i = 0; i < mEndpoints.length; i++) {
builder.append("\n");
builder.append(mEndpoints[i].toString());
}
builder.append("]");
return builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy