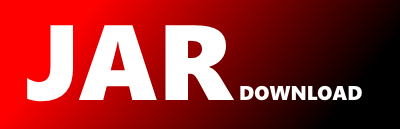
org.biojava.nbio.protmod.ProteinModificationRegistry Maven / Gradle / Ivy
/*
* BioJava development code
*
* This code may be freely distributed and modified under the
* terms of the GNU Lesser General Public Licence. This should
* be distributed with the code. If you do not have a copy,
* see:
*
* http://www.gnu.org/copyleft/lesser.html
*
* Copyright for this code is held jointly by the individual
* authors. These should be listed in @author doc comments.
*
* For more information on the BioJava project and its aims,
* or to join the biojava-l mailing list, visit the home page
* at:
*
* http://www.biojava.org/
*
* Created on Nov 17, 2010
* Author: Jianjiong Gao
*
*/
package org.biojava.nbio.protmod;
import org.biojava.nbio.protmod.io.ProteinModificationXmlReader;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.InputStream;
import java.util.*;
/**
* This class serves as a instance registry by maintaining
* a pool of ProteinModification instances.
*
* A list of common protein modifications were preloaded
* from an XML file.
*
* @author Jianjiong Gao
* @since 3.0
*/
public class ProteinModificationRegistry {
private static final Logger logger = LoggerFactory.getLogger(ProteinModificationRegistry.class);
private static Set registry = null;
private static Map byId = null;
private static Map> byResidId = null;
private static Map> byPsimodId = null;
private static Map> byPdbccId = null;
private static Map> byKeyword = null;
private static Map> byComponent = null;
private static Map> byCategory = null;
private static Map> byOccurrenceType = null;
private static String DIR_XML_PTM_LIST = "ptm_list.xml";
/**
* register common protein modifications from XML file.
*/
private static void registerCommonProteinModifications(InputStream inStream) {
try {
ProteinModificationXmlReader.registerProteinModificationFromXml(inStream);
} catch (Exception e) {
logger.error("Exception: ", e);
}
}
/**
* Initialization the static variables and register common modifications.
*/
public static void init() {
lazyInit();
}
/** Initialization the static variables and register common modifications.
* Allows external user to provide alternative ptm_list.xml file instead of the one contained in this jar file.
*
* @param inStream InputStream to a XML file containing the list of PTMs (as in ptm_list.xml)
*/
public static void init(InputStream inStream) {
lazyInit(inStream);
}
/**
* Lazy Initialization the static variables and register common modifications.
* just opens the stream to ptm_list.xml and delegates to lazyInit(InputStream) for parsing.
*/
private static synchronized void lazyInit() {
if (registry==null) {
InputStream isXml = ProteinModification.class.getResourceAsStream(DIR_XML_PTM_LIST);
lazyInit(isXml);
}
}
/**
* Lazy Initialization the static variables and register common modifications.
*/
private static synchronized void lazyInit(InputStream inStream) {
if (registry==null) {
registry = new HashSet();
byId = new HashMap();
byResidId = new HashMap>();
byPsimodId = new HashMap>();
byPdbccId = new HashMap>();
byKeyword = new HashMap>();
byComponent = new HashMap>();
byCategory = new EnumMap>(
ModificationCategory.class);
for (ModificationCategory cat:ModificationCategory.values()) {
byCategory.put(cat, new HashSet());
}
byOccurrenceType = new EnumMap>(
ModificationOccurrenceType.class);
for (ModificationOccurrenceType occ:ModificationOccurrenceType.values()) {
byOccurrenceType.put(occ, new HashSet());
}
registerCommonProteinModifications(inStream);
}
}
/**
* Register a new ProteinModification.
*/
public static void register(final ProteinModification modification) {
if (modification==null) throw new IllegalArgumentException("modification == null!");
lazyInit();
String id = modification.getId();
if (byId.containsKey(id)) {
throw new IllegalArgumentException(id+" has already been registered.");
}
registry.add(modification);
byId.put(id, modification);
ModificationCategory cat = modification.getCategory();
byCategory.get(cat).add(modification);
ModificationOccurrenceType occType = modification.getOccurrenceType();
byOccurrenceType.get(occType).add(modification);
ModificationCondition condition = modification.getCondition();
List comps = condition.getComponents();
for (Component comp:comps) {
Set mods = byComponent.get(comp);
if (mods==null) {
mods = new HashSet();
byComponent.put(comp, mods);
}
mods.add(modification);
}
String pdbccId = modification.getPdbccId();
if (pdbccId!=null) {
Set mods = byPdbccId.get(pdbccId);
if (mods==null) {
mods = new HashSet();
byPdbccId.put(pdbccId, mods);
}
mods.add(modification);
}
String residId = modification.getResidId();
if (residId!=null) {
Set mods = byResidId.get(residId);
if (mods==null) {
mods = new HashSet();
byResidId.put(residId, mods);
}
mods.add(modification);
}
String psimodId = modification.getPsimodId();
if (psimodId!=null) {
Set mods = byPsimodId.get(psimodId);
if (mods==null) {
mods = new HashSet();
byPsimodId.put(psimodId, mods);
}
mods.add(modification);
}
for (String keyword : modification.getKeywords()) {
Set mods = byKeyword.get(keyword);
if (mods==null) {
mods = new HashSet();
byKeyword.put(keyword, mods);
}
mods.add(modification);
}
}
/**
* Remove a modification from registry.
* @param mod
*/
public static void unregister(ProteinModification modification) {
if (modification==null) throw new IllegalArgumentException("modification == null!");
registry.remove(modification);
byId.remove(modification.getId());
Set mods;
mods = byResidId.get(modification.getResidId());
if (mods!=null) mods.remove(modification);
mods = byPsimodId.get(modification.getPsimodId());
if (mods!=null) mods.remove(modification);
mods = byPdbccId.get(modification.getPdbccId());
if (mods!=null) mods.remove(modification);
for (String keyword : modification.getKeywords()) {
mods = byKeyword.get(keyword);
if (mods!=null) mods.remove(modification);
}
ModificationCondition condition = modification.getCondition();
List comps = condition.getComponents();
for (Component comp : comps) {
mods = byComponent.get(comp);
if (mods!=null) mods.remove(modification);
}
byCategory.get(modification.getCategory()).remove(modification);
byOccurrenceType.get(modification.getOccurrenceType()).remove(modification);
}
/**
*
* @param id modification ID.
* @return ProteinModification that has the corresponding ID.
*/
public static ProteinModification getById(final String id) {
lazyInit();
return byId.get(id);
}
/**
*
* @param residId RESID ID.
* @return a set of ProteinModifications that have the RESID ID.
*/
public static Set getByResidId(final String residId) {
lazyInit();
return byResidId.get(residId);
}
/**
*
* @param psimodId PSI-MOD ID.
* @return a set of ProteinModifications that have the PSI-MOD ID.
*/
public static Set getByPsimodId(final String psimodId) {
lazyInit();
return byPsimodId.get(psimodId);
}
/**
*
* @param pdbccId Protein Data Bank Chemical Component ID.
* @return a set of ProteinModifications that have the PDBCC ID.
*/
public static Set getByPdbccId(final String pdbccId) {
lazyInit();
return byPdbccId.get(pdbccId);
}
/**
*
* @param keyword a keyword.
* @return a set of ProteinModifications that have the keyword.
*/
public static Set getByKeyword(final String keyword) {
lazyInit();
return byKeyword.get(keyword);
}
/**
* Get ProteinModifications that involves one or more components.
* @param comp1 a {@link Component}.
* @param comps other {@link Component}s.
* @return a set of ProteinModifications that involves all the components.
*/
public static Set getByComponent(final Component comp1,
final Component... comps) {
lazyInit();
Set mods = byComponent.get(comp1);
if (mods==null) {
return Collections.emptySet();
}
if (comps.length==0) {
return Collections.unmodifiableSet(mods);
} else {
Set ret = new HashSet(mods);
for (Component comp:comps) {
mods = byComponent.get(comp);
if (mods==null) {
return Collections.emptySet();
} else {
ret.retainAll(mods);
}
}
return ret;
}
}
/**
*
* @return set of all registered ProteinModifications.
*/
public static Set allModifications() {
lazyInit();
return Collections.unmodifiableSet(registry);
}
/**
*
* @param cat {@link ModificationCategory}.
* @return set of registered ProteinModifications in a particular category.
*/
public static Set getByCategory(final ModificationCategory cat) {
lazyInit();
Set ret = byCategory.get(cat);
return Collections.unmodifiableSet(ret);
}
/**
*
* @param occ {@link ModificationOccurrenceType}.
* @return set of registered ProteinModifications of a particular occurrence type.
*/
public static Set getByOccurrenceType(final ModificationOccurrenceType occ) {
lazyInit();
Set ret = byOccurrenceType.get(occ);
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of IDs of all registered ProteinModifications.
*/
public static Set allIds() {
lazyInit();
Set ret = byId.keySet();
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of PDBCC IDs of all registered ProteinModifications.
*/
public static Set allPdbccIds() {
lazyInit();
Set ret = byPdbccId.keySet();
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of RESID IDs of all registered ProteinModifications.
*/
public static Set allResidIds() {
lazyInit();
Set ret = byResidId.keySet();
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of PSI-MOD IDs of all registered ProteinModifications.
*/
public static Set allPsimodIds() {
lazyInit();
Set ret = byPsimodId.keySet();
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of components involved in all registered ProteinModifications.
*/
public static Set allComponents() {
lazyInit();
Set ret = byComponent.keySet();
return Collections.unmodifiableSet(ret);
}
/**
*
* @return set of keywords of all registered ProteinModifications.
*/
public static Set allKeywords() {
lazyInit();
Set ret = byKeyword.keySet();
return Collections.unmodifiableSet(ret);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy