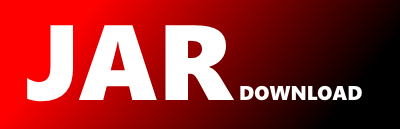
org.bidib.jbidibc.usbstickbasis.adapter.SelectedCar Maven / Gradle / Ivy
package org.bidib.jbidibc.usbstickbasis.adapter;
import java.util.HashMap;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jgoodies.binding.beans.Model;
public class SelectedCar extends Model {
private static final Logger LOGGER = LoggerFactory.getLogger(SelectedCar.class);
private static final long serialVersionUID = 1L;
public static final String PROPERTYNAME_DECODERADDRESS = "decoderAddress";
public static final String PROPERTYNAME_SCALE = "scale";
public static final String PROPERTYNAME_FUNCTIONMAP = "functionMap";
public static final String PROPERTYNAME_FUNCTIONVALUE = "functionValue";
private Integer decoderAddress;
private Integer scale;
private Map functionMap = new HashMap<>();
public SelectedCar() {
}
/**
* @return the decoderAddress
*/
public Integer getDecoderAddress() {
return decoderAddress;
}
/**
* @param decoderAddress
* the decoderAddress to set
*/
public void setDecoderAddress(Integer decoderAddress) {
Integer oldValue = this.decoderAddress;
this.decoderAddress = decoderAddress;
firePropertyChange(PROPERTYNAME_DECODERADDRESS, oldValue, decoderAddress);
if (oldValue != decoderAddress) {
LOGGER.info("The decoder address has changed. Clear the function map.");
functionMap.clear();
}
}
/**
* @return the scale
*/
public Integer getScale() {
return scale;
}
/**
* @param scale
* the scale to set
*/
public void setScale(Integer scale) {
Integer oldValue = this.scale;
this.scale = scale;
firePropertyChange(PROPERTYNAME_SCALE, oldValue, scale);
}
/**
* @return the functionMap
*/
public Map getFunctionMap() {
return functionMap;
}
/**
* @param functionMap
* the functionMap to set
*/
public void setFunctionMap(Map functionMap) {
this.functionMap = functionMap;
}
/**
* Get the value of the function from the cache.
*
* @param functionAddress
* the function address
* @return the value, or {@code null} if not available
*/
public Integer getFunctionValue(Integer functionAddress) {
return functionMap.get(functionAddress);
}
/**
* Set the function value in the cache.
*
* @param functionAddress
* the function address
* @param functionValue
* the new value
*/
public void setFunctionValue(Integer functionAddress, Integer functionValue) {
if (functionValue != null) {
functionMap.put(functionAddress, functionValue);
}
else {
functionMap.remove(functionAddress);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy