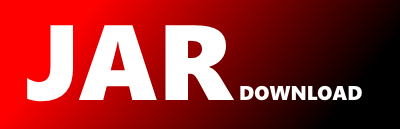
org.biojava.nbio.ontology.Triple Maven / Gradle / Ivy
/*
* BioJava development code
*
* This code may be freely distributed and modified under the
* terms of the GNU Lesser General Public Licence. This should
* be distributed with the code. If you do not have a copy,
* see:
*
* http://www.gnu.org/copyleft/lesser.html
*
* Copyright for this code is held jointly by the individual
* authors. These should be listed in @author doc comments.
*
* For more information on the BioJava project and its aims,
* or to join the biojava-l mailing list, visit the home page
* at:
*
* http://www.biojava.org/
*
*/
package org.biojava.nbio.ontology;
import org.biojava.nbio.ontology.utils.Annotation;
import java.util.Arrays;
import java.util.Set;
import java.util.TreeSet;
/**
* A triple in an ontology. This is two terms and a relationship between
* them, similar to RDF and other similar logic systems.
*
*
* For documentation purposes, a Triple may provide a name. However, a Triple
* may also be named as "(subject, object, predicate)" if no specific name is
* provided.
*
*
* @author Thomas Down
* @author Matthew Pocock
* @since 1.4
* @see org.biojavax.ontology.ComparableTriple
*/
public interface Triple
extends Term {
/**
* Return the subject term of this triple
* @return the subject term
*/
public Term getSubject();
/**
* Return the object term of this triple.
* @return the object term
*/
public Term getObject();
/**
* Return a Term which defines the type of relationship between the subject and object terms.
* @return the predicate
*/
public Term getPredicate();
/**
* The hashcode for a Triple.
*
* This must be implemented as:
*
* return getSubject().hashCode() +
* 31 * getObject().hashCode() +
* 31 * 31 * getPredicate().hashCode();
*
* If you do not implement hashcode in this way then you have no guarantee
* that your Triple objects will be found in an ontology and that they will
* not be duplicated.
*
*/
@Override
public int hashCode();
/**
* Check to see if an object is an equivalent Triple.
*
*
* Two triples are equivalent if they have the same subject, object and
* predicate fields.
*
* if (! (o instanceof Triple)) {
* return false;
* }
* Triple to = (Triple) o;
* return to.getSubject() == getSubject() &&
* to.getObject() == getObject() &&
* to.getPredicate() == getPredicate();
*
* If you do not implement equals in this way then you have no guarantee
* that your Triple objects will be found in an ontology and that they will
* not be duplicated.
*
*/
@Override
public boolean equals(Object obj);
/**
* Basic in-memory implementation of a Triple in an ontology
*
* This can be used to implement Ontology.createTriple
* @see org.biojavax.ontology.SimpleComparableTriple
*/
public static final class Impl
implements Triple, java.io.Serializable {
/**
*
*/
private static final long serialVersionUID = 3807331980372839221L;
private final Term subject;
private final Term object;
private final Term predicate;
private /*final*/ String name;
private /*final*/ String description;
private Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy