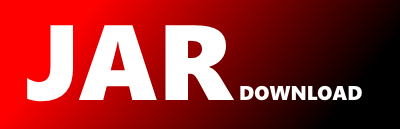
org.bigml.mimir.image.featurize.CachingFeaturizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mimir Show documentation
Show all versions of mimir Show documentation
Java/Clojure Prediction Code for BigML
The newest version!
package org.bigml.mimir.image.featurize;
import java.awt.image.BufferedImage;
import java.util.Deque;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.Map;
/**
* A wrapper class for an ImageFeaturizer that caches the values for the last
* several images seen, returning them if present in the rudimentary cache. Note
* that the cache relies on the hashcode
of the input value to
* determine if the features for the image are in the cache, and is thus
* vunerable to collision based adversarial attacks.
*
* @author [email protected]
*
*/
public class CachingFeaturizer extends ImageFeaturizer {
public CachingFeaturizer(ImageFeaturizer featurizer) {
_featurizer = featurizer;
_cache = new Cache();
};
@Override
public int numberOfFeatures() {
return _featurizer.numberOfFeatures();
}
@Override
protected String[] featureNames() {
return _featurizer.featureNames();
}
@Override
protected double[] featuresFromImage(BufferedImage image) {
double[] features = _cache.lookup(image);
if (features == null) {
features = _featurizer.featuresFromImage(image);
_cache.add(image, features);
}
return features;
}
private class Cache {
private Cache() {
_cacheMap = new HashMap
© 2015 - 2024 Weber Informatics LLC | Privacy Policy