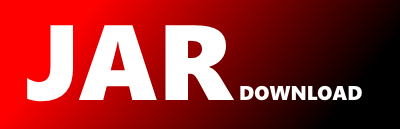
org.bigml.mimir.utils.Json Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mimir Show documentation
Show all versions of mimir Show documentation
Java/Clojure Prediction Code for BigML
The newest version!
package org.bigml.mimir.utils;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.NullNode;
/**
* Utility methods for parsing JSON resources
*
* @author [email protected]
*
*/
public class Json {
public static final NullNode NULL = JsonNodeFactory.instance.nullNode();
public static JsonNode parseObject(String object) {
ObjectMapper om = new ObjectMapper();
try {
return om.readTree(object);
}
catch (IOException e) {
throw new RuntimeException(e);
}
}
public static JsonNode parseStream(InputStream stream) {
ObjectMapper om = new ObjectMapper();
try {
return om.readTree(stream);
}
catch (IOException e) {
throw new RuntimeException(e);
}
}
public static JsonNode readObject(String path) {
try (InputStream is =
ResourceLoader.streamForFile(path)) {
return parseStream(is);
}
catch (IOException e) {
throw new RuntimeException(e);
}
}
public static JsonNode readObject(URL u) {
try {
return parseStream(u.openStream());
}
catch (Exception e) {
throw new RuntimeException(e);
}
}
public static double[] get1DArray(JsonNode node) {
if (node == null || node.isNull()) return null;
double[] array = new double[node.size()];
for (int i = 0; i < array.length; i++)
array[i] = node.get(i).asDouble();
return array;
}
public static double[][] get2DArray(JsonNode node) {
if (node == null || node.isNull()) return null;
double[][] array = new double[node.size()][];
for (int i = 0; i < array.length; i++)
array[i] = get1DArray(node.get(i));
return array;
}
public static double[][][] get3DArray(JsonNode node) {
if (node == null || node.isNull()) return null;
double[][][] array = new double[node.size()][][];
for (int i = 0; i < array.length; i++)
array[i] = get2DArray(node.get(i));
return array;
}
public static double[][][][] get4DArray(JsonNode node) {
if (node == null || node.isNull()) return null;
double[][][][] array = new double[node.size()][][][];
for (int i = 0; i < array.length; i++)
array[i] = get3DArray(node.get(i));
return array;
}
public static int[] get1DIntArray(JsonNode node) {
if (node == null || node.isNull()) return null;
int[] array = new int[node.size()];
for (int i = 0; i < array.length; i++)
array[i] = node.get(i).asInt();
return array;
}
public static int[][] get2DIntArray(JsonNode node) {
if (node == null || node.isNull()) return null;
int[][] array = new int[node.size()][];
for (int i = 0; i < array.length; i++)
array[i] = get1DIntArray(node.get(i));
return array;
}
public static long[] get1DLongArray(JsonNode node) {
if (node == null || node.isNull()) return null;
long[] array = new long[node.size()];
for (int i = 0; i < array.length; i++)
array[i] = node.get(i).asLong();
return array;
}
public static long[][] get2DLongArray(JsonNode node) {
if (node == null || node.isNull()) return null;
long[][] array = new long[node.size()][];
for (int i = 0; i < array.length; i++)
array[i] = get1DLongArray(node.get(i));
return array;
}
public static String[] getStringArray(JsonNode node) {
if (node == null || node.isNull()) return null;
String[] array = new String[node.size()];
for (int i = 0; i < array.length; i++)
array[i] = node.get(i).asText();
return array;
}
public static double getDoubleOrNaN(JsonNode node, String key) {
if (node.has(key) && !node.get(key).isNull())
return node.get(key).asDouble();
else
return Double.NaN;
}
public static String getString(JsonNode node, String key, String dVal) {
if (node.has(key))
return node.get(key).asText();
else return dVal;
}
public static boolean getBoolean(JsonNode node, String key, boolean dVal) {
if (node.has("key")) return node.get("key").asBoolean();
else return dVal;
}
public static List keys(JsonNode node) {
List strs = new ArrayList();
Iterator ks = node.fieldNames();
while (ks.hasNext()) strs.add(ks.next());
Collections.sort(strs);
return strs;
}
public static Object nodeToValue(JsonNode node) {
if (node.isInt()) return node.asInt();
else if (node.isNumber()) return node.asDouble();
else if (node.isNull()) return null;
else if (node.isTextual()) return node.asText();
else throw new RuntimeException("Value is: " + node.toString());
}
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy